Tool for playing 8bit like music on Piezo speaker.
PlayTone Class Reference
Class for playing 8bit-like songs on PWM speaker. More...
#include <PlayTone.h>
Public Member Functions | |
PlayTone (PinName PS) | |
Constructor, chosen pin must be PWM compatible. | |
int | setBPM (int) |
Function for setting BPM. | |
void | transpose (int) |
Function for transposing whole set of notes. | |
void | playTone (int, float) |
Function for playing single tone. | |
void | playSequence (int, int[], float[]) |
Function for playing sequence of tones. | |
void | playSequence (int, Note[]) |
Function for playing sequence of tones. | |
void | setStaccatoDuty (float) |
Function for setting constant staccato note duty cycle based on BPM and independently on note value. | |
void | playStaccato (int, float) |
Function for playing single tone in Staccato. | |
void | playStaccatoSequence (int, int[], float[]) |
Function for playing sequence of tones in Staccato. | |
void | playStaccatoSequence (int, Note[]) |
Function for playing sequence of tones in Staccato. | |
void | silence (float) |
Function for playing rest of given length based on set BPM. | |
void | stop () |
Function for stopping sounds. |
Detailed Description
Class for playing 8bit-like songs on PWM speaker.
This class suports easy conversion from notes to sound from piezo speaker.
Currently tested with LPC1768.
Writen by: Jan Crha (TeaPack_CZ), 2016.
Last modified: 2016-10-02
Example:
#include "mbed.h" #include "PlayTone.h" #include "Popcorn.h" PlayTone Pt(p21); Popcorn Pop; int main() { int PopLen = Pop.GetLen(); int PopBPM = Pop.GetBPM(); Note PopcornTheme[PopLen]; Pop.GetPopcorn(PopcornTheme); Pt.setBPM(PopBPM); Pt.setStaccatoDuty(1/16.0); Pt.playStaccatoSequence(PopLen, PopcornTheme); }
Definition at line 53 of file PlayTone.h.
Constructor & Destructor Documentation
PlayTone | ( | PinName | PS ) |
Constructor, chosen pin must be PWM compatible.
Definition at line 3 of file PlayTone.cpp.
Member Function Documentation
void playSequence | ( | int | sequence_length, |
int | notes[], | ||
float | values[] | ||
) |
Function for playing sequence of tones.
- Parameters:
-
sequence_length length of sequence to play notes[] list of notes indexes values[] list of note lengths based from setted BPM
Definition at line 134 of file PlayTone.cpp.
void playSequence | ( | int | sequence_length, |
Note | notes[] | ||
) |
Function for playing sequence of tones.
- Parameters:
-
sequence_length length of sequence Notes[] list of Notes
Definition at line 145 of file PlayTone.cpp.
void playStaccato | ( | int | ind, |
float | value | ||
) |
Function for playing single tone in Staccato.
- See also:
- playTone()
- setStaccatoDuty()
Definition at line 107 of file PlayTone.cpp.
void playStaccatoSequence | ( | int | sequence_length, |
int | notes[], | ||
float | values[] | ||
) |
Function for playing sequence of tones in Staccato.
- See also:
- playSequence()
- setStaccatoDuty()
Definition at line 156 of file PlayTone.cpp.
void playStaccatoSequence | ( | int | sequence_length, |
Note | notes[] | ||
) |
Function for playing sequence of tones in Staccato.
- See also:
- playSequence()
- setStaccatoDuty()
Definition at line 167 of file PlayTone.cpp.
void playTone | ( | int | ind, |
float | value | ||
) |
Function for playing single tone.
- Parameters:
-
index of note from list length of note based from setted BPM
Definition at line 97 of file PlayTone.cpp.
int setBPM | ( | int | Bpm ) |
Function for setting BPM.
- Parameters:
-
Bpm range is from 40 to 260
- Returns:
- Duration of note in ms
Definition at line 178 of file PlayTone.cpp.
void setStaccatoDuty | ( | float | duty ) |
Function for setting constant staccato note duty cycle based on BPM and independently on note value.
- Parameters:
-
duty in range from 0 to 1
Definition at line 188 of file PlayTone.cpp.
void silence | ( | float | value ) |
Function for playing rest of given length based on set BPM.
Definition at line 119 of file PlayTone.cpp.
void stop | ( | ) |
Function for stopping sounds.
Definition at line 124 of file PlayTone.cpp.
void transpose | ( | int | level ) |
Function for transposing whole set of notes.
Definition at line 129 of file PlayTone.cpp.
Generated on Tue Jul 12 2022 20:54:31 by
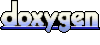