
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
lifter.h
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 #include "mbed.h" 00029 #include "define.h" 00030 00031 #ifndef _LIFTER_H 00032 #define _LIFTER_H 00033 00034 /* 00035 DigitalOut lifter_enable(p23); 00036 PwmOut lifter_pwmUp(p21); 00037 PwmOut lifter_pwmDown(p22); 00038 DigitalIn lifter_encoder_A(p26); 00039 DigitalIn lifter_encoder_B(p25); 00040 */ 00041 00042 //100 pulse => 8 mm 00043 #define RPMM 13 //pulseCount per mm 00044 00045 class Lifter 00046 { 00047 public: 00048 Lifter(MyDigitalOut* _enable, MyPwmOut* _pwmUp, MyPwmOut* _pwmDown, MyInterruptIn* encoder_A, MyDigitalIn* encoder_B); 00049 ~Lifter(); 00050 void lifterUp(uint16_t mm); 00051 void lifterDown(uint16_t mm); 00052 void lifterMove(uint16_t move_dis, uint8_t move_dir, uint16_t rotate_dis, uint8_t rotate_dir); 00053 uint8_t getDir(); 00054 uint8_t isStopped(); 00055 00056 uint32_t pulseCount; 00057 uint32_t targetPulseCount; 00058 00059 void setLifterStop(); 00060 void setLifterUp(); 00061 void setLifterDown(); 00062 00063 private: 00064 MyDigitalOut* _enable; 00065 MyPwmOut* _pwmUp; 00066 MyPwmOut* _pwmDown; 00067 MyInterruptIn* _encoder_A; //6 pulse per round 00068 MyDigitalIn* _encoder_B; //5v is up, 0v is down. looks like no use 00069 uint8_t _dir; 00070 uint8_t _stop; //0 means moving, 1 means stopped 00071 }; 00072 00073 #endif
Generated on Thu Jul 14 2022 22:42:47 by
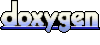