Interrupt based I2C functionality for the LPC1768. (If you want the other one, send a PM and I might make it). Also adds buffer functionality to the I2C bus.
Dependents: Algoritmo_Fuzzy FlyBed1 FlyBedLight
MODI2C Class Reference
Library that allows interrupt driven communication with I2C devices. More...
#include <MODI2C.h>
Public Member Functions | |
MODI2C (PinName sda, PinName scl) | |
Constructor. | |
int | write (int address, char *data, int length, bool repeated=false, int *status=NULL) |
Write data on the I2C bus. | |
int | read_nb (int address, char *data, int length, bool repeated=false, int *status=NULL) |
Read data non-blocking from the I2C bus. | |
int | read (int address, char *data, int length, bool repeated=false) |
Read data from the I2C bus. | |
void | frequency (int hz) |
Sets the I2C bus frequency. | |
void | start (void) |
Creates a start condition on the I2C bus. | |
void | stop (void) |
Creates a stop condition on the I2C bus. | |
void | detach (void) |
Removes attached function. | |
void | attach (void(*function)(void), int operation=IRQ_I2C_BOTH) |
Calls user function when I2C command is finished. | |
template<typename T > | |
void | attach (T *object, void(T::*member)(void), int operation=IRQ_I2C_BOTH) |
Calls user function when I2C command is finished. | |
int | getQueue (void) |
Returns the current number of commands in the queue (including one currently being processed) |
Detailed Description
Library that allows interrupt driven communication with I2C devices.
For now this is all in beta, so if you got weird results while the mbed I2C library works, it is probably my fault. Similar to googles definition of beta, it is unlikely it will ever come out of beta.
Example:
#include "mbed.h" #include "MODI2C.h" #include "MPU6050.h" Serial pc(USBTX, USBRX); // tx, rx MODI2C mod(p9, p10); int main() { char registerAdd = MPU6050_WHO_AM_I_REG; char registerResult; int status; while (1) { mod.write(MPU6050_ADDRESS*2, ®isterAdd, 1, true); mod.read_nb(MPU6050_ADDRESS*2, ®isterResult, 1, &status); while (!status) wait_us(1); pc.printf("Register holds 0x%02X\n\r", registerResult); wait(2); } }
Definition at line 77 of file MODI2C.h.
Constructor & Destructor Documentation
MODI2C | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor.
- Parameters:
-
sda - mbed pin to use for the SDA I2C line. scl - mbed pin to use for the SCL I2C line.
Definition at line 11 of file MODI2C.cpp.
Member Function Documentation
void attach | ( | void(*)(void) | function, |
int | operation = IRQ_I2C_BOTH |
||
) |
Calls user function when I2C command is finished.
- Parameters:
-
function - the function to call. operation - when to call IRQ: IRQ_I2C_BOTH (default) - IRQ_I2C_READ - IRQ_I2C_WRITE
Definition at line 164 of file MODI2C.cpp.
void attach | ( | T * | object, |
void(T::*)(void) | member, | ||
int | operation = IRQ_I2C_BOTH |
||
) |
Calls user function when I2C command is finished.
- Parameters:
-
object - the object to call the function on. member - the function to call operation - when to call IRQ: IRQ_I2C_BOTH (default) - IRQ_I2C_READ - IRQ_I2C_WRITE
Definition at line 170 of file MODI2C.cpp.
void detach | ( | void | ) |
Removes attached function.
Definition at line 175 of file MODI2C.cpp.
void frequency | ( | int | hz ) |
Sets the I2C bus frequency.
- Parameters:
-
hz - the bus frequency in herz
Definition at line 114 of file MODI2C.cpp.
int getQueue | ( | void | ) |
Returns the current number of commands in the queue (including one currently being processed)
Note that this is the number of commands, not the number of bytes
- Parameters:
-
return - number of commands in queue
Definition at line 124 of file MODI2C.cpp.
int read | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false |
||
) |
Read data from the I2C bus.
This function should should be a drop-in replacement for the standard mbed function.
- Parameters:
-
address - I2C address of the slave (7 bit address << 1). data - pointer to byte array where the data will be stored length - amount of bytes that need to be received repeated - determines if it should end with a stop condition return - returns zero on success, LPC status code on failure
Definition at line 71 of file MODI2C.cpp.
int read_nb | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false , |
||
int * | status = NULL |
||
) |
Read data non-blocking from the I2C bus.
Reads data from the I2C bus, completely non-blocking. Aditionally it will always return zero, since it does not wait to see how the transfer goes. A pointer to an integer can be added as argument which returns the status.
- Parameters:
-
address - I2C address of the slave (7 bit address << 1). data - pointer to byte array where the data will be stored length - amount of bytes that need to be received repeated - determines if it should end with a stop condition status - (optional) pointer to integer where the final status code of the I2C transmission is placed. (0x58 is success) return - returns zero
Definition at line 47 of file MODI2C.cpp.
void start | ( | void | ) |
Creates a start condition on the I2C bus.
If you use this function you probably break something (but mbed also had it public)
Definition at line 106 of file MODI2C.cpp.
void stop | ( | void | ) |
Creates a stop condition on the I2C bus.
If you use this function you probably break something (but mbed also had it public)
Definition at line 110 of file MODI2C.cpp.
int write | ( | int | address, |
char * | data, | ||
int | length, | ||
bool | repeated = false , |
||
int * | status = NULL |
||
) |
Write data on the I2C bus.
This function should generally be a drop-in replacement for the standard mbed write function. However this function is completely non-blocking, so it barely takes time. This can cause different behavior. Everything else is similar to read_nb.
- Parameters:
-
address - I2C address of the slave (7 bit address << 1). data - pointer to byte array that holds the data length - amount of bytes that need to be sent repeated - determines if it should end with a stop condition (default false) status - (optional) pointer to integer where the final status code of the I2C transmission is placed. (0x28 is success) return - returns zero
Definition at line 26 of file MODI2C.cpp.
Generated on Sat Jul 16 2022 11:45:43 by
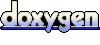