Library to allow the use of Arduino specific language with the mbed
Dependents: Gameduino Factory_monitor_0v1 mbed_mindflex NEW_NFC_TEST
arduino.c
00001 /** 00002 * @section LICENSE 00003 * Copyright (c) 2012 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 * 00023 * @section DESCRIPTION 00024 * This is a library for allowing the use of programs written for the Arduino 00025 * with the mbed. This was started for use with the Gameduino shield Library. 00026 * It is currently uncomplete and not fully tested, but I don't see myself spending time on it. 00027 * See TODOs and check http://arduino.cc/en/Reference/HomePage for missing parts 00028 * 00029 * @file arduino.c 00030 * 00031 * Example: 00032 * @code 00033 * 00034 * #include "mbed.h" 00035 * #include "arduino.h" 00036 * 00037 * void setup(){ 00038 * // setup code 00039 * } 00040 * 00041 *void loop(){ 00042 * // loop code 00043 *} 00044 * 00045 * int main() { 00046 * timer_start(); 00047 * setup(); 00048 * while(1) { 00049 * loop(); 00050 * } 00051 * } 00052 * @endcode 00053 */ 00054 #include "arduino.h " 00055 00056 /***************************************************** 00057 Digital I/O 00058 00059 pinMode()- TODO 00060 digitalWrite()- TODO 00061 digitalRead()- TODO 00062 00063 ******************************************************/ 00064 00065 /***************************************************** 00066 Analog I/O 00067 00068 analogReference() - TODO 00069 analogRead() - TODO 00070 analogWrite()-(PWM) - TODO 00071 00072 *****************************************************/ 00073 00074 /***************************************************** 00075 Advanced I/O 00076 00077 tone() - TODO 00078 noTone() - TODO 00079 shiftOut() - TODO 00080 shiftIn() - TODO 00081 pulseIn() - TODO 00082 00083 *****************************************************/ 00084 00085 /***************************************************** 00086 Time 00087 00088 millis() - need to start timer first 00089 micros() - need to start timer first 00090 delay() - done by Macro 00091 delayMicroseconds() - Done byMacro 00092 00093 *****************************************************/ 00094 00095 Timer arduino_timer; 00096 /** start the arduino_timer timer for millis() and micros() running. 00097 * 00098 * @param void 00099 */ 00100 void timer_start(void) { 00101 arduino_timer.start(); 00102 } 00103 /** return a long for the amount of time since the timer was started in milliseconds. 00104 * 00105 * @param void 00106 */ 00107 long millis(void) { 00108 return arduino_timer.read_ms(); 00109 } 00110 /** return a long for the amount of time since the timer was started in microseconds. 00111 * 00112 * @param void 00113 */ 00114 long micros(void) { 00115 return arduino_timer.read_us(); 00116 } 00117 00118 00119 /***************************************************** 00120 Maths 00121 00122 min() - done by Macro 00123 max() - done by Macro 00124 abs() - done by Macro 00125 constrain() - TODO 00126 map() - TODO 00127 pow() - implemented by including math.h 00128 sqrt() - implemented by including math.h 00129 00130 *****************************************************/ 00131 00132 00133 /***************************************************** 00134 Trigonometry 00135 00136 sin() - implemented by including math.h 00137 cos() - implemented by including math.h 00138 tan() - implemented by including math.h 00139 00140 *****************************************************/ 00141 00142 /***************************************************** 00143 Random Numbers 00144 00145 randomSeed() - done by Macro 00146 random() - function below 00147 00148 *****************************************************/ 00149 00150 /** generates a random number from 0 to defined number 00151 * 00152 * @param number maximum value for random number 00153 */ 00154 int random(int number) { 00155 return (rand()%number); 00156 } 00157 /** generates a random number between two numbers 00158 * 00159 * @param numberone minimum value for random number 00160 * @param numbertwo maximum value for random number 00161 */ 00162 int random(int numberone, int numbertwo) { 00163 int random = 0; 00164 if ((numberone < 0) && (numbertwo < 0)) { 00165 numberone = numberone * -1; 00166 numbertwo = numbertwo * -1; 00167 random = -1 * (rand()%(numberone + numbertwo)); 00168 } 00169 if ((numbertwo < 0) && (numberone >= 0)) { 00170 numbertwo = numbertwo * -1; 00171 random = (rand()%(numberone + numbertwo)) - numbertwo; 00172 } 00173 if ((numberone < 0) && (numbertwo >= 0)) { 00174 numberone = numberone * -1; 00175 random = (rand()%(numberone + numbertwo)) - numberone; 00176 } else { 00177 random = (rand()%(numberone + numbertwo)) - min(numberone, numbertwo); 00178 } 00179 return random; 00180 } 00181 00182 /***************************************************** 00183 Bits and Bytes 00184 00185 lowByte() - function below 00186 highByte() - function below 00187 bitRead() - TODO 00188 bitWrite() - TODO 00189 bitSet() - TODO 00190 bitClear() - TODO 00191 bit() - TODO 00192 00193 *****************************************************/ 00194 00195 /** returns the lower nibble of first byte 00196 * 00197 * @param high byte of which the high nibble is required 00198 */ 00199 byte lowByte(short int low) { 00200 byte bytelow = 0; 00201 bytelow = (low & 0xFF); 00202 return bytelow; 00203 } 00204 /** returns the higher nibble of first byte 00205 * 00206 * @param high byte of which the high nibble is required 00207 */ 00208 byte highByte(short int high) { 00209 byte bytehigh = 0; 00210 bytehigh = ((high >> 8) & 0xFF); 00211 return bytehigh; 00212 } 00213 00214 00215 00216 /***************************************************** 00217 External Interrupts 00218 00219 attachInterrupt() - TODO 00220 detachInterrupt() - TODO 00221 00222 *****************************************************/ 00223 00224 /***************************************************** 00225 Interrupts 00226 00227 interrupts() - TODO 00228 noInterrupts() - TODO 00229 00230 *****************************************************/ 00231 00232 /***************************************************** 00233 Communication 00234 00235 Serial - TODO 00236 Stream - TODO 00237 00238 *****************************************************/
Generated on Wed Jul 13 2022 19:09:56 by
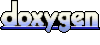