Library for the MMA7660 triple axis accelerometer
Dependents: Websocket_Ethernet_acc app-board-Sprint-WS-Acc app-board-Ethernet-Websocket app-board-Wifly-Websocket ... more
MMA7660.h
00001 /* Copyright (c) <year> <copyright holders>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 00021 00022 #ifndef MMA7660_H 00023 #define MMA7660_H 00024 00025 #define MMA7660_ADDRESS 0x98 00026 #define MMA7660_SENSITIVITY 21.33 00027 00028 #define MMA7660_XOUT_R 0x00 00029 #define MMA7660_YOUT_R 0x01 00030 #define MMA7660_ZOUT_R 0x02 00031 #define MMA7660_TILT_R 0x03 00032 #define MMA7660_INT_R 0x06 00033 #define MMA7660_MODE_R 0x07 00034 #define MMA7660_SR_R 0x08 00035 00036 00037 /** An interface for the MMA7660 triple axis accelerometer 00038 * 00039 * @code 00040 * //Uses the measured z-acceleration to drive leds 2 and 3 of the mbed 00041 * 00042 * #include "mbed.h" 00043 * #include "MMA7660.h" 00044 * 00045 * MMA7660 MMA(p28, p27); 00046 * 00047 * DigitalOut connectionLed(LED1); 00048 * PwmOut Zaxis_p(LED2); 00049 * PwmOut Zaxis_n(LED3); 00050 * 00051 * int main() { 00052 * if (MMA.testConnection()) 00053 * connectionLed = 1; 00054 * 00055 * while(1) { 00056 * Zaxis_p = MMA.z(); 00057 * Zaxis_n = -MMA.z(); 00058 * } 00059 * 00060 * } 00061 * @endcode 00062 */ 00063 class MMA7660 00064 { 00065 public: 00066 /** 00067 * The 6 different orientations and unknown 00068 * 00069 * Up & Down = X-axis 00070 * Right & Left = Y-axis 00071 * Back & Front = Z-axis 00072 * 00073 */ 00074 enum Orientation {Up, Down, 00075 Right, Left, 00076 Back, Front, 00077 Unknown 00078 }; 00079 00080 /** 00081 * Creates a new MMA7660 object 00082 * 00083 * @param sda - I2C data pin 00084 * @param scl - I2C clock pin 00085 * @param active - true (default) to enable the device, false to keep it standby 00086 */ 00087 MMA7660(PinName sda, PinName scl, bool active = true); 00088 00089 /** 00090 * Tests if communication is possible with the MMA7660 00091 * 00092 * Because the MMA7660 lacks a WHO_AM_I register, this function can only check 00093 * if there is an I2C device that responds to the MMA7660 address 00094 * 00095 * @param return - true for successfull connection, false for no connection 00096 */ 00097 bool testConnection( void ); 00098 00099 /** 00100 * Sets the active state of the MMA7660 00101 * 00102 * Note: This is unrelated to awake/sleep mode 00103 * 00104 * @param state - true for active, false for standby 00105 */ 00106 void setActive( bool state); 00107 00108 /** 00109 * Reads acceleration data from the sensor 00110 * 00111 * When the parameter is a pointer to an integer array it will be the raw data. 00112 * When it is a pointer to a float array it will be the acceleration in g's 00113 * 00114 * @param data - pointer to array with length 3 where the acceleration data will be stored, X-Y-Z 00115 */ 00116 void readData( int *data); 00117 void readData( float *data); 00118 00119 /** 00120 * Get X-data 00121 * 00122 * @param return - X-acceleration in g's 00123 */ 00124 float x( void ); 00125 00126 /** 00127 * Get Y-data 00128 * 00129 * @param return - Y-acceleration in g's 00130 */ 00131 float y( void ); 00132 00133 /** 00134 * Get Z-data 00135 * 00136 * @param return - Z-acceleration in g's 00137 */ 00138 float z( void ); 00139 00140 /** 00141 * Sets the active samplerate 00142 * 00143 * The entered samplerate will be rounded to nearest supported samplerate. 00144 * Supported samplerates are: 120 - 64 - 32 - 16 - 8 - 4 - 2 - 1 samples/second. 00145 * 00146 * @param samplerate - the samplerate that will be set 00147 */ 00148 void setSampleRate(int samplerate); 00149 00150 /** 00151 * Returns if it is on its front, back, or unknown side 00152 * 00153 * This is read from MMA7760s registers, page 12 of datasheet 00154 * 00155 * @param return - Front, Back or Unknown orientation 00156 */ 00157 Orientation getSide( void ); 00158 00159 /** 00160 * Returns if it is on it left, right, down or up side 00161 * 00162 * This is read from MMA7760s registers, page 12 of datasheet 00163 * 00164 * @param return - Left, Right, Down, Up or Unknown orientation 00165 */ 00166 Orientation getOrientation ( void ); 00167 00168 00169 private: 00170 00171 /** 00172 * Writes data to the device 00173 * 00174 * @param adress - register address to write to 00175 * @param data - data to write 00176 */ 00177 void write( char address, char data); 00178 00179 /** 00180 * Read data from the device 00181 * 00182 * @param adress - register address to write to 00183 * @return - data from the register specified by RA 00184 */ 00185 char read( char adress); 00186 00187 /** 00188 * Read multiple regigsters from the device, more efficient than using multiple normal reads. 00189 * 00190 * @param adress - register address to write to 00191 * @param length - number of bytes to read 00192 * @param data - pointer where the data needs to be written to 00193 */ 00194 void read( char adress, char *data, int length); 00195 00196 /** 00197 * Reads single axis 00198 */ 00199 float getSingle(int number); 00200 00201 I2C _i2c; 00202 bool active; 00203 float samplerate; 00204 }; 00205 00206 00207 #endif
Generated on Tue Jul 12 2022 21:12:30 by
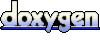