
Example Audio Spectrum Analyzer
Embed:
(wiki syntax)
Show/hide line numbers
LCD_Serial.h
00001 /* 00002 @file LCD_Serial.h 00003 @version: 1.0 00004 00005 @web www.micros-designs.com.ar 00006 @date 05/02/11 00007 00008 *- Version Log --------------------------------------------------------------* 00009 * Fecha Autor Comentarios * 00010 *----------------------------------------------------------------------------* 00011 * 05/02/11 Suky Original * 00012 *----------------------------------------------------------------------------*/ 00013 /////////////////////////////////////////////////////////////////////////// 00014 //// //// 00015 //// //// 00016 //// (C) Copyright 2011 www.micros-designs.com.ar //// 00017 //// Este codigo puede ser usado, modificado y distribuido libremente //// 00018 //// sin eliminar esta cabecera y sin garantia de ningun tipo. //// 00019 //// //// 00020 //// //// 00021 /////////////////////////////////////////////////////////////////////////// 00022 #include "mbed.h" 00023 #include "DataCGRAM.h" 00024 00025 class LCDSerial:public Stream { 00026 public: 00027 #define LCDClr 0x01 // Borra pantalla, cursor a Inicio 00028 #define LCDHome 0x02 // Cursor a Inicio, DDRAM sin cambios 00029 #define LCDIncCursor 0x06 // Modo incrementa cursor 00030 #define LCDDecCursor 0x04 // Modo decrementa cursor 00031 #define LCDOn 0x0C // Pantalla On 00032 #define LCDOff 0x08 // Pantalla Off 00033 #define LCDCursorOn 0x0E // Pantalla On, cursor On 00034 #define LCDCursorOff 0x0C // Pantalla On, cursor Off 00035 #define LCDCursorBlink 0x0F // Pantalla On, Cursor parpadeante 00036 #define LCDCursorLeft 0x10 // Mueve cursor a la izquierda 00037 #define LCDCursorRight 0x14 // Mueve cursor a la derecha 00038 #define LCDDisplayLeft 0x18 // Mueve Display a la izquierda 00039 #define LCDDisplayRight 0x1C // Mueve Display a la Derecha 00040 /** Crea LCD interface 00041 * 00042 * @param DATA 00043 * @param CLK 00044 * @param E 00045 */ 00046 LCDSerial(PinName DATA,PinName CLK,PinName E,PinName BACK=NC); 00047 void vGotoxy(char x,char y); 00048 void vSetBacklight(bool Value); 00049 void vPutc(char Data); 00050 void vCommand(char Data); 00051 #if DOXYGEN_ONLY 00052 /** Escribe un caracter en LCD 00053 * 00054 * @param c El caracter a escribir en LCD 00055 */ 00056 int putc(int c); 00057 00058 /** Escribe un string formateado en LCD 00059 * 00060 * @param format A printf-style format string, followed by the 00061 * variables to use in formating the string. 00062 */ 00063 int printf(const char* format, ...); 00064 #endif 00065 private: 00066 #define LcdType 2 // 0=5x7, 1=5x10, 2=varias lineas 00067 #define LCD_LINE_1_ADDRESS 0x00 00068 #define LCD_LINE_2_ADDRESS 0x40 00069 #define LCD_LINE_3_ADDRESS 0x14 00070 #define LCD_LINE_4_ADDRESS 0x54 00071 #define LCD_COMMAND 0 00072 #define LCD_DATA 1 00073 DigitalOut _DATA,_CLK,_E,_BACK; 00074 char NLinea; 00075 // Stream implementation functions 00076 virtual int _putc(int value); 00077 virtual int _getc(); 00078 virtual void vWriteLCD(char Data,char Type); 00079 virtual void vSetCGRAM(const char *Data); 00080 }; 00081 00082 00083 LCDSerial::LCDSerial(PinName DATA,PinName CLK,PinName E,PinName BACK) 00084 :_DATA(DATA),_CLK(CLK),_E(E),_BACK(BACK){ 00085 char Temp; 00086 00087 NLinea=1; 00088 wait_ms(15); 00089 _DATA=0; 00090 _CLK=0; 00091 _E=0; 00092 _BACK=0; 00093 00094 for(int k=0;k<8;k++){ 00095 _CLK=1; 00096 wait_us(1); 00097 _CLK=0; 00098 wait_us(1); 00099 } 00100 00101 Temp=0x03; 00102 for(int k=0;k<8;k++){ 00103 _DATA=!!