
More HACMan stuff again
Dependencies: FatFileSystem SDFileSystem mbed
ledSign.cpp
00001 #include "ledSign.h" 00002 00003 // Sign Output Pins 00004 00005 BusOut address(p17, p18, p19, p20); // Address 0 to 16 00006 BusOut colour(p15, p16); // 0 = off, 1 = red, 2 = green, 3 = orange 00007 00008 DigitalOut abTop(p14); // bank A or B switch for Top Row - 0 = A, 1 = B 00009 DigitalOut clkTop(p13); // clock for Top Row 00010 DigitalOut weTop(p28); // Write Enable for Top Row 00011 DigitalOut aeTop(p27); // Address Enable for Top Row 00012 DigitalOut enbTop(p26); // Enable for Top Row 00013 00014 DigitalOut abBot(p25); // bank A or B switch for Bottom Row - 0 = A, 1 = B 00015 DigitalOut clkBot(p24); // clock for Bottom Row 00016 DigitalOut weBot(p23); // Write Enable for Bottom Row 00017 DigitalOut aeBot(p22); // Address Enable for Bottom Row 00018 DigitalOut enbBot(p21); // Enable for Bottom Row 00019 00020 LedSign::LedSign() { 00021 address = 0; 00022 colour = 0; 00023 abTop = 0; 00024 clkTop = 0; 00025 weTop = 0; 00026 aeTop = 0; 00027 enbTop = 0; 00028 abBot = 0; 00029 clkBot = 0; 00030 weBot = 0; 00031 aeBot = 0; 00032 enbBot = 0; 00033 } 00034 00035 void LedSign::enable() { 00036 enbTop = 1; 00037 enbBot = 1; 00038 } 00039 00040 void LedSign::disable() { 00041 enbTop = 0; 00042 enbBot = 0; 00043 } 00044 00045 void LedSign::swapBank() { 00046 (abTop) ? abTop = 0 : abTop = 1; 00047 (abBot) ? abBot = 0 : abBot = 1; 00048 } 00049 00050 void LedSign::writeRow(int * pointer, int wRow) { 00051 if(wRow <= 15) { 00052 for(int col = 0; col < 128; col++) { 00053 colour = *(pointer + col); 00054 clockTop(); 00055 } 00056 00057 writeTop(wRow); 00058 } 00059 if(wRow >= 16) { 00060 for(int col = 0; col < 128; col++) { 00061 colour = *(pointer + col); 00062 clockBot(); 00063 } 00064 00065 writeBot(wRow - 16); 00066 } 00067 } 00068 00069 void LedSign::writeScreenColour(int newColour) { 00070 colour = newColour; //set colour to write to screen 00071 for (int i=0; i<128; i++) { // clock in 128 bits to turn all the LED's on 00072 clockIn(); 00073 } 00074 00075 for (int i=0; i<16; i++) { //actually write them for all lines 00076 writeTop(i); 00077 writeBot(i); 00078 } 00079 00080 swapBank(); 00081 00082 } 00083 00084 //********************Private Declerations******************** 00085 00086 void LedSign::writeTop(int topAddress) { 00087 address = topAddress; 00088 aeTop = 1; 00089 wait_us(1); 00090 weTop = 1; 00091 wait_us(1); 00092 weTop = 0; 00093 wait_us(1); 00094 aeTop = 0; 00095 wait_us(1); 00096 } 00097 00098 void LedSign::writeBot(int botAddress) { 00099 address = botAddress; 00100 aeBot = 1; 00101 wait_us(1); 00102 weBot = 1; 00103 wait_us(1); 00104 weBot = 0; 00105 wait_us(1); 00106 aeBot = 0; 00107 wait_us(1); 00108 } 00109 00110 void LedSign::clockTop() { 00111 wait_us(1); 00112 clkTop = 1; 00113 wait_us(1); 00114 clkTop = 0; 00115 wait_us(1); 00116 } 00117 00118 void LedSign::clockBot() { 00119 wait_us(1); 00120 clkBot = 1; 00121 wait_us(1); 00122 clkBot = 0; 00123 wait_us(1); 00124 } 00125 00126 void LedSign::clockIn() { 00127 wait_us(1); 00128 clkTop = 1; 00129 clkBot = 1; 00130 wait_us(1); 00131 clkTop = 0; 00132 clkBot = 0; 00133 wait_us(1); 00134 }
Generated on Sun Jul 17 2022 08:25:42 by
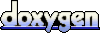