Library to read 433MHz codes with decoder HT6P20
Dependents: Gateway_Cotosys Gateway_Cotosys_os5
RFDecoder.cpp
00001 /* 00002 RFDecoder - Ported from the Lucas Maziero Arduino libary to decode RF controls based on chip HT6P20 00003 Copyright (c) 2011 Miguel Maldonado. All right reserved. 00004 00005 Project home: https://github.com/lucasmaziero/RF_HT6P20 00006 00007 This library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Lesser General Public 00009 License as published by the Free Software Foundation; either 00010 version 2.1 of the License, or (at your option) any later version. 00011 00012 This library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Lesser General Public License for more details. 00016 */ 00017 00018 #include "RFDecoder.h" 00019 #include "mbed.h" 00020 #include <Pulse.h> 00021 00022 00023 /** Class constructor. 00024 * The constructor assigns the specified pinout, attatches 00025 * an Interrupt to the receive pin. 00026 * @param tx Transmitter pin of the RF module. 00027 * @param rx Receiver pin of the RF module. 00028 */ 00029 00030 RFDecoder::RFDecoder(PinName tx, PinName rx) : _tx(DigitalOut(tx)), _rx(PulseInOut(rx)){ 00031 RFDecoder::addressFull = NULL; 00032 00033 } 00034 00035 unsigned long RFDecoder::getCode(){ 00036 return(addressFull); 00037 } 00038 00039 bool RFDecoder::available(){ 00040 static bool startbit=0; //checks if start bit was identified 00041 static int counter; //received bits counter: 20 of Address + 4 of Data + 4 of EndCode (Anti-Code) 00042 static unsigned long buffer; //buffer for received data storage 00043 00044 int dur0, dur1; // pulses durations (auxiliary) 00045 00046 if (!startbit) { // Check the PILOT CODE until START BIT; 00047 dur0 = _rx.read_low_us(); 00048 00049 //If time at "0" is between 9200 us (23 cycles of 400us) and 13800 us (23 cycles of 600 us). 00050 if((dur0 > 9200) && (dur0 < 13800) && !startbit){ 00051 //calculate wave length - lambda 00052 lambda_RX = (dur0 / 23); 00053 00054 //Reset variables 00055 dur0 = 0; 00056 buffer = 0; 00057 counter = 0; 00058 00059 startbit = true; 00060 } 00061 } 00062 00063 //If Start Bit is OK, then starts measure os how long the signal is level "1" and check is value is into acceptable range. 00064 if (startbit && counter < 28){ 00065 ++counter; 00066 00067 dur1 = _rx.read_high_us(); 00068 00069 if((dur1 > 0.5 * lambda_RX) && (dur1 < (1.5 * lambda_RX))){ //If pulse width at "1" is between "0.5 and 1.5 lambda", means that pulse is only one lambda, so the data is "1". 00070 buffer = (buffer << 1) + 1; // add "1" on data buffer 00071 } 00072 else if((dur1 > 1.5 * lambda_RX) && (dur1 < (2.5 * lambda_RX))){ //If pulse width at "1" is between "1.5 and 2.5 lambda", means that pulse is two lambdas, so the data is "0". 00073 buffer = (buffer << 1); // add "0" on data buffer 00074 } 00075 else{ 00076 startbit = false; //Reset the loop 00077 } 00078 } 00079 00080 //Check if all 28 bits were received (20 of Address + 4 of Data + 4 of Anti-Code) 00081 if (counter==28) 00082 { 00083 00084 // Check if Anti-Code is OK (last 4 bits of buffer equal "0101") 00085 if ((bitRead(buffer, 0) == 1) && (bitRead(buffer, 1) == 0) && (bitRead(buffer, 2) == 1) && (bitRead(buffer, 3) == 0)){ 00086 counter = 0; 00087 startbit = false; 00088 00089 //Get ADDRESS COMPLETO from Buffer 00090 addressFull = buffer; 00091 00092 //If a valid data is received, return OK 00093 return true; 00094 } 00095 else 00096 { 00097 //Reset the loop 00098 startbit = false; 00099 } 00100 00101 } 00102 00103 //If none valid data is received, return NULL and FALSE values 00104 addressFull = 0; 00105 return false; 00106 } 00107 00108 bool RFDecoder::bitRead( int N, int pos) 00109 { // pos = 7 6 5 4 3 2 1 0 00110 int b = N >> pos ; // Shift bits 00111 b = b & 1 ; // get only the last bit 00112 return b ; 00113 } 00114
Generated on Wed Mar 1 2023 13:46:36 by
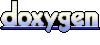