
InterruptIn speed check
Fork of InterruptIn_speed by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //This is a mercury test 00002 00003 #include "mbed.h" 00004 #include "FastPWM.h" 00005 00006 FastPWM pwm(PTA13); 00007 InterruptIn interrupt(PTD5); 00008 00009 volatile int count; 00010 00011 void interrupt_handler(void) 00012 { 00013 count++; 00014 } 00015 00016 00017 int main (void) 00018 { 00019 //Start_frequency is 60kHz 00020 double frequency = 65e3; 00021 pwm = 0; 00022 interrupt.rise(&interrupt_handler); 00023 00024 while(1) { 00025 //Set PWM period, reset count 00026 pwm.period(1/frequency); 00027 count = 0; 00028 00029 //Enable PWM at 50% dutycycle for 1 second 00030 pwm = 0.5; 00031 wait(1); 00032 pwm = 0; 00033 00034 printf("Expected %.0f edges, measured %d edges.\n", frequency, count); 00035 if (count < frequency * 0.9) { 00036 printf("Test failed at %.0fkHz!\n\n", frequency / 1000); 00037 break; 00038 } 00039 printf("Test succeeded at %.0fkHz!\n\n", frequency / 1000); 00040 frequency += 10e3; 00041 00042 } 00043 while(1); 00044 } 00045
Generated on Thu Jul 14 2022 02:14:31 by
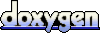