
Library set up as dummy module on mbed to mimic Nordic.
Embed:
(wiki syntax)
Show/hide line numbers
packetformatter.cpp
00001 #include <stdio.h> 00002 #include <string.h> 00003 #include "packetformatter.h" 00004 #include "charactercode.h" 00005 //#define DEBUG 00006 00007 static unsigned int checkSum; 00008 static unsigned int packetSize; 00009 static unsigned char buffer[1024]; 00010 00011 /****************************************************************************** 00012 * Function ConvertToPacket( Packet *_packet , unsigned char *_buffer ) 00013 * 00014 * This function is passed an empty packet a buffer with a message. The message 00015 * is broken down and placed into the packet. 00016 * 00017 * 00018 * PreCondition: None 00019 * 00020 * Input: '_packet' - empty packet , '_buffer' - message 00021 * 00022 * Output: 00023 * 00024 * Side Effects: None 00025 * 00026 *****************************************************************************/ 00027 int ConvertToPacket( Packet *_packet , unsigned char *_buffer ){ 00028 memset( _packet , '\0' , sizeof( Packet ) ); 00029 00030 _packet->deviceID = (int)_buffer[1] ; 00031 _packet->sourceID = (int)_buffer[2] ; 00032 _packet->command = _buffer[3]; 00033 _packet->packetLength = _buffer[4]; 00034 _packet->packetLength = _packet->packetLength << 8; 00035 _packet->packetLength |= _buffer[5]; 00036 00037 int i; 00038 for( i = 0 ; i < _packet->packetLength ; i++ ){ 00039 _packet->packetData[i] = *( _buffer + i + 6 ); 00040 } 00041 return 0; 00042 } 00043 00044 /****************************************************************************** 00045 * Function unsigned short escapedToSpecialChar(unsigned char) 00046 * 00047 * This function is called to convert a data if it is a special character (0x7F, 0x8F, 0x8E) 00048 * to equivalent escaped character. 00049 * 00050 * e.g. Special Character Escaped Character 00051 * 0x7F = 0x8E 0x01 00052 * 0x8F = 0x8E 0x02 00053 * 0x8E = 0x8E 0x8E 00054 * 00055 * PreCondition: None 00056 * 00057 * Input: 'c' - character to convert if it is an special character. 00058 * 00059 * Output: Equivalent escaped character 00060 * 00061 * Side Effects: None 00062 * 00063 *****************************************************************************/ 00064 unsigned short 00065 specialToEscapedChar(unsigned char c) 00066 { 00067 00068 unsigned short escapedChar = 0; 00069 char isSpecialChar = ((STARTPOLL == c) || (STARTPACKET == c) || (FLAGBYTE == c)); 00070 00071 if(isSpecialChar) 00072 { 00073 escapedChar = (FLAGBYTE << 8); 00074 switch(c) 00075 { 00076 case STARTPACKET: 00077 escapedChar |= 0x01; 00078 break; 00079 case STARTPOLL: 00080 escapedChar |= 0x02; 00081 break; 00082 case FLAGBYTE: 00083 escapedChar |= FLAGBYTE; 00084 break; 00085 } 00086 } 00087 else 00088 { 00089 escapedChar = (unsigned char) c; 00090 } 00091 00092 return escapedChar; 00093 } 00094 00095 /****************************************************************************** 00096 * Function static void insertValueToBuffer(unsigned char) 00097 * 00098 * This function is called to insert the value to buffer. 00099 * 00100 * PreCondition: None 00101 * 00102 * Input: 'value' - value to insert in the buffer. 00103 * 00104 * Output: None 00105 * 00106 * Side Effects: Increment the packetSize variable 00107 * 00108 *****************************************************************************/ 00109 static void 00110 insertValueToBuffer(unsigned char value) 00111 { 00112 buffer[packetSize] = value; 00113 packetSize++; 00114 } 00115 00116 /****************************************************************************** 00117 * Function static void processPacketData(unsigned char) 00118 * 00119 * This function is called to process the packet if it is a special character it will 00120 * convert to escaped character e.g. 0x7F = 0x8E 0x01, 0x8E = 0x8E 0x8E, 0x8F = 0x8E 0x02. 00121 * The size of return value of specialToEscapedChar function is 2 bytes so it must be inserted 00122 * in the buffer twice. 00123 * 00124 * PreCondition: None 00125 * 00126 * Input: 'packetData' - value to convert if it is a special character. 00127 * 00128 * Output: None 00129 * 00130 * Side Effects: None 00131 * 00132 *****************************************************************************/ 00133 static void 00134 processPacketData(unsigned char packetData) 00135 { 00136 00137 unsigned short packetDataResult = specialToEscapedChar(packetData); 00138 00139 if( (packetDataResult >> 8) != 0) 00140 { 00141 insertValueToBuffer((unsigned char)(packetDataResult>> 8)); 00142 } 00143 00144 insertValueToBuffer((unsigned char) packetDataResult); 00145 00146 } 00147 00148 /****************************************************************************** 00149 * Function void getFormattedPacket(Packet *, unsigned char *) 00150 * 00151 * This function is called to process the packet in to a series of bytes that 00152 * conforms into the protocol of the communication. 