
More HACMan stuff again
Dependencies: FatFileSystem SDFileSystem mbed
txtFile.cpp
00001 #include "txtFile.h" 00002 00003 00004 /** 00005 * Constructor when called opens a file 00006 * 00007 * @param fileAddr 00008 * the address of the file, either local, sd or whatever 00009 * @param readWrite 00010 * whether reading or writing to the file 00011 */ 00012 TxtFile::TxtFile(char fileAddr[], char *readWrite, bool doParseFile) { 00013 00014 isFileOpen = false; 00015 isFileParsed = false; 00016 currentFilePos = 0; 00017 00018 if ((fp = fopen(fileAddr, readWrite)) == NULL) { 00019 isFileOpen = false; 00020 } else { 00021 isFileOpen = true; 00022 } 00023 00024 if(doParseFile) { 00025 parseFile(); 00026 isFileParsed = true; 00027 } 00028 } 00029 00030 /** 00031 * Destructor when called closes the open file 00032 */ 00033 TxtFile::~TxtFile() { 00034 closeFile(); 00035 } 00036 00037 /** 00038 * Returns true if the file is open 00039 * 00040 * @returns isFileOpen 00041 */ 00042 bool TxtFile::isOpen() { 00043 return isFileOpen; 00044 } 00045 00046 /** 00047 * Returns true if file has been parsed 00048 * 00049 * @returns isFileParsed 00050 */ 00051 bool TxtFile::isParsed() { 00052 return isFileParsed; 00053 } 00054 00055 /** 00056 * Checks if the file is open, and closes it if it is 00057 * 00058 * @returns isClosed 00059 * returns true if a file was closed, false if not 00060 */ 00061 bool TxtFile::closeFile() { 00062 if (isOpen()) { 00063 fclose(fp); 00064 return true; 00065 } else 00066 return false; 00067 } 00068 00069 /** 00070 * Counts the number of lines, and sets line start positions 00071 */ 00072 void TxtFile::parseFile() { 00073 int c = 0; //current character 00074 int cPrev = 0; //previous character 00075 int lengthCount = 0; //local length counter 00076 int lineCount = 0; //local line counter 00077 int curPos = 0; //current position in file 00078 00079 if (!isFileParsed) { //check to see if not already done 00080 seekPos(0); //seek to beginning of file 00081 00082 c = fgetc(fp); //get first character 00083 fileLineStart[lineCount] = curPos; //first line ALWAYS starts at zero... so far anyway 00084 00085 while (c != EOF) { //while not at the end of the file 00086 if ((c != '\n') && (c != '\r')) { //if not a newline or carriage return 00087 lengthCount++; //increment length counter 00088 } 00089 00090 if (((c == '\n') && (cPrev == '\r')) || ((c == '\r') && (cPrev == '\n'))) { //if a newline (\r\n or \n\r) 00091 fileLineLength[lineCount] = lengthCount; //set fileLineLength for current line to lengthCount 00092 lineCount++; //increment line counter 00093 lengthCount = 0; //reset lengthCount 00094 fileLineStart[lineCount] = curPos + 1; //set file line start to next position - may not need the +1? 00095 } 00096 curPos++; 00097 cPrev = c; 00098 c = getc(fp); 00099 } 00100 00101 noLines = lineCount; 00102 00103 isFileParsed = true; 00104 seekPos(0); //seek back to the beginning of the file 00105 } 00106 } 00107 00108 /** 00109 * Returns the number of lines in the file 00110 * 00111 * @returns noLines 00112 */ 00113 int TxtFile::lineCount() ( 00114 return noLines; 00115 } 00116 00117 /** 00118 * Seeks to the position in the current opened file 00119 * 00120 * @params seekLoc 00121 * byte location to seek to 00122 */ 00123 void TxtFile::seekPos(int seekLoc) { 00124 fseek(fp, seekLoc, SEEK_SET); 00125 currentFilePos = seekLoc; 00126 } 00127 00128 int TxtFile::getPos() { 00129 return currentFilePos; 00130 } 00131 00132 int TxtFile::seekLineStart() { 00133 int backCount = 0; 00134 int c = 0; 00135 00136 c = fgetc(fp); //get character at current position 00137 00138 while ((c != '\n') && (c != '\r')) { //check if character is a newline or carriage return 00139 backCount++; //increment backCount 00140 fseek(fp, -2, SEEK_CUR); //seek back 2, 1 for the fgetc, 1 more to actually go back one 00141 c = fgetc(fp); //get a new character 00142 } 00143 currentFilePos -= backCount; 00144 return backCount; 00145 } 00146 00147 int TxtFile::seekLineEnd() { 00148 int count = 0; 00149 int c = 0; 00150 00151 c = fgetc(fp); //get character at position after the newline or carriage return 00152 00153 while ((c != '\n') && (c != '\r')) { //check if character is a newline or carriage return 00154 count++; //increment main counter 00155 c = fgetc(fp); //get a new character 00156 } 00157 currentFilePos += count; 00158 return count; 00159 } 00160 00161 void TxtFile::seekLine(int line) { 00162 if(isFileParsed) { 00163 seekPos(fileLineStart[line - 1]); 00164 } 00165 } 00166 00167 /** 00168 * Returns the line length of the current line the 'seek head' is on 00169 * 00170 * @returns lineLength 00171 */ 00172 int TxtFile::lineLength() { 00173 int count; //initialise all values needed 00174 00175 seekLineStart(); 00176 00177 count = seekLineEnd(); 00178 00179 return count; //return count, which is the number of characters in the line 00180 } 00181 00182 /** 00183 * 00184 */ 00185 void csvToIntArray(int line, int arrayStart, int arrayEnd, int *array) { 00186 00187 00188 // int i = char a - '0' <---- really easy way to convert char to int. :D 00189 }
Generated on Sun Jul 17 2022 08:25:42 by
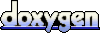