(Temp&0x80); 00104 Temp<<=1; 00105 _CLK=1; 00106 wait_us(1); 00107 _CLK=0; 00108 } 00109 for(int k=0;k<3;k++){ 00110 _E=1; 00111 wait_ms(2); 00112 _E=0; 00113 wait_ms(2); 00114 } 00115 Temp=0x02; 00116 for(int k=0;k<8;k++){ 00117 _DATA=!!(Temp&0x80); 00118 Temp<<=1; 00119 _CLK=1; 00120 wait_us(1); 00121 _CLK=0; 00122 } 00123 _E=1; 00124 wait_us(1); 00125 _E=0; 00126 00127 vWriteLCD(0x20 | (LcdType<<2),LCD_COMMAND); // Tipo display.- 00128 wait_ms(2); 00129 vWriteLCD(0x01,LCD_COMMAND); // Borramos display.- 00130 wait_ms(2); 00131 vWriteLCD(0x06,LCD_COMMAND); // Incrementa cursor.- 00132 vWriteLCD(0x0C,LCD_COMMAND); // Encendemos display.- 00133 vSetCGRAM(CGRAM_DATA); // Guardamos data en CGRAM.- 00134 } 00135 00136 void LCDSerial::vWriteLCD(char Data,char Type){ 00137 char data_temp; 00138 00139 00140 wait_us(100); 00141 00142 data_temp=(Data>>4); // Rs es bit 4 00143 if(Type){ 00144 data_temp|=0x10; 00145 } 00146 for(int i=0;i<8;i++){ 00147 _DATA=!!(data_temp & 0x80); 00148 data_temp<<=1; 00149 _CLK=1; 00150 wait_us(1); 00151 _CLK=0; 00152 } 00153 00154 _E = 1; 00155 wait_us(1); 00156 _E = 0; 00157 data_temp=(Data&0x0F); // Rs es bit 4 00158 if(Type){ 00159 data_temp|=0x10; 00160 } 00161 for(int i=0;i<8;i++){ 00162 _DATA=!!(data_temp & 0x80); 00163 data_temp<<=1; 00164 _CLK=1; 00165 wait_us(1); 00166 _CLK=0; 00167 } 00168 _E = 1; 00169 wait_us(1); 00170 _E = 0; 00171 00172 } 00173 00174 void LCDSerial::vGotoxy(char x,char y){ 00175 char Direccion; 00176 00177 switch(y){ 00178 case 1:Direccion = LCD_LINE_1_ADDRESS;NLinea=1;break; 00179 case 2:Direccion = LCD_LINE_2_ADDRESS;NLinea=2;break; 00180 case 3:Direccion = LCD_LINE_3_ADDRESS;NLinea=3;break; 00181 case 4:Direccion = LCD_LINE_4_ADDRESS;NLinea=4;break; 00182 default:Direccion = LCD_LINE_1_ADDRESS;NLinea=1;break; 00183 } 00184 00185 Direccion+=x-1; 00186 vWriteLCD(0x80|Direccion,LCD_COMMAND); 00187 } 00188 00189 int LCDSerial::_getc(){ 00190 return(-1); 00191 } 00192 00193 int LCDSerial::_putc(int value){ 00194 00195 switch(value){ 00196 case '\f': 00197 vWriteLCD(0x01,LCD_COMMAND); 00198 NLinea=1; 00199 wait_ms(2); 00200 break; 00201 case '\n': 00202 vGotoxy(1,++NLinea); 00203 break; 00204 default: 00205 vWriteLCD(value,LCD_DATA); 00206 } 00207 00208 return(value); 00209 } 00210 00211 void LCDSerial::vSetBacklight(bool Value){ 00212 _BACK=Value; 00213 } 00214 00215 void LCDSerial::vSetCGRAM(const char *Data){ 00216 00217 vWriteLCD(0x40,LCD_COMMAND); 00218 for(int k=0;k<64;k++){ 00219 vWriteLCD(*Data++,LCD_DATA); 00220 } 00221 } 00222 00223 void LCDSerial::vPutc(char Data){ 00224 _putc(Data); 00225 } 00226 00227 void LCDSerial::vCommand(char Data){ 00228 vWriteLCD(Data,LCD_COMMAND); 00229 }
Generated on Wed Jul 13 2022 00:00:17 by
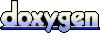