
Library set up as dummy module on mbed to mimic Nordic.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //#define TEST 00002 #define DEBUG 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "comms.h" 00007 #include "rs485.h" 00008 #include "uart1.h" 00009 #include "BTpacket.h" 00010 00011 extern const unsigned char DEVADDRESS = 0xF0; 00012 #define NORDIC_ADDRESS DEVADDRESS 00013 #define BTMODULE_A_ADDRESS 0x20 // #CC not sure if this has been defined by CEPD 00014 #define BTMODULE_B_ADDRESS 0x22 // #CC not sure if this has been defined by CEPD 00015 00016 enum Commands { FX1 = 0x10 , FX1_ACK = 0x90 , // #CC list the commands needed for the NORDIC Module 00017 FX2 = 0x12 , FX2_ACK = 0x92 00018 }; 00019 00020 /*=========================================== 00021 * These functions need to be completed 00022 * 00023 */ 00024 int Bluetooth_ReceivePacket( Packet * ); 00025 int Bluetooth_SendChar( unsigned char ); 00026 int Bluetooth_SerialHandler( void ); 00027 /*==========================================*/ 00028 00029 void Fx1( unsigned char * ); 00030 void Fx2( unsigned char * ); 00031 00032 00033 /*=========================================== 00034 * 00035 * 00036 */ 00037 void receivedSerialData( void ){ 00038 while( uart1_is_char() ){ 00039 SerialHandler( uart1_get_char() ); 00040 } 00041 } 00042 00043 void bus_thread(void const *argument){ 00044 while (true){ 00045 receivedSerialData(); 00046 wait_ms( 100 ); 00047 } 00048 } 00049 00050 void Test( void ); 00051 void PrintPacket( Packet *_packet ); 00052 /*==========================================*/ 00053 00054 int main() { 00055 00056 RegisterCommand( FX1 , &Fx1 ); 00057 RegisterCommand( FX2 , &Fx2 ); 00058 00059 initComms(); 00060 pc.printf( "\n\rStarted...\n\r" ); 00061 init_uart1(); 00062 00063 Thread bus( bus_thread , NULL , osPriorityRealtime , DEFAULT_STACK_SIZE ); 00064 00065 while( true ){ }; 00066 00067 #ifdef TEST 00068 Test(); 00069 #endif // DEBUG 00070 00071 00072 } 00073 00074 //================ Custom Functions ============================================= 00075 /* These functions local functions to the Nordic chip that are exposed to the 00076 * system. They must follow this prototype (same return values and parameter) 00077 * and must be registered with a command using RegisterCommand() from RS485 00078 * library. 00079 */ 00080 void Fx1( unsigned char *_receivedData ){ 00081 00082 pc.printf( "In FX1!\n\r" ); 00083 00084 Packet packet; 00085 packet.sourceID = 0xF0; 00086 packet.deviceID = 0xFE; 00087 packet.command = 0x90; 00088 packet.packetData[0] = 0x22; 00089 packet.packetLength = 0x01; 00090 00091 SetResponsePacket( &packet ); 00092 } 00093 00094 void Fx2( unsigned char *_receivedData ){ 00095 00096 pc.printf( "In FX2!\n\r" ); 00097 00098 Packet packet; 00099 packet.sourceID = 0xF0; 00100 packet.deviceID = 0xFE; 00101 packet.command = 0x90; 00102 packet.packetData[0] = 0x22; 00103 packet.packetLength = 0x01; 00104 00105 SetResponsePacket( &packet ); 00106 } 00107 //================ End Custom Functions ========================================= 00108 00109 //#CC This needs to handle the uart port and pass received bytes on 00110 /****************************************************************************** 00111 * Bluetooth_SerialHandler 00112 * 00113 * The UART port needs to be handled and when a byte is received it is passed on 00114 * to SerialHandler() form the rs485 library. 