
jkcollision demo for the Gameduino
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "GD.h" 00003 #include "arduino.h" 00004 #include "shield.h" 00005 00006 GDClass GD(ARD_MOSI, ARD_MISO, ARD_SCK, ARD_D9, USBTX, USBRX) ; 00007 SPI spimain(ARD_MOSI, ARD_MISO, ARD_SCK); // mosi, miso, sclk 00008 #define PI 3.1415926535 00009 00010 void readn(byte *dst, unsigned int addr, int c) { 00011 GD.__start(addr); 00012 while (c--) 00013 *dst++ = spimain.write(0); 00014 GD.__end(); 00015 } 00016 00017 static byte coll[256]; 00018 static void load_coll() { 00019 while (GD.rd(VBLANK) == 0) // Wait until vblank 00020 ; 00021 while (GD.rd(VBLANK) == 1) // Wait until display 00022 ; 00023 while (GD.rd(VBLANK) == 0) // Wait until vblank 00024 ; 00025 readn(coll, COLLISION, sizeof(coll)); 00026 } 00027 00028 void setup() { 00029 int i; 00030 00031 GD.begin(); 00032 00033 GD.wr(JK_MODE, 1); 00034 GD.wr16(RAM_PAL, RGB(255,255,255)); 00035 00036 // Use the 4 palettes: 00037 // 0 pink, for J sprites 00038 // 1 green, for K sprites 00039 // 2 dark pink, J collisions 00040 // 3 dark green, K collisions 00041 for (i = 0; i < 256; i++) { 00042 GD.wr16(RAM_SPRPAL + (0 * 512) + (i << 1), RGB(255, 0, 255)); 00043 GD.wr16(RAM_SPRPAL + (1 * 512) + (i << 1), RGB(0, 255, 0)); 00044 GD.wr16(RAM_SPRPAL + (2 * 512) + (i << 1), RGB(100, 0, 100)); 00045 GD.wr16(RAM_SPRPAL + (3 * 512) + (i << 1), RGB(0, 100, 0)); 00046 } 00047 } 00048 00049 byte spr; 00050 static void polar(float th, int r, byte jk) { 00051 // add 2 to the palette if this sprite is colliding 00052 byte colliding = coll[spr] != 0xff; 00053 GD.sprite(spr, 200 + int(r * sin(th)), 142 + int(r * cos(th)), 0, jk + (colliding ? 2 : 0), 0, jk); 00054 spr++; 00055 } 00056 Timer timer; 00057 int main() { 00058 00059 timer.start(); 00060 setup(); 00061 byte i; 00062 float th; 00063 while (1) { 00064 spr = 0; 00065 // draw the J sprites (pink) 00066 for (i = 0; i < 5; i++) { 00067 th = (((timer.read_us()+2147)/500) / 3000.) + 2 * PI * i / 5; 00068 polar(th, 134, 0); 00069 } 00070 // draw the K sprites (green) 00071 randomSeed(4); 00072 for (i = 0; i < 17; i++) { 00073 th = (((timer.read_us()+2147)/500) / float((rand()%2000)+1000)) + 2 * PI * i / 17; 00074 polar(th, 134, 1); 00075 } 00076 load_coll(); 00077 } 00078 } 00079
Generated on Fri Jul 15 2022 21:24:06 by
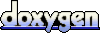