Optimised fork of bikeNomad's WS2811 LED control library. Supports KL25Z and KL46Z
Fork of Multi_WS2811 by
LedStrip.h
00001 // Parent class for all addressable LED strips. 00002 // Partially based on work by and (c) 2011 Jelmer Tiete 00003 // whose library is ported from the Arduino implementation of Adafruit Industries 00004 // found at: http://github.com/adafruit/LPD8806 00005 // and their strips: http://www.adafruit.com/products/306 00006 // Released under the MIT License: http://mbed.org/license/mit 00007 00008 // This is a pure virtual parent class for all LED strips, so that different types 00009 // of strip may be used in a single array or container. 00010 00011 #include "mbed.h" 00012 00013 #ifndef LEDSTRIP_H 00014 #define LEDSTRIP_H 00015 00016 /** Generic LED Strip 00017 Pure virtual parent class for all types of LED strip 00018 */ 00019 class LedStrip 00020 { 00021 public: 00022 /** Create an LED strip 00023 @param pixelCount Number of RGB LEDs on the strip 00024 */ 00025 LedStrip(uint16_t pixelCount); 00026 ~LedStrip(); 00027 00028 //! Initialise the LED strip 00029 virtual void begin(void)=0; 00030 //! Apply the new LED strip values 00031 virtual void show(void)=0; 00032 //! Blank the LED strip 00033 virtual void blank(void)=0; 00034 00035 /** Pack RGB Color data 00036 @param red Amount of Red 00037 @param green Amount of Green 00038 @param blue Amount of Blue 00039 @returns Packed RGB color data for one pixel 00040 */ 00041 static uint32_t Color(uint8_t red, uint8_t green, uint8_t blue); 00042 00043 //! Number of RGB pixels 00044 uint16_t numPixels() { 00045 return numLEDs; 00046 } 00047 //! Number of bytes used for pixel colour data 00048 uint16_t numPixelBytes() { 00049 return numLEDs * 3; 00050 } 00051 /** Total brightness of all diodes\n 00052 * Use to check power budget 00053 @returns Sum total of all diodes (red + green + blue) 00054 */ 00055 uint32_t total_luminance(); 00056 00057 /** Set Blue level of pixel 00058 @param pixNum Pixel Number 00059 @param blue Amount of Blue 00060 */ 00061 void setPixelB(uint16_t pixNum, uint8_t blue); 00062 /** Set Green level of pixel 00063 @param pixNum Pixel Number 00064 @param green Amount of Green 00065 */ 00066 void setPixelG(uint16_t pixNum, uint8_t green); 00067 /** Set Red level of pixel 00068 @param pixNum Pixel Number 00069 @param red Amount of Red 00070 */ 00071 void setPixelR(uint16_t pixNum, uint8_t red); 00072 00073 /** Set color of pixel 00074 @param pixNum Pixel Number 00075 @param color Packed RGB color data 00076 */ 00077 void setPixelColor(uint16_t pixNum, uint32_t color); 00078 /** Set color of pixel 00079 @param pixNum Pixel Number 00080 @param red Amount of Red 00081 @param green Amount of Green 00082 @param blue Amount of Blue 00083 */ 00084 void setPixelColor(uint16_t pixNum, uint8_t red, uint8_t green, uint8_t blue); 00085 /** Set color of all pixels 00086 @param *buffer Packed pixel data 00087 @param count Number of pixels 00088 */ 00089 void setPackedPixels(uint8_t * buffer, uint32_t count); 00090 00091 protected: 00092 uint8_t *pixels; // Holds LED color values 00093 uint16_t numLEDs; // Number of RGB LEDs in strand 00094 }; 00095 #endif
Generated on Mon Jul 18 2022 21:17:31 by
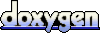