
Invaders game for the Gameduino
Embed:
(wiki syntax)
Show/hide line numbers
graphics.h
00001 /*--------------------------------------------- 00002 Send all graphics to the Gameduino 00003 ---------------------------------------------*/ 00004 #ifndef INVADER_GRAPHICS 00005 #define INVADER_GRAPHICS 00006 #include "shield.h" 00007 #include "mbed.h" 00008 #include "arduino.h" 00009 void makeGraphics(); 00010 00011 /*--------------------------------------------- 00012 Identifiers for custom chars 00013 ---------------------------------------------*/ 00014 enum char_id { 00015 CH_FLOOR=128, 00016 CH_PLAYERL, 00017 CH_PLAYERR 00018 }; 00019 /*--------------------------------------------- 00020 Identifiers for each sprite graphic 00021 00022 Sprites are made in four color mode so 00023 each 'sprite' can have four images inside 00024 it. 00025 00026 The first image is usually the 'normal' 00027 (eg. player/saucer) one and the third is 00028 usually blank (so you can hide the sprite). 00029 00030 The other two are used for animations 00031 (eg. invaders) and explosions (eg. player) 00032 ---------------------------------------------*/ 00033 enum graphic_id { 00034 // Invader sprites - Top, Middle, Bottom, two animation frames each... 00035 GR_INVADER_T, 00036 GR_INVADER_M, 00037 GR_INVADER_B, 00038 GR_BOMB_ZIGZAG, // Zigzag bomb 00039 GR_BOMB_BARS, // The bomb with rolling horizontal bars across it 00040 GR_BOMB_DIAG, // The bomb with diagonal bars across it 00041 GR_BOMB_OTHER, // Other bomb graphics (explosion and blank) 00042 // The player (with bullet) 00043 GR_PLAYER, 00044 GR_BULLET, // nb. Has a '0' in frame 2 (for the saucer...) 00045 // The saucer at the top 00046 GR_SAUCER, 00047 GR_SAUCER_SCORE, 00048 // Shields 00049 GR_SHIELD1, 00050 GR_SHIELD2, 00051 GR_SHIELD3, 00052 GR_SHIELD4 00053 }; 00054 00055 /*--------------------------------------------- 00056 Functions for wrecking/rebuilding shields 00057 ---------------------------------------------*/ 00058 void remakeShields(); 00059 00060 // Damage the shield with either a bomb or a bullet (ie. above/below) 00061 // n=shield number [0..4], x is relative to the shield's top-left corner 00062 int8_t zapShield(int8_t n, int8_t x, bool withBullet); // Return Y coordinate of blast 00063 00064 00065 #endif 00066
Generated on Thu Jul 14 2022 11:20:53 by
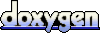