
The calibration code for our robot project
Dependencies: MODSERIAL QEI mbed
main.cpp
00001 #include "mbed.h" 00002 #include "QEI.h" 00003 #include "MODSERIAL.h" 00004 //#include <"math.h"> 00005 00006 QEI motor1(D13,D12,NC, 624); 00007 MODSERIAL pc(USBTX,USBRX); 00008 DigitalOut direction1(D7); 00009 PwmOut speed1(D6); 00010 DigitalIn button1(PTC6); 00011 00012 int main() 00013 { 00014 DigitalOut led(LED_RED); 00015 pc.baud(115200); 00016 int activation=0;//int for starting calibration 00017 float cycle = 0.6;//define the speed of the motor 00018 int first = 0; 00019 while(1){ 00020 if (button1.read()==0){ 00021 activation = 1;//acivate the int if the button is pressed 00022 } 00023 00024 //pc.printf("%d\n",activation);//test if the button is activated 00025 //wait(1); 00026 00027 if(activation == 1){ 00028 DigitalOut led(LED_BLUE); 00029 direction1.write(false);//turn motor CCW or CW if set to 0 00030 //motor CW = 0 00031 //motor CCW = 1 00032 speed1.period_us(100);//Set period of PWM to 100 us. 00033 speed1.write(cycle);//write the speed to the motor 00034 00035 00036 if(first==0){//on the first loop wait a moment for the motor to start. 00037 wait(3); 00038 first=1; 00039 } 00040 //set up the speed calculation of the motor 00041 wait(0.1); 00042 int pos1=motor1.getPulses(); 00043 //pc.printf("position 1 is: %d\n",pos1);//print position 1 00044 wait(0.1); 00045 int pos2=motor1.getPulses(); 00046 //pc.printf("position 2 is: %d\n",pos2);//print position 1 00047 int diff = pos2 - pos1;//check if the encoder count stops changing 00048 int rads = abs(diff/0.1); 00049 pc.printf("the speed is : %d counts/second\n", rads);//print out the speed 00050 if(diff == 0){ 00051 cycle=0;//speed of 0 to stop motor 00052 speed1.write(cycle);//write the new speed to the motor 00053 motor1.reset();//reset the motor position 00054 int posnew=motor1.getPulses(); 00055 pc.printf("The new position is: %d\n",posnew); 00056 activation=0; 00057 break; 00058 } 00059 } 00060 } 00061 } 00062 //determine speed of movement from the encoder signal 00063 // the amount of counts for one revolution is 32 00064 //this is X2 encodng, as the QEI uses X2 by default 00065 //and the motor encoder has a X4 encoder and thus 64 counts per revolution 00066 //thus keeping in mind the gearbox with 1:131,25 gear ratio: 00067 // the amount of counts for a gearbox revolution or resolution is 8400 00068 // a revolution is 2 pi rad, resolution is : 8400/2 pi = 1336.90 counts/rad 00069 //or for the encoder x2 its 668,45 counts/rad 00070 /* 00071 Rotational position in degrees can be calculated by: 00072 * 00073 * (pulse count / X * N) * 360 00074 * 00075 * Where X is the encoding type [e.g. X4 encoding => X=4], and N is the number 00076 * of pulses per revolution. 00077 * 00078 * Linear position can be calculated by: 00079 * 00080 * (pulse count / X * N) * (1 / PPI) 00081 * 00082 * Where X is encoding type [e.g. X4 encoding => X=44], N is the number of 00083 * pulses per revolution, and PPI is pulses per inch, or the equivalent for 00084 * any other unit of displacement. PPI can be calculated by taking the 00085 * circumference of the wheel or encoder disk and dividing it by the number 00086 * of pulses per revolution. 00087 00088 * Reset the encoder. 00089 * 00090 * Sets the pulses and revolutions count to zero. 00091 00092 void reset(void); 00093 */ 00094
Generated on Sun Jul 24 2022 08:22:55 by
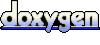