
Example for the RCSwitch Library. In receive mode, this will receive messages from the remote and decide which protocol has been used for transmission. In transmit mode, this will cycle through different formats for sending codewords.
main.cpp
00001 #include "mbed.h" 00002 #include "RCSwitch.h" 00003 00004 // This Example should only do one of either transmit or receive 00005 //#define TRANSMIT 00006 #define RECEIVE 00007 00008 Serial pc(USBTX, USBRX); // tx, rx 00009 RCSwitch mySwitch = RCSwitch( p11, p21 ); //tx, rx 00010 00011 int main() 00012 { 00013 pc.printf("Setup"); 00014 while(1) { 00015 #ifdef RECEIVE 00016 if (mySwitch.available()) { 00017 00018 int value = mySwitch.getReceivedValue(); 00019 00020 if (value == 0) { 00021 pc.printf("Unknown encoding"); 00022 } else { 00023 pc.printf("Received %d \n\r", mySwitch.getReceivedValue()); 00024 pc.printf(" bit %d \n\r", mySwitch.getReceivedBitlength()); 00025 pc.printf(" Protocol: %d \n\r", mySwitch.getReceivedProtocol()); 00026 } 00027 mySwitch.resetAvailable(); 00028 } 00029 #endif 00030 #ifdef TRANSMIT 00031 // Example: TypeA_WithDIPSwitches 00032 mySwitch.switchOn("11111", "00010"); 00033 wait(1); 00034 mySwitch.switchOn("11111", "00010"); 00035 wait(1); 00036 00037 // Same switch as above, but using decimal code 00038 mySwitch.send(5393, 24); 00039 wait(1); 00040 mySwitch.send(5396, 24); 00041 wait(1); 00042 00043 // Same switch as above, but using binary code 00044 mySwitch.send("000000000001010100010001"); 00045 wait(1); 00046 mySwitch.send("000000000001010100010100"); 00047 wait(1); 00048 00049 // Same switch as above, but tri-state code 00050 mySwitch.sendTriState("00000FFF0F0F"); 00051 wait(1); 00052 mySwitch.sendTriState("00000FFF0FF0"); 00053 wait(1); 00054 #endif 00055 } 00056 }
Generated on Tue Jul 12 2022 22:35:34 by
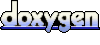