
Load different fonts into uLCD.
Dependencies: 4DGL-uLCD-SE PinDetect mbed
Fork of uLCD144G2_demo by
main.cpp
00001 // uLCD-144-G2 different fonts 00002 // 00003 #include "mbed.h" 00004 #include "uLCD_4DGL.h" 00005 #include "PinDetect.h" 00006 00007 uLCD_4DGL uLCD(p9,p10,p11); // serial tx, serial rx, reset pin; 00008 PinDetect pb1(p15); 00009 PinDetect pb2(p16); 00010 PinDetect pb3(p17); 00011 00012 int volatile sizeCount=1; //font size count, set to 1 initially 00013 int volatile fontCount=0; //font count 00014 int volatile isInterrupted=1; //indicate an interrupt 00015 00016 //pb1 to increase font size 00017 void pb1_hit_callback (void) { 00018 if (sizeCount < 4) {sizeCount++;} 00019 uLCD.cls(); 00020 isInterrupted=1; 00021 } 00022 00023 //pb2 to decrease font size 00024 void pb2_hit_callback (void) { 00025 if (sizeCount > 1) {sizeCount--;} 00026 uLCD.cls(); 00027 isInterrupted=1; 00028 } 00029 00030 //pb3 changes font. Loops through default, comic sans, tahoma, and courier new 00031 void pb3_hit_callback (void) { 00032 if (fontCount < 3) {fontCount++;} 00033 else {fontCount = 0;} 00034 uLCD.cls(); 00035 isInterrupted=1; 00036 } 00037 00038 int main() 00039 { 00040 pb1.mode(PullUp); 00041 pb2.mode(PullUp); 00042 pb3.mode(PullUp); 00043 wait(.01); 00044 // Setup Interrupt callback functions for a pb hit 00045 pb1.attach_deasserted(&pb1_hit_callback); 00046 pb2.attach_deasserted(&pb2_hit_callback); 00047 pb3.attach_deasserted(&pb3_hit_callback); 00048 // Start sampling pb inputs using interrupts 00049 pb1.setSampleFrequency(); 00050 pb2.setSampleFrequency(); 00051 pb3.setSampleFrequency(); 00052 uLCD.media_init(); // initialize uSD card 00053 uLCD.printf("\n Hello\n Font\n World"); 00054 while (1) { 00055 //change font settings if a button is pushed 00056 if(isInterrupted == 1) { 00057 uLCD.cls(); 00058 isInterrupted = 0; 00059 //comic sans 00060 if(fontCount == 1) { 00061 uLCD.set_sector_address(0,0); 00062 uLCD.set_font(MEDIAFONT); 00063 } 00064 //tahoma 00065 else if(fontCount == 2) { 00066 uLCD.set_sector_address(0,0xE); 00067 uLCD.set_font(MEDIAFONT); 00068 } 00069 //courier new 00070 else if(fontCount == 3) { 00071 uLCD.set_sector_address(0,0x1C); 00072 uLCD.set_font(MEDIAFONT); 00073 } 00074 //default font 00075 else { 00076 uLCD.set_sector_address(0,0); 00077 uLCD.set_font(FONT_7X8); 00078 } 00079 //increase or decrease font 00080 uLCD.text_width(sizeCount); //1X size text 00081 uLCD.text_height(sizeCount); 00082 uLCD.printf("\n Hello\n Font\n World"); 00083 } 00084 00085 } 00086 }
Generated on Mon Jul 25 2022 22:14:11 by
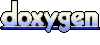