
Invaders game for the Gameduino
Embed:
(wiki syntax)
Show/hide line numbers
joystick.h
00001 #ifndef JOYSTICK_INCLUDED 00002 #define JOYSTICK_INCLUDED 00003 /*------------------------------------------------------------ 00004 Universal joystick driver for Gameduino 00005 00006 http://www.artlum.com/gameduino/gameduino.html#joystick 00007 00008 Important: Read the file "read_me_first.txt" *before* 00009 writing any code. Do it NOW! 00010 00011 Thanks go to 'Guy" for his contributions to this 00012 ------------------------------------------------------------*/ 00013 00014 //#include "WProgram.h" 00015 #include "arduino.h" 00016 00017 #define STICK_LEFT_BIT 0x01 00018 #define STICK_RIGHT_BIT 0x02 00019 #define STICK_UP_BIT 0x04 00020 #define STICK_DOWN_BIT 0x08 00021 #define ANALOG_STICK_BIT 0x80 00022 #define STICK_INFO_MASK (ANALOG_STICK_BIT) 00023 00024 class Joystick { 00025 byte buttons; // State of buttons, packed into a byte 00026 byte prev; // State of the buttons on previous read 00027 byte dpad; // State of Up/Down/Left/Right buttons plus some control flags 00028 signed char stickX,stickY;// Analogue x/y position 00029 char xCal,yCal; // Calibration 00030 public: 00031 // The constructor sets up the Arduino pins for input 00032 // and calibrates the joystick using the current position 00033 Joystick(); 00034 00035 // Read the current state 00036 void read(); 00037 00038 // Return "true" if analog X/Y position is available 00039 bool hasAnalogStick(); 00040 00041 // Digital joystick functions 00042 enum button_name { 00043 buttonA = 0x01, 00044 buttonB = 0x02, 00045 buttonC = 0x04, 00046 buttonX = 0x08, 00047 buttonY = 0x10, 00048 buttonZ = 0x20, 00049 buttonStart = 0x40, 00050 buttonSelect = 0x80 00051 }; 00052 // Test a named button 00053 // nb. You can combine button names to test multiple buttons at the same time 00054 bool isPressed(byte n) { return (buttons&n)!=0; } 00055 00056 // This tells you if a button changed between the last two calls to "read()" 00057 bool changed(byte n) { return bool((buttons^prev)&n); } 00058 00059 // Joystick up/down/left/right (or analog stick) 00060 bool left() { return (dpad&0x01)!=0; } 00061 bool right() { return (dpad&0x02)!=0; } 00062 bool up() { return (dpad&0x04)!=0; } 00063 bool down() { return (dpad&0x08)!=0; } 00064 00065 00066 // Analog joystick functions 00067 00068 // Force the analog joystick to recalibrate itself 00069 void recalibrate(); 00070 00071 // Current joystick position in range [-128..127] 00072 char analogX() { return stickX; } 00073 char analogY() { return stickY; } 00074 00075 // For debugging - show all state onscreen at (x,y) 00076 void dump(int x, int y); 00077 }; 00078 00079 00080 // JOYSTICK_INCLUDED 00081 #endif
Generated on Thu Jul 14 2022 11:20:53 by
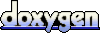