00115 * 00116 * PreCondition: None 00117 * 00118 * Input: '_packet' - packet received from the bus. 00119 * 00120 * 00121 * Output: None 00122 * 00123 * Side Effects: None 00124 * 00125 *****************************************************************************/ 00126 int Bluetooth_SerialHandler( void ){ 00127 // if( ByteAvailableOnSerial ){ 00128 // SerialHandler( GetByte() ); 00129 // } 00130 return 0; 00131 } 00132 00133 //#CC This needs to be modified as commented below 00134 /****************************************************************************** 00135 * Bluetooth_ReceivePacket 00136 * 00137 * This gets automatically called by the bus library when a complete packet is 00138 * received. It checks the address the packet is going to and redirects the 00139 * message appropriately (ie: if message is for the Nordic chip it calls a 00140 * function or it sends it off to a BT device). 00141 * 00142 * PreCondition: None 00143 * 00144 * Input: '_packet' - packet received from the bus. 00145 * 00146 * 00147 * Output: None 00148 * 00149 * Side Effects: None 00150 * 00151 *****************************************************************************/ 00152 int Bluetooth_ReceivePacket( Packet *_packet ){ 00153 00154 #ifdef DEBUG 00155 PrintPacket( _packet ); 00156 #endif // DEBUG 00157 00158 switch ( _packet->command ){ // makes decision based off where packet is addressed to go 00159 00160 case NORDIC_ADDRESS: // if the message is for the Nordic chip... 00161 if( !CheckFunction( _packet ) ){ // this will call the appropriate function if one is registered 00162 //#CC // code goes here if the function is not registered 00163 } 00164 break; 00165 00166 case BTMODULE_A_ADDRESS: // if message is for Bluetooth Module A 00167 //#CC 00168 /* 00169 Need to bring in packet and modify to send out over BT 00170 */ 00171 break; 00172 00173 case BTMODULE_B_ADDRESS: 00174 00175 break; 00176 00177 default: 00178 break; 00179 00180 } 00181 00182 return 0; 00183 } 00184 00185 int Bluetooth_SendChar( unsigned char _c ){ 00186 bus.putc( _c ); 00187 return 0; 00188 } 00189 00190 void PrintPacket( Packet *_packet ){ 00191 pc.printf( "Process Packet\n\r" ); 00192 00193 pc.printf( "\t%x - Device ID\n\r" , _packet->deviceID ); 00194 pc.printf( "\t%x - Source ID\n\r" , _packet->sourceID ); 00195 pc.printf( "\t%x - Command\n\r" , _packet->command ); 00196 pc.printf( "\t%x - Length\n\r" , _packet->packetLength ); 00197 unsigned char *ptr = (unsigned char *)&_packet->packetData; 00198 for( int i = 0 ; i < _packet->packetLength ; i++ ){ 00199 pc.printf( "\t%x - Data[%d]\n\r" , *ptr++ , i ); 00200 } 00201 } 00202 00203 void Test( void ){ 00204 00205 Packet commandPacket; 00206 commandPacket.sourceID = 0xFE; 00207 commandPacket.deviceID = 0xF0; 00208 commandPacket.command = 0x10; 00209 commandPacket.packetLength = 0x04; 00210 commandPacket.packetData[0] = 0x8E; 00211 commandPacket.packetData[1] = 0x7F; 00212 commandPacket.packetData[2] = 0x8F; 00213 commandPacket.packetData[3] = 0xB0; 00214 00215 unsigned char buf1[1024]; 00216 unsigned char buf2[1024]; 00217 unsigned char *ptr = buf1; 00218 00219 // buf for commandPacket 00220 int size1 = getFormattedPacket( &commandPacket , buf1 ); 00221 int size2; 00222 00223 // buf for POLL 00224 buf1[0] = 0x8F; 00225 buf1[1] = 0xF0; 00226 size1 = 2; 00227 00228 buf2[0] = 0x8D; 00229 size2 = 1; 00230 00231 pc.printf( "size: %i\t" , size1 ); 00232 00233 pc.printf( "buf: " ); 00234 for( int i = 0 ; i < size1 ; i++ ){ 00235 pc.printf( "%x " , *(ptr++) ); 00236 } 00237 pc.printf( " \n\r" ); 00238 00239 while(1) { 00240 // poll with no message 00241 ptr = buf1; 00242 for( int i = 0 ; i < size1 ; i++ ){ 00243 SerialHandler( *(ptr++) ); 00244 wait( 1 ); 00245 } 00246 00247 // create message 00248 Bluetooth_SendChar( 0xF0 ); 00249 Bluetooth_SendChar( 0x01 ); 00250 Bluetooth_SendChar( 0x10 ); 00251 00252 // poll with message 00253 ptr = buf1; 00254 for( int i = 0 ; i < size1 ; i++ ){ 00255 SerialHandler( *(ptr++) ); 00256 wait( 1 ); 00257 } 00258 00259 // send ACK 00260 ptr = buf2; 00261 for( int i = 0 ; i < size2 ; i++ ){ 00262 SerialHandler( *(ptr++) ); 00263 wait( 1 ); 00264 } 00265 } 00266 }
Generated on Tue Jul 12 2022 22:58:49 by
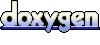