Library for reading out the LCM 101 sensor and also usable for S610 loadcells
Fork of LCM101 by
lcm101.h
00001 #ifndef _LCM101_H_ 00002 #define _LCM101_H_ 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * Simple class to read out an LCM101 S-beam force sensor connected to an analog 00008 * input. 00009 */ 00010 class Lcm101 { 00011 public: 00012 00013 /** 00014 * @param pin_a_in PinName of analog input 00015 * @param offset of analog value (calibration data) 00016 * @param factor multiplication factor for analog value (calibration data) 00017 */ 00018 Lcm101 (PinName pin_a_in, float offset, float factor) : 00019 analog_in_(pin_a_in), 00020 kOffset_(offset), 00021 kFactor_(factor) 00022 { 00023 } 00024 00025 /** 00026 * @return unscaled analog input value 00027 */ 00028 float getForceRaw () 00029 { 00030 return analog_in_.read(); 00031 } 00032 00033 /** 00034 * @return force value kOffset_ + kFactor_ * getForceRaw(); 00035 */ 00036 float getForce () 00037 { 00038 return kOffset_ + kFactor_ * getForceRaw (); 00039 //it appears that higher order polynomials show better accuracy, within the 40 kg range that is. 00040 // Awaiting other instructions, a linear fit will be currently used however. 00041 } 00042 00043 /** 00044 * @return ffset of analog value 00045 */ 00046 float get_offset () { return kOffset_; } 00047 00048 /** 00049 * @return factor multiplication factor for analog value 00050 */ 00051 float get_factor () { return kFactor_; } 00052 00053 00054 private: 00055 AnalogIn analog_in_; 00056 00057 const float kOffset_; 00058 const float kFactor_; 00059 }; 00060 00061 #endif
Generated on Thu Jul 14 2022 23:40:15 by
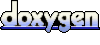