fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
us_ticker.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <stddef.h> 00017 #include "us_ticker_api.h" 00018 #include "PeripheralNames.h" 00019 00020 #define US_TICKER_TIMER_IRQn SCT_IRQn 00021 00022 int us_ticker_inited = 0; 00023 00024 void us_ticker_init(void) { 00025 if (us_ticker_inited) return; 00026 us_ticker_inited = 1; 00027 00028 // Enable the SCT clock 00029 LPC_SYSCON->SYSAHBCLKCTRL |= (1 << 8); 00030 00031 // Clear peripheral reset the SCT: 00032 LPC_SYSCON->PRESETCTRL |= (1 << 8); 00033 00034 // Unified counter (32 bits) 00035 LPC_SCT->CONFIG |= 1; 00036 00037 // halt and clear the counter 00038 LPC_SCT->CTRL_L |= (1 << 2) | (1 << 3); 00039 00040 // System Clock (12)MHz -> us_ticker (1)MHz 00041 LPC_SCT->CTRL_L |= ((SystemCoreClock/1000000 - 1) << 5); 00042 00043 // unhalt the counter: 00044 // - clearing bit 2 of the CTRL register 00045 LPC_SCT->CTRL_L &= ~(1 << 2); 00046 00047 NVIC_SetVector(US_TICKER_TIMER_IRQn, (uint32_t)us_ticker_irq_handler); 00048 NVIC_EnableIRQ(US_TICKER_TIMER_IRQn); 00049 } 00050 00051 uint32_t us_ticker_read() { 00052 if (!us_ticker_inited) 00053 us_ticker_init(); 00054 00055 return LPC_SCT->COUNT_U; 00056 } 00057 00058 void us_ticker_set_interrupt(unsigned int timestamp) { 00059 // halt the counter: 00060 // - setting bit 2 of the CTRL register 00061 LPC_SCT->CTRL_L |= (1 << 2); 00062 00063 // set timestamp in compare register 00064 LPC_SCT->MATCH[0].U = timestamp; 00065 00066 // unhalt the counter: 00067 // - clearing bit 2 of the CTRL register 00068 LPC_SCT->CTRL_L &= ~(1 << 2); 00069 00070 // if events are not enabled, enable them 00071 if (!(LPC_SCT->EVEN & 0x01)) { 00072 00073 // comb mode = match only 00074 LPC_SCT->EVENT[0].CTRL = (1 << 12); 00075 00076 // ref manual: 00077 // In simple applications that do not 00078 // use states, write 0x01 to this 00079 // register to enable an event 00080 LPC_SCT->EVENT[0].STATE |= 0x1; 00081 00082 // enable events 00083 LPC_SCT->EVEN |= 0x1; 00084 } 00085 } 00086 00087 void us_ticker_disable_interrupt(void) { 00088 LPC_SCT->EVEN &= ~1; 00089 } 00090 00091 void us_ticker_clear_interrupt(void) { 00092 LPC_SCT->EVFLAG = 1; 00093 }
Generated on Tue Jul 12 2022 13:47:02 by
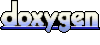