
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
lifter.cpp
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 #include "lifter.h" 00029 00030 Lifter::Lifter(MyDigitalOut* _enable, MyPwmOut* _pwmUp, MyPwmOut* _pwmDown, MyInterruptIn* _encoder_A, MyDigitalIn* _encoder_B) 00031 { 00032 pulseCount = 0; 00033 targetPulseCount = 0; 00034 _dir = 0; 00035 _stop = 0; 00036 00037 this->_enable = _enable; 00038 this->_pwmUp = _pwmUp; 00039 this->_pwmDown = _pwmDown; 00040 this->_encoder_A = _encoder_A; 00041 this->_encoder_B = _encoder_B; 00042 00043 setLifterStop(); 00044 } 00045 00046 Lifter::~Lifter() 00047 { 00048 delete _enable; 00049 delete _pwmUp; 00050 delete _pwmDown; 00051 delete _encoder_A; //6 pulse per round 00052 delete _encoder_B; //5v is up, 0v is down. looks like no use 00053 } 00054 00055 void Lifter::lifterUp(uint16_t mm) 00056 { 00057 targetPulseCount = mm * RPMM; 00058 //targetPulseCount = mm; 00059 setLifterUp(); 00060 } 00061 00062 void Lifter::lifterDown(uint16_t mm) 00063 { 00064 targetPulseCount = mm * RPMM; 00065 //targetPulseCount = mm; 00066 setLifterDown(); 00067 } 00068 00069 uint8_t Lifter::getDir() 00070 { 00071 /* 00072 if(*_encoder_B == 1) //up 00073 { 00074 return true; 00075 } 00076 else //down 00077 { 00078 return false; 00079 } 00080 */ 00081 return _dir; 00082 } 00083 uint8_t Lifter::isStopped() 00084 { 00085 return _stop; 00086 } 00087 00088 void Lifter::lifterMove(uint16_t move_dis, uint8_t move_dir, uint16_t rotate_dis, uint8_t rotate_dir) 00089 { 00090 pulseCount = targetPulseCount = 0; 00091 _stop = 0; 00092 00093 if(_dir = move_dir == 0x00) //up 00094 { 00095 lifterUp(move_dis); 00096 } 00097 else if(_dir = move_dir == 0x02) //down 00098 { 00099 lifterDown(move_dis); 00100 } 00101 } 00102 00103 void Lifter::setLifterStop() 00104 { 00105 *_enable = 0; 00106 *_pwmUp = 1.0f; 00107 *_pwmDown = 1.0f; 00108 _stop = 1; 00109 } 00110 void Lifter::setLifterUp() 00111 { 00112 *_enable = 0; 00113 *_pwmUp = 1.0f; 00114 *_pwmDown = 0.0f; 00115 } 00116 void Lifter::setLifterDown() 00117 { 00118 *_enable = 0; 00119 *_pwmUp = 0.0f; 00120 *_pwmDown = 1.0f; 00121 }
Generated on Thu Jul 14 2022 22:42:47 by
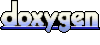