
Nanopb (lightweight version of googles protobuf) test. It is not working as it should yet.
Embed:
(wiki syntax)
Show/hide line numbers
pb_decode.h
00001 /* pb_decode.h: Functions to decode protocol buffers. Depends on pb_decode.c. 00002 * The main function is pb_decode. You also need an input stream, and the 00003 * field descriptions created by nanopb_generator.py. 00004 */ 00005 00006 #ifndef _PB_DECODE_H_ 00007 #define _PB_DECODE_H_ 00008 00009 #include "pb.h" 00010 00011 #ifdef __cplusplus 00012 extern "C" { 00013 #endif 00014 00015 /* Structure for defining custom input streams. You will need to provide 00016 * a callback function to read the bytes from your storage, which can be 00017 * for example a file or a network socket. 00018 * 00019 * The callback must conform to these rules: 00020 * 00021 * 1) Return false on IO errors. This will cause decoding to abort. 00022 * 2) You can use state to store your own data (e.g. buffer pointer), 00023 * and rely on pb_read to verify that no-body reads past bytes_left. 00024 * 3) Your callback may be used with substreams, in which case bytes_left 00025 * is different than from the main stream. Don't use bytes_left to compute 00026 * any pointers. 00027 */ 00028 struct _pb_istream_t 00029 { 00030 #ifdef PB_BUFFER_ONLY 00031 /* Callback pointer is not used in buffer-only configuration. 00032 * Having an int pointer here allows binary compatibility but 00033 * gives an error if someone tries to assign callback function. 00034 */ 00035 int *callback; 00036 #else 00037 bool (*callback)(pb_istream_t *stream, uint8_t *buf, size_t count); 00038 #endif 00039 00040 void *state; /* Free field for use by callback implementation */ 00041 size_t bytes_left; 00042 00043 #ifndef PB_NO_ERRMSG 00044 const char *errmsg; 00045 #endif 00046 }; 00047 00048 /*************************** 00049 * Main decoding functions * 00050 ***************************/ 00051 00052 /* Decode a single protocol buffers message from input stream into a C structure. 00053 * Returns true on success, false on any failure. 00054 * The actual struct pointed to by dest must match the description in fields. 00055 * Callback fields of the destination structure must be initialized by caller. 00056 * All other fields will be initialized by this function. 00057 * 00058 * Example usage: 00059 * MyMessage msg = {}; 00060 * uint8_t buffer[64]; 00061 * pb_istream_t stream; 00062 * 00063 * // ... read some data into buffer ... 00064 * 00065 * stream = pb_istream_from_buffer(buffer, count); 00066 * pb_decode(&stream, MyMessage_fields, &msg); 00067 */ 00068 bool pb_decode(pb_istream_t *stream, const pb_field_t fields[], void *dest_struct); 00069 00070 /* Same as pb_decode, except does not initialize the destination structure 00071 * to default values. This is slightly faster if you need no default values 00072 * and just do memset(struct, 0, sizeof(struct)) yourself. 00073 * 00074 * This can also be used for 'merging' two messages, i.e. update only the 00075 * fields that exist in the new message. 00076 */ 00077 bool pb_decode_noinit(pb_istream_t *stream, const pb_field_t fields[], void *dest_struct); 00078 00079 /* Same as pb_decode, except expects the stream to start with the message size 00080 * encoded as varint. Corresponds to parseDelimitedFrom() in Google's 00081 * protobuf API. 00082 */ 00083 bool pb_decode_delimited(pb_istream_t *stream, const pb_field_t fields[], void *dest_struct); 00084 00085 00086 /************************************** 00087 * Functions for manipulating streams * 00088 **************************************/ 00089 00090 /* Create an input stream for reading from a memory buffer. 00091 * 00092 * Alternatively, you can use a custom stream that reads directly from e.g. 00093 * a file or a network socket. 00094 */ 00095 pb_istream_t pb_istream_from_buffer(uint8_t *buf, size_t bufsize); 00096 00097 /* Function to read from a pb_istream_t. You can use this if you need to 00098 * read some custom header data, or to read data in field callbacks. 00099 */ 00100 bool pb_read(pb_istream_t *stream, uint8_t *buf, size_t count); 00101 00102 00103 /************************************************ 00104 * Helper functions for writing field callbacks * 00105 ************************************************/ 00106 00107 /* Decode the tag for the next field in the stream. Gives the wire type and 00108 * field tag. At end of the message, returns false and sets eof to true. */ 00109 bool pb_decode_tag(pb_istream_t *stream, pb_wire_type_t *wire_type, uint32_t *tag, bool *eof); 00110 00111 /* Skip the field payload data, given the wire type. */ 00112 bool pb_skip_field(pb_istream_t *stream, pb_wire_type_t wire_type); 00113 00114 /* Decode an integer in the varint format. This works for bool, enum, int32, 00115 * int64, uint32 and uint64 field types. */ 00116 bool pb_decode_varint(pb_istream_t *stream, uint64_t *dest); 00117 00118 /* Decode an integer in the zig-zagged svarint format. This works for sint32 00119 * and sint64. */ 00120 bool pb_decode_svarint(pb_istream_t *stream, int64_t *dest); 00121 00122 /* Decode a fixed32, sfixed32 or float value. You need to pass a pointer to 00123 * a 4-byte wide C variable. */ 00124 bool pb_decode_fixed32(pb_istream_t *stream, void *dest); 00125 00126 /* Decode a fixed64, sfixed64 or double value. You need to pass a pointer to 00127 * a 8-byte wide C variable. */ 00128 bool pb_decode_fixed64(pb_istream_t *stream, void *dest); 00129 00130 /* Make a limited-length substream for reading a PB_WT_STRING field. */ 00131 bool pb_make_string_substream(pb_istream_t *stream, pb_istream_t *substream); 00132 void pb_close_string_substream(pb_istream_t *stream, pb_istream_t *substream); 00133 00134 #ifdef __cplusplus 00135 } /* extern "C" */ 00136 #endif 00137 00138 #endif 00139
Generated on Thu Jul 14 2022 19:55:28 by
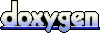