
Invaders game for the Gameduino
Embed:
(wiki syntax)
Show/hide line numbers
sound.cpp
00001 /*------------------------------------------------------------------ 00002 Game sounds 00003 ------------------------------------------------------------------*/ 00004 #include "game.h" 00005 #include "samples.h" 00006 00007 00008 /*------------------------------------------------------------------ 00009 Set these flags to start a sound playing 00010 ------------------------------------------------------------------*/ 00011 bool playerShootSound; 00012 bool alienDeathSound; 00013 bool playerDeathSound; 00014 bool alienBeatSound; 00015 bool saucerSound,stopSaucerSnd,saucerDieSound; 00016 static byte alienBeat; 00017 00018 #if SYNTHSOUNDS 00019 SoundPlayer soundPlayer; 00020 Sound shootSound; 00021 #endif 00022 00023 /*------------------------------------------------------------------ 00024 Sound functions 00025 ------------------------------------------------------------------*/ 00026 void resetGameSounds() 00027 { 00028 SoundController::reset(); 00029 playerShootSound = false; 00030 alienDeathSound = false; 00031 playerDeathSound = false; 00032 alienBeatSound = false; 00033 saucerSound = stopSaucerSnd = saucerDieSound = false; 00034 alienBeat = 0; 00035 } 00036 00037 void updateGameSounds() 00038 { 00039 if (1) { 00040 if (playerShootSound) { 00041 playerShootSound = false; 00042 SoundController::playSample((prog_char*)shoot_snd, sizeof(shoot_snd),0); 00043 } 00044 if (alienDeathSound) { 00045 alienDeathSound = false; 00046 SoundController::playSample((prog_char*)aliendeath_snd, sizeof(aliendeath_snd),1); 00047 } 00048 if (playerDeathSound) { 00049 playerDeathSound = false; 00050 SoundController::playSample((prog_char*)playerdeath_snd, sizeof(playerdeath_snd),1); 00051 } 00052 if (saucerSound) { 00053 saucerSound = false; 00054 SoundController::playSample((prog_char*)saucer_snd, -sizeof(saucer_snd),2); 00055 } 00056 if (stopSaucerSnd) { 00057 stopSaucerSnd = false; 00058 SoundController::playSample((prog_char*)0,0,2); 00059 } 00060 if (saucerDieSound) { 00061 saucerDieSound = false; 00062 SoundController::playSample((prog_char*)playerdeath_snd, sizeof(playerdeath_snd),2); 00063 } 00064 if (alienBeatSound) { 00065 alienBeatSound = false; 00066 prog_char *b = 0; unsigned int n = 0; 00067 switch (alienBeat&3) { 00068 case 0: b = (prog_char*)beat1_snd; n = sizeof(beat1_snd); break; 00069 case 1: b = (prog_char*)beat2_snd; n = sizeof(beat2_snd); break; 00070 case 2: b = (prog_char*)beat3_snd; n = sizeof(beat3_snd); break; 00071 case 3: b = (prog_char*)beat4_snd; n = sizeof(beat4_snd); break; 00072 } 00073 SoundController::playSample(b,n,3); 00074 ++alienBeat; 00075 } 00076 SoundController::update(); 00077 } 00078 #if SYNTHSOUNDS 00079 else { 00080 if (playerShootSound) { 00081 playerShootSound = false; 00082 shootSound.adsr = ADSR(10,15,100,36); 00083 soundPlayer.setVolume(200).setSound(shootSound).play(440,100); 00084 } 00085 SoundController::update(); 00086 } 00087 #endif 00088 00089 }
Generated on Thu Jul 14 2022 11:20:53 by
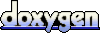