Library to allow the use of Arduino specific language with the mbed
Dependents: Gameduino Factory_monitor_0v1 mbed_mindflex NEW_NFC_TEST
arduino.h
00001 /** 00002 * @section LICENSE 00003 * Copyright (c) 2012 Chris Dick 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 * 00023 * @section DESCRIPTION 00024 * This is a library for allowing the use of programs written for the Arduino 00025 * with the mbed. This was started for use with the Gameduino shield Library 00026 * 00027 * @file "arduino.h" 00028 */ 00029 #ifndef ARDUINO_H 00030 #define ARDUINO_H 00031 00032 #include "mbed.h" 00033 #include "math.h" 00034 // Macros 00035 00036 #define pgm_read_word(x) (*(const short int*)x) 00037 #define pgm_read_dword_near(x) (*(const int*)x) 00038 #define pgm_read_word_near(x) (*(const unsigned int*)x) 00039 #define pgm_read_int_near(x) (*(const int*)x) 00040 #define pgm_read_int(x) (*(const int*)x) 00041 #define pgm_read_byte(x) (*(const char*)x) 00042 #define pgm_read_byte_near(x) (*(const char*)x) 00043 #define PROGMEM const 00044 #define char(x) ((char)x) 00045 #define byte(x) ((byte)x) 00046 #define int(x) ((int)x) 00047 #define word(x) ((word)x) 00048 #define long(x) ((long)x) 00049 #define float(x) ((float)x) 00050 00051 /** Macro for delay() 00052 * 00053 * @param void 00054 */ 00055 #define delay(x) (wait_ms(x)) 00056 /** Macro for delayMicroseconds() 00057 * 00058 * @param void 00059 */ 00060 #define delayMicroseconds(x) (wait_us(x)) 00061 00062 /** Macro for min() 00063 * 00064 * @param any 00065 */ 00066 #define min(a,b) ((a)<(b)?(a):(b)) 00067 /** Macro for max() 00068 * 00069 * @param any 00070 */ 00071 #define max(a,b) ((a)>(b)?(a):(b)) 00072 /** Macro for abs() 00073 * 00074 * @param any 00075 */ 00076 #define abs(x) ((x)>0?(x):(x*-1)) 00077 00078 /** Macro for randomSeed() 00079 * 00080 * @param int 00081 */ 00082 #define randomSeed(x) srand(x) 00083 00084 00085 // typedefs 00086 00087 typedef unsigned char prog_uint8_t; 00088 typedef unsigned int prog_uint16_t; 00089 typedef unsigned int prog_uint32_t; 00090 typedef unsigned char byte; 00091 typedef bool boolean; 00092 typedef unsigned char prog_uchar; 00093 typedef signed char prog_char; 00094 typedef signed long int word; 00095 00096 // function prototypes 00097 00098 void timer_start(void); 00099 long millis(void); 00100 long micros(void); 00101 byte lowByte(short int low); 00102 byte highByte(short int high); 00103 int random(int number); 00104 int random(int numberone, int numbertwo); 00105 #endif
Generated on Wed Jul 13 2022 19:09:56 by
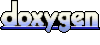