00153 * Protocol Format Example: 00154 * 0x7F - Start Packet 00155 * 0xFE - Device ID 00156 * 0x01 - Source ID 00157 * 0x05 - Command type 00158 * 0x00 - Payload length 1st byte 00159 * 0x01 - Payload length 2nd byte 00160 * 0x04 - Payload or data 00161 * 0x76 - checksum 00162 * 00163 * 00164 * PreCondition: None 00165 * 00166 * Input: 'packet' - instance of Packet data structure that contains the 00167 * following information: 00168 * + device id 00169 * + source id 00170 * + command 00171 * + packet or payload length 00172 * + packet or payload 00173 * 00174 * 'packetBuffer' - empty buffer that will be the holder of the process data or packet. 00175 * 00176 * Output: Total size of data inserted in the buffer. 00177 * 00178 * Side Effects: None 00179 * 00180 *****************************************************************************/ 00181 int getFormattedPacket(Packet * packet, unsigned char * packetBuffer) 00182 { 00183 00184 checkSum = 0; 00185 packetSize = 0; 00186 00187 insertValueToBuffer(STARTPACKET); 00188 checkSum += STARTPACKET; 00189 00190 #ifdef DEBUG 00191 printf("Start Packet: %x\n", STARTPACKET); 00192 #endif 00193 00194 processPacketData(packet->deviceID); 00195 checkSum += packet->deviceID; 00196 00197 #ifdef DEBUG 00198 printf("Device ID: %x\n", packet->deviceID); 00199 #endif 00200 00201 processPacketData(packet->sourceID); 00202 checkSum += packet->sourceID; 00203 00204 #ifdef DEBUG 00205 printf("Source ID: %x\n", packet->sourceID); 00206 #endif 00207 00208 processPacketData(packet->command); 00209 checkSum += packet->command; 00210 00211 #ifdef DEBUG 00212 printf("Command: %x\n", packet->command); 00213 #endif 00214 00215 unsigned char mostSignificantBytePacketLength = packet->packetLength >> 8; 00216 unsigned char leastSignificantBytePacketLength = (unsigned char) packet->packetLength; 00217 processPacketData(mostSignificantBytePacketLength); 00218 checkSum += mostSignificantBytePacketLength; 00219 processPacketData(leastSignificantBytePacketLength); 00220 checkSum += leastSignificantBytePacketLength; 00221 00222 #ifdef DEBUG 00223 printf("Packet length MSB: %x\n", mostSignificantBytePacketLength); 00224 printf("Packet length LSB: %x\n", leastSignificantBytePacketLength); 00225 #endif 00226 00227 int i; 00228 for(i = 0; i < packet->packetLength; i++) 00229 { 00230 processPacketData(packet->packetData[i]); 00231 checkSum += packet->packetData[i]; 00232 00233 #ifdef DEBUG 00234 printf("Data %d: %x\n", i, packet->packetData[i]); 00235 #endif 00236 } 00237 00238 unsigned char checksumLSB = (unsigned char)(checkSum & 0xFF); 00239 unsigned char twosComplementCheckSum = ~checksumLSB + 1; 00240 processPacketData(twosComplementCheckSum); 00241 00242 #ifdef DEBUG 00243 printf("Checksum: %x\n", twosComplementCheckSum); 00244 #endif 00245 00246 memcpy(packetBuffer, buffer, packetSize); 00247 00248 return packetSize; 00249 } 00250 00251 /****************************************************************************** 00252 * Function void getFormattedBootloaderPacket(unsigned char *, unsigned char *, int) 00253 * 00254 * This function is called to process the data in to a series of bytes that 00255 * conforms into the bootloader protocol. 00256 * Protocol Format Example: 00257 * 0x55 - STX or Start of TeXt 00258 * 0x55 - STX or Start of TeXt 00259 * 0x01 - Data 00260 * 0x03 - Data 00261 * 0x0C - Checksum 00262 * 0x04 - ETX or End of TeXt 00263 * 00264 * 00265 * PreCondition: None 00266 * 00267 * Input: 00268 * 'buffer' - empty buffer that will be the holder of the process. 00269 * 'data' - values to be send in bootloader. 00270 * 'size' - size of data to be send. 00271 * 00272 * Output: Total size of data inserted in the buffer. 00273 * 00274 * Side Effects: None 00275 * 00276 *****************************************************************************/ 00277 int 00278 getFormattedBootloaderPacket(unsigned char *buffer, unsigned char *data, int size) 00279 { 00280 int i; 00281 int j; 00282 int sum; 00283 00284 i = 0; 00285 buffer[i++] = STX; 00286 buffer[i++] = STX; 00287 sum = 0; 00288 00289 for(j = 0; j < size; j++) 00290 { 00291 if(data[j] == ETX || data[j] == STX || data[j] == ENQ) 00292 { 00293 buffer[j+i++] = ENQ; 00294 } 00295 00296 buffer[j+i] = data[j]; 00297 sum += data[j]; 00298 } 00299 00300 sum = ((sum * -1) & 0xFF); 00301 if (sum == ETX || sum == STX || sum == ENQ) 00302 buffer[j+i++] = ENQ; 00303 00304 buffer[j+i] = sum; 00305 j++; 00306 buffer[j+i] = ETX; 00307 j++; 00308 00309 //printf("getFormattedBootloaderPacket return = %d\n",j+i); 00310 00311 return (j+i); 00312 } 00313 00314
Generated on Tue Jul 12 2022 22:58:49 by
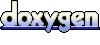