Updated
Embed:
(wiki syntax)
Show/hide line numbers
BMP280.h
00001 /** 00002 * BME280 Combined humidity and pressure sensor library 00003 * 00004 * @author Toyomasa Watarai 00005 * @version 1.0 00006 * @date 06-April-2015 00007 * 00008 * Library for "BME280 temperature, humidity and pressure sensor module" from Switch Science 00009 * https://www.switch-science.com/catalog/2236/ 00010 * 00011 * For more information about the BME280: 00012 * http://ae-bst.resource.bosch.com/media/products/dokumente/bme280/BST-BME280_DS001-10.pdf 00013 */ 00014 00015 #ifndef MBED_BMP280_H 00016 #define MBED_BMP280_H 00017 00018 #include "mbed.h" 00019 00020 //#define _DEBUG 00021 // default address with SDO High 0x77 00022 // address with SDO LOW 0x76 00023 #define DEFAULT_SLAVE_ADDRESS (0x77) 00024 00025 #ifdef _DEBUG 00026 extern Serial pc; 00027 #define DEBUG_PRINT(...) pc.printf(__VA_ARGS__) 00028 #else 00029 #define DEBUG_PRINT(...) 00030 #endif 00031 00032 00033 /** BME280 class 00034 * 00035 * BME280: A library to read environmental data using Bosch BME280 device 00036 * Readds temperature and pressure 00037 * 00038 * BME280 is an environmental sensor 00039 * @endcode 00040 */ 00041 00042 class BMP280 00043 { 00044 public: 00045 00046 /** Create a BME280 instance 00047 * which is connected to specified I2C pins with specified address 00048 * 00049 * @param sda I2C-bus SDA pin 00050 * @param scl I2C-bus SCL pin 00051 * @param slave_adr (option) I2C-bus address (default: 0x77) 00052 */ 00053 BMP280(PinName sda, PinName sck, char slave_adr = DEFAULT_SLAVE_ADDRESS); 00054 00055 /** Create a BME280 instance 00056 * which is connected to specified I2C pins with specified address 00057 * 00058 * @param i2c_obj I2C object (instance) 00059 * @param slave_adr (option) I2C-bus address (default: 0x77) 00060 */ 00061 BMP280(I2C &i2c_obj, char slave_adr = DEFAULT_SLAVE_ADDRESS); 00062 00063 /** Destructor of BME280 00064 */ 00065 virtual ~BMP280(); 00066 00067 /** Initializa BME280 sensor 00068 * 00069 * Configure sensor setting and read parameters for calibration 00070 * 00071 */ 00072 void initialize(void); 00073 00074 /** Read the current temperature value (degree Celsius) from BME280 sensor 00075 * 00076 */ 00077 float getTemperature(void); 00078 00079 /** Read the current pressure value (hectopascal)from BME280 sensor 00080 * 00081 */ 00082 float getPressure(void); 00083 00084 /** Read the current humidity value (humidity %) from BME280 sensor 00085 * 00086 */ 00087 // float getHumidity(void); 00088 00089 private: 00090 00091 I2C *i2c_p; 00092 I2C &i2c; 00093 char address; 00094 uint16_t dig_T1; 00095 int16_t dig_T2, dig_T3; 00096 uint16_t dig_P1; 00097 int16_t dig_P2, dig_P3, dig_P4, dig_P5, dig_P6, dig_P7, dig_P8, dig_P9; 00098 uint16_t dig_H1, dig_H3; 00099 int16_t dig_H2, dig_H4, dig_H5, dig_H6; 00100 int32_t t_fine; 00101 00102 }; 00103 00104 #endif // MBED_BME280_H
Generated on Thu Jul 14 2022 19:46:16 by
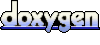