
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 #include "mbed.h" 00029 #include "communication.h" 00030 #include "port.h" 00031 00032 int main() 00033 { 00034 init_PORT(); 00035 00036 while(1) 00037 { 00038 buzzer.OFF(); 00039 com.parseMessage(); 00040 buzzer.time_out_init(); 00041 if(com.getInfoOK(0) == 1) //car 00042 { 00043 printf("main(). Car action starting...\r\n"); 00044 com.forwardMessage(); 00045 com.ACK(&lifter, &camera_platform); 00046 com.resetInfoOK(0); 00047 com.resetInfoOK(1); 00048 printf("main(). Car action ended...\r\n"); 00049 } 00050 else if(com.getInfoOK(0) == 2) //lifter 00051 { 00052 printf("main(). Lifter action starting...\r\n"); 00053 lifter.lifterMove(com.getMoveDis(), com.getMoveDir(), com.getRotateDis(), com.getRotateDir()); 00054 com.ACK(&lifter, &camera_platform); 00055 com.resetInfoOK(0); 00056 com.resetInfoOK(1); 00057 printf("main(). Lifter action ended...\r\n"); 00058 } 00059 else if(com.getInfoOK(0) == 3) //camera_platform 00060 { 00061 printf("main(). camera_platform action starting...\r\n"); 00062 camera_platform.cameraPlatformMove(com.getMoveDis(), com.getMoveDir(), com.getRotateDis(), com.getRotateDir()); 00063 com.ACK(&lifter, &camera_platform); 00064 com.resetInfoOK(0); 00065 com.resetInfoOK(1); 00066 printf("main(). Camera_platform action ended...\r\n"); 00067 } 00068 else if(com.getInfoOK(0) == 4) //compass 00069 { 00070 printf("main(). Compass action starting...\r\n"); 00071 com.ACK(&lifter, &camera_platform); 00072 com.resetInfoOK(0); 00073 com.resetInfoOK(1); 00074 printf("main(). Compass action ended...\r\n"); 00075 } 00076 else if(com.getInfoOK(0) == 5) //buzzer 00077 { 00078 printf("main(). Buzzer action starting...\r\n"); 00079 buzzer.ON(); 00080 wait(0.1); 00081 buzzer.OFF(); 00082 wait(0.1); 00083 buzzer.ON(); 00084 wait(0.2); 00085 buzzer.OFF(); 00086 com.ACK(&lifter, &camera_platform); 00087 com.resetInfoOK(0); 00088 com.resetInfoOK(1); 00089 printf("main(). Buzzer action ended...\r\n"); 00090 } 00091 00092 buzzer.cleanFlag(); 00093 } 00094 } 00095 00096 00097 00098
Generated on Thu Jul 14 2022 22:42:47 by
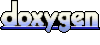