fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
mbed_interface.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <stdio.h> 00017 #include "mbed_interface.h" 00018 00019 #include "gpio_api.h" 00020 #include "wait_api.h" 00021 #include "semihost_api.h" 00022 #include "error.h" 00023 #include "toolchain.h" 00024 00025 #if DEVICE_SEMIHOST 00026 00027 // return true if a debugger is attached, indicating mbed interface is connected 00028 int mbed_interface_connected(void) { 00029 return semihost_connected(); 00030 } 00031 00032 int mbed_interface_reset(void) { 00033 if (mbed_interface_connected()) { 00034 semihost_reset(); 00035 return 0; 00036 } else { 00037 return -1; 00038 } 00039 } 00040 00041 WEAK int mbed_interface_uid(char *uid); 00042 WEAK int mbed_interface_uid(char *uid) { 00043 if (mbed_interface_connected()) { 00044 return semihost_uid(uid); // Returns 0 if successful, -1 on failure 00045 } else { 00046 uid[0] = 0; 00047 return -1; 00048 } 00049 } 00050 00051 int mbed_interface_disconnect(void) { 00052 if (mbed_interface_connected()) { 00053 return semihost_disabledebug(); 00054 } else { 00055 return -1; 00056 } 00057 } 00058 00059 int mbed_interface_powerdown(void) { 00060 if (mbed_interface_connected()) { 00061 return semihost_powerdown(); 00062 } else { 00063 return -1; 00064 } 00065 } 00066 00067 // for backward compatibility 00068 void mbed_reset(void) { 00069 mbed_interface_reset(); 00070 } 00071 00072 WEAK int mbed_uid(char *uid); 00073 WEAK int mbed_uid(char *uid) { 00074 return mbed_interface_uid(uid); 00075 } 00076 #endif 00077 00078 WEAK void mbed_mac_address(char *mac); 00079 WEAK void mbed_mac_address(char *mac) { 00080 #if DEVICE_SEMIHOST 00081 char uid[DEVICE_ID_LENGTH + 1]; 00082 int i; 00083 00084 // if we have a UID, extract the MAC 00085 if (mbed_interface_uid(uid) == 0) { 00086 char *p = uid; 00087 #if defined(DEVICE_MAC_OFFSET) 00088 p += DEVICE_MAC_OFFSET; 00089 #endif 00090 for (i=0; i<6; i++) { 00091 int byte; 00092 sscanf(p, "%2x", &byte); 00093 mac[i] = byte; 00094 p += 2; 00095 } 00096 } else { // else return a default MAC 00097 #endif 00098 mac[0] = 0x00; 00099 mac[1] = 0x02; 00100 mac[2] = 0xF7; 00101 mac[3] = 0xF0; 00102 mac[4] = 0x00; 00103 mac[5] = 0x00; 00104 #if DEVICE_SEMIHOST 00105 } 00106 #endif 00107 }
Generated on Tue Jul 12 2022 13:47:01 by
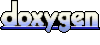