
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
camera_platform.h
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 #include "mbed.h" 00029 #include "define.h" 00030 00031 #ifndef _CAMERA_PLATFORM_H 00032 #define _CAMERA_PLATFORM_H 00033 00034 /* 00035 PwmOut camera_platform_pwmRoll(p24); 00036 PwmOut camera_platform_pwmPitch(p26); 00037 PwmOut camera_platform_pwmYaw(p25); 00038 */ 00039 00040 #define ROLL_MID 1500 00041 #define ROLL_LOW 1000 00042 #define ROLL_HIGH 2000 00043 #define ROLL_ANGLE_MAX 90 00044 #define ROLL_ANGLE_MIN -90 00045 #define ROLL_USPD (2000-1500)/180 00046 00047 #define PITCH_MID 1500 00048 #define PITCH_LOW 1300 00049 #define PITCH_HIGH 2200 00050 #define PITCH_ANGLE_MAX 90 00051 #define PITCH_ANGLE_MIN -25 00052 #define PITCH_USPD (2200-1300)/115 00053 00054 #define YAW_MID 1400 00055 #define YAW_LOW 600 00056 #define YAW_HIGH 2200 00057 #define YAW_ANGLE_MAX 90 00058 #define YAW_ANGLE_MIN -90 00059 #define YAW_USPD (2200-600)/180 //us per degree => 500/90 00060 00061 #define ROLL 0 00062 #define PITCH 1 00063 #define YAW 2 00064 00065 class Camera_platform 00066 { 00067 public: 00068 Camera_platform(MyPwmOut* _pwmRoll, MyPwmOut* _pwmPitch, MyPwmOut* _pwmYaw); 00069 ~Camera_platform(); 00070 00071 void cameraPlatformMove(uint16_t move_dis, uint8_t move_dir, uint16_t rotate_dis, uint8_t rotate_dir); 00072 00073 void setRollLeft(float _degree); 00074 void setRollRight(float _degree); 00075 void setPitchUp(float _degree); 00076 void setPitchDown(float _degree); 00077 void setYawClock(float _degree); 00078 void setYawCClock(float _degree); 00079 00080 void resetCameraPlatform(); 00081 00082 uint8_t dir; 00083 uint8_t angle; 00084 00085 private: 00086 MyPwmOut* _pwmRoll; 00087 MyPwmOut* _pwmPitch; 00088 MyPwmOut* _pwmYaw; 00089 00090 float _roll_angle; //record the current roll angle. need to divide 100 to convert to degree 00091 float _pitch_angle; //record the current pitch angle. need to divide 100 to convert to degree 00092 float _yaw_angle; //record the current yaw angle. need to divide 100 to convert to degree 00093 00094 void setPWM(uint16_t _pwm_value_us, uint8_t _pwm_channel); //0 is roll, 1 is pitch, 2 is yaw 00095 uint16_t computePwmValue(float _degree, uint8_t _dir, uint8_t _pwm_channel); //0 is left/up/clock, 1 is right/down/cclock 00096 }; 00097 00098 #endif
Generated on Thu Jul 14 2022 22:42:47 by
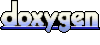