
Sample TFT to KL25Z program.
Dependencies: SPI_STMPE610 TFT_fonts UniGraphic mbed
Fork of TFT_test_frdm-kl25z by
main.cpp
00001 /******************************************************************* 00002 * TFT Sample program for STEM project 00003 * By Henry Foley, Feb 2017 00004 * 00005 * Hardware: Freedom KL25Z uC development board with a TFT 2.8" 00006 * resistive touch display shield from Adafruit (1651). 00007 * 00008 */ 00009 00010 #include "mbed.h" 00011 #include "ILI9341.h" 00012 #include "SPI_STMPE610.h" 00013 #include "Arial12x12.h" 00014 #include "Arial24x23.h" 00015 #include "Arial28x28.h" 00016 #include "Verdana22x21-16.h" 00017 00018 #define PIN_XP PTB3 00019 #define PIN_XM PTB1 00020 #define PIN_YP PTB2 00021 #define PIN_YM PTB0 00022 #define PIN_MOSI PTD2 00023 #define PIN_MISO PTD3 00024 #define PIN_SCLK PTD1 00025 #define PIN_CS_TFT PTD0 00026 #define PIN_DC_TFT PTD5 00027 #define PIN_BL_TFT PTC9 00028 #define PIN_CS_SD PTA4 00029 #define PIN_CS_TSC PTA13 00030 #define PIN_RESET_TFT PTB10 00031 00032 00033 00034 DigitalOut backlight(PTA12) ; 00035 00036 SPI_STMPE610 TSC(PIN_MOSI, PIN_MISO, PIN_SCLK, PIN_CS_TSC) ; 00037 00038 ILI9341 TFT(SPI_8, 10000000, 00039 PIN_MOSI, PIN_MISO, PIN_SCLK, 00040 PIN_CS_TFT, PIN_RESET_TFT, PIN_DC_TFT, "Adafruit2.8") ; 00041 00042 void initTFT(void); 00043 void drawScreen(void); 00044 uint16_t map(uint16_t, uint16_t, uint16_t, uint16_t, uint16_t); 00045 void display_xy(void); 00046 00047 int main() 00048 { 00049 static uint16_t x, y, z ; 00050 uint16_t temp_x; 00051 int touched = 0; 00052 00053 initTFT() ; 00054 00055 drawScreen(); 00056 00057 while(true) 00058 { 00059 touched = TSC.getRAWPoint(&x, &y, &z); 00060 00061 if(touched) // any touch 00062 { 00063 00064 // scale the raw values to match the screen orentation and 320(x = horiz) 240(y = vert) size, 0,0 = upper left corner 00065 temp_x = x; 00066 x = map(y,180, 3800,0,320); 00067 y = map(temp_x,3800,280,0,240); 00068 if( (x > 0 && x < 320) && ( y > 0 && y < 240 )) // if valid values 00069 { 00070 drawScreen(); 00071 00072 TFT.BusEnable(true) ; 00073 TFT.background(Blue); 00074 wait(0.2); 00075 TFT.foreground(White); 00076 TFT.locate(120, 160); 00077 TFT.printf("x%3d", x); 00078 TFT.locate(120, 190); 00079 TFT.printf("y%3d", y); 00080 TFT.BusEnable(false) ; 00081 wait(0.5); // to reduce mutiple hits from one touch 00082 } 00083 00084 } 00085 } 00086 } // end of main() 00087 00088 void initTFT(void) 00089 { 00090 //Configure the display driver 00091 TFT.BusEnable(true) ; 00092 TFT.set_orientation(1); 00093 backlight = 1 ; 00094 TFT.background(Blue); 00095 wait(0.2); 00096 TFT.foreground(White); 00097 wait(0.2); 00098 TFT.cls(); 00099 wait(0.2); 00100 TFT.set_font((unsigned char*) Arial28x28); 00101 wait(0.2); 00102 TFT.locate(15, 10); 00103 TFT.printf(" TFT Test "); 00104 TFT.locate(15, 100); 00105 TFT.BusEnable(false); 00106 wait(5); 00107 } 00108 00109 00110 void drawScreen(void) 00111 { 00112 TFT.BusEnable(true); 00113 TFT.foreground(White); // Set default text colors 00114 TFT.background(Blue); 00115 TFT.cls(); // wipe the screen clean and set bg colors 00116 wait(0.1); 00117 00118 TFT.foreground(Black); 00119 TFT.set_font((unsigned char*) Arial24x23); 00120 TFT.fillcircle( 60, 175, 50, Yellow); 00121 TFT.background(Yellow) ; 00122 TFT.locate(25, 160); 00123 TFT.printf(" A "); 00124 00125 TFT.fillcircle( 160, 105, 50, Cyan); 00126 TFT.background(Cyan) ; 00127 TFT.locate(127, 92); 00128 TFT.printf(" B "); 00129 00130 TFT.fillcircle( 260, 175, 50, Green); 00131 TFT.background(Green) ; 00132 TFT.locate(222, 160); 00133 TFT.printf(" C "); 00134 00135 TFT.fillcircle( 60, 60, 50, Orange); 00136 TFT.background(Orange) ; 00137 TFT.locate(15, 50); 00138 TFT.printf(" D "); 00139 00140 TFT.fillcircle( 260, 60, 50, GreenYellow); 00141 TFT.background(GreenYellow) ; 00142 TFT.locate(225, 50); 00143 TFT.printf(" E "); 00144 00145 TFT.BusEnable(false) ; 00146 } 00147 00148 // mapping function to change the orentation of the screen to horizontal 00149 uint16_t map(uint16_t x, uint16_t in_min, uint16_t in_max, uint16_t out_min, uint16_t out_max) 00150 { 00151 return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min; 00152 } 00153 00154 // Code used to debug screen orentation and touch pad 00155 void display_xy(void) 00156 { 00157 static uint16_t x, y, z ; 00158 uint16_t temp_x; 00159 int touched = 0; 00160 00161 while(true) { 00162 touched = TSC.getRAWPoint(&x, &y, &z); 00163 00164 x = x & 0x7f; 00165 y = y & 0x7f; 00166 00167 if(x) { // any touch 00168 wait(0.5); 00169 TFT.BusEnable(true); 00170 TFT.locate(5, 40); 00171 TFT.printf("x= %5d x=%4X", x, x); 00172 TFT.locate(5, 80); 00173 TFT.printf("y= %5d y=%4X", y, y); 00174 // TFT.locate(5, 120); 00175 // TFT.printf("raw z%6d z%4X", z, z); 00176 00177 // scale the raw values to match the screen orentation and 320(x = horiz) 240(y = vert) size 00178 // 0,0 = upper left corner 00179 temp_x = x; 00180 x = map(y,180, 3800,0,320); // TFT.set_orientation(1); 00181 y = map(temp_x,3800,280,0,240); 00182 00183 // x = map(y,3800, 180,0,320); // TFT.set_orientation(3); 00184 // y = map(temp_x,280,3800,0,240); 00185 00186 TFT.locate(5, 120); 00187 TFT.printf("map x%5d y%5d", x, y); 00188 00189 TFT.BusEnable(false); 00190 wait(0.5); 00191 } 00192 } 00193 } 00194
Generated on Wed Jul 13 2022 02:17:30 by
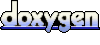