
a
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 ///////////////////////////////////////////////// BIBLIOTHEQUE /////////////////////////////////////////////////////////////////////////////////////// 00002 00003 #include "mbed.h" 00004 #include "main.h" 00005 #include "sx1272-hal.h" 00006 #include "debug.h" 00007 #include "sx1272.h" 00008 00009 00010 Serial pc(USBTX, USBRX); 00011 00012 #define PCBAUD 9600 00013 00014 //////////////////////////////////////////////// DEFINITION OUTIL DEBUG /////////////////////////////////////////////////////////////////////////// 00015 00016 /* Set this flag to '1' to display debug messages on the console */ 00017 00018 00019 #define DEBUG_MESSAGE 1 //permet d'afficher des messages sur le terminal 00020 00021 00022 ////////////////////////////////////////////////////////////////////// DEFINITION MODULATION ///////////////////////////////////////////////////// 00023 00024 /* Set this flag to '1' to use the LoRa modulation or to '0' to use FSK modulation */ 00025 00026 00027 #define USE_MODEM_LORA 1 //On utilise LoRa 00028 00029 #define RF_FREQUENCY 868000000 // Hz 00030 #define TX_OUTPUT_POWER 14 // dBm 00031 00032 #if USE_MODEM_LORA == 1 // Definition des parametres de LoRa 00033 00034 #define LORA_BANDWIDTH 2 // [0: 125 kHz, 00035 // 1: 250 kHz, 00036 // 2: 500 kHz, 00037 // 3: Reserved] 00038 #define LORA_SPREADING_FACTOR 7 // [SF7..SF12] // ??????????????? 00039 #define LORA_CODINGRATE 1 // [1: 4/5, // ??????????????? 00040 // 2: 4/6, // ??????????????? 00041 // 3: 4/7, 00042 // 4: 4/8] 00043 #define LORA_PREAMBLE_LENGTH 8 // Same for Tx and Rx // ??????????????? 00044 #define LORA_SYMBOL_TIMEOUT 5 // Symbols // ??????????????? 00045 #define LORA_FIX_LENGTH_PAYLOAD_ON false // ??????????????? 00046 #define LORA_FHSS_ENABLED false // ??????????????? 00047 #define LORA_NB_SYMB_HOP 4 // ??????????????? 00048 #define LORA_IQ_INVERSION_ON false // ??????????????? 00049 #define LORA_CRC_ENABLED true // ??????????????? 00050 00051 00052 #else 00053 #error "Please define a modem in the compiler options." //ERREUR si ni LoRa ni FSK n'a ete active 00054 #endif 00055 00056 #define RX_TIMEOUT_VALUE 10000 // in ms 00057 #define BUFFER_SIZE 32 // Define the payload size here 00058 00059 00060 00061 00062 00063 //////////////////////////////////////////////////// GLOBAL VARIABLES DECLARATIONS ///////////////////////////////////////////////////////////////////// 00064 00065 typedef enum 00066 { 00067 LOWPOWER = 0, 00068 IDLE, 00069 00070 RX, 00071 RX_TIMEOUT, 00072 RX_ERROR, 00073 00074 TX, 00075 TX_TIMEOUT, 00076 00077 CAD, 00078 CAD_DONE 00079 00080 }AppStates_t; 00081 00082 volatile AppStates_t State = LOWPOWER; 00083 00084 /*! 00085 * Radio events function pointer 00086 */ 00087 00088 00089 static RadioEvents_t RadioEvents; 00090 00091 00092 00093 /* 00094 * Global variables declarations 00095 */ 00096 00097 00098 SX1272MB2xAS Radio( NULL ); 00099 00100 uint16_t BufferSize = BUFFER_SIZE; //Taille de la memoire tampon 00101 uint8_t Buffer[BUFFER_SIZE]; 00102 00103 int16_t RssiValue = 0.0; //Rssi = Received Signal Strentgh Indication 00104 int8_t SnrValue = 0.0; 00105 00106 00107 ////////////////////////////////////////////////////////////////////// MAIN ///////////////////////////////////////////////////////// 00108 00109 00110 int main( void ) 00111 { 00112 pc.baud(PCBAUD); 00113 00114 debug( "\n\n\r SX1272 X Y Z Demo Application \n\n\r" ); 00115 00116 // Initialize Radio driver 00117 RadioEvents.TxDone = OnTxDone; 00118 RadioEvents.RxDone = OnRxDone; 00119 RadioEvents.RxError = OnRxError; 00120 RadioEvents.TxTimeout = OnTxTimeout; 00121 RadioEvents.RxTimeout = OnRxTimeout; 00122 Radio.Init( &RadioEvents ); 00123 00124 // verify the connection with the board 00125 while( Radio.Read( REG_VERSION ) == 0x00 ) 00126 { 00127 debug( "Radio could not be detected!\n\r", NULL ); 00128 wait( 1 ); 00129 } 00130 00131 debug_if( ( DEBUG_MESSAGE & ( Radio.DetectBoardType( ) == SX1272MB2XAS ) ), "\n\r > Board Type: SX1272MB2xAS < \n\r" ); 00132 00133 Radio.SetChannel( RF_FREQUENCY ); 00134 00135 #if USE_MODEM_LORA == 1 00136 00137 debug_if( LORA_FHSS_ENABLED, "\n\n\r > LORA FHSS Mode < \n\n\r" ); 00138 debug_if( !LORA_FHSS_ENABLED, "\n\n\r > LORA Mode < \n\n\r" ); 00139 00140 Radio.SetTxConfig( MODEM_LORA, TX_OUTPUT_POWER, 0, LORA_BANDWIDTH, 00141 LORA_SPREADING_FACTOR, LORA_CODINGRATE, 00142 LORA_PREAMBLE_LENGTH, LORA_FIX_LENGTH_PAYLOAD_ON, 00143 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00144 LORA_IQ_INVERSION_ON, 2000 ); 00145 00146 Radio.SetRxConfig( MODEM_LORA, LORA_BANDWIDTH, LORA_SPREADING_FACTOR, 00147 LORA_CODINGRATE, 0, LORA_PREAMBLE_LENGTH, 00148 LORA_SYMBOL_TIMEOUT, LORA_FIX_LENGTH_PAYLOAD_ON, 0, 00149 LORA_CRC_ENABLED, LORA_FHSS_ENABLED, LORA_NB_SYMB_HOP, 00150 LORA_IQ_INVERSION_ON, true ); 00151 00152 00153 #else 00154 00155 #error "Please define a modem in the compiler options." 00156 00157 #endif 00158 00159 Radio.Rx( 3500 ); 00160 State = RX; 00161 pc.printf("\n\r ++++++++++++++++++++++++++++++++ \n\r"); 00162 00163 while(1) 00164 { 00165 00166 if( BufferSize > 0 ) 00167 { 00168 if( strncmp( (const char *)Buffer, "B", 1)==0) 00169 { 00170 00171 pc.printf("%s,\n", Buffer); 00172 pc.printf("\n\r"); 00173 00174 00175 } 00176 00177 BufferSize = 0; 00178 Radio.Rx( 3500 ); 00179 } 00180 00181 00182 00183 00184 } 00185 } 00186 00187 00188 00189 00190 00191 00192 00193 00194 ///////////////////////////////////////// DECLARATION DES FONCTIONS///////////////////////////////////////////////////// 00195 00196 void OnTxDone( void ) 00197 { 00198 Radio.Sleep( ); 00199 State = TX; 00200 debug_if( DEBUG_MESSAGE, "> OnTxDone\n\r" ); 00201 } 00202 00203 void OnRxDone( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ) 00204 { 00205 Radio.Sleep( ); 00206 BufferSize = size; 00207 memcpy( Buffer, payload, BufferSize ); 00208 RssiValue = rssi; 00209 SnrValue = snr; 00210 State = RX; 00211 debug_if( DEBUG_MESSAGE, "> OnRxDone\n\r" ); 00212 } 00213 00214 void OnTxTimeout( void ) 00215 { 00216 Radio.Sleep( ); 00217 State = TX_TIMEOUT; 00218 debug_if( DEBUG_MESSAGE, "> OnTxTimeout\n\r" ); 00219 } 00220 00221 void OnRxTimeout( void ) 00222 { 00223 //Radio.Sleep( ); 00224 Buffer[BufferSize] = 0; 00225 State = RX_TIMEOUT; 00226 debug_if( DEBUG_MESSAGE, "> OnRxTimeout\n\r" ); 00227 } 00228 00229 void OnRxError( void ) 00230 { 00231 State = RX_ERROR; 00232 debug_if( DEBUG_MESSAGE, "> OnRxError\n\r" ); 00233 }
Generated on Fri Jul 22 2022 06:24:56 by
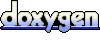