fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
analogout_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "analogout_api.h" 00017 00018 #include "cmsis.h" 00019 #include "pinmap.h" 00020 #include "error.h" 00021 00022 static const PinMap PinMap_DAC[] = { 00023 {P0_26, DAC_0, 2}, 00024 {NC , NC , 0} 00025 }; 00026 00027 void analogout_init(dac_t *obj, PinName pin) { 00028 obj->dac = (DACName)pinmap_peripheral(pin, PinMap_DAC); 00029 if (obj->dac == (uint32_t)NC) { 00030 error("DAC pin mapping failed"); 00031 } 00032 00033 // power is on by default, set DAC clk divider is /4 00034 LPC_SC->PCLKSEL0 &= ~(0x3 << 22); 00035 00036 // map out (must be done before accessing registers) 00037 pinmap_pinout(pin, PinMap_DAC); 00038 00039 analogout_write_u16(obj, 0); 00040 } 00041 00042 void analogout_free(dac_t *obj) {} 00043 00044 static inline void dac_write(int value) { 00045 value &= 0x3FF; // 10-bit 00046 00047 // Set the DAC output 00048 LPC_DAC->DACR = (0 << 16) // bias = 0 00049 | (value << 6); 00050 } 00051 00052 static inline int dac_read() { 00053 return (LPC_DAC->DACR >> 6) & 0x3FF; 00054 } 00055 00056 void analogout_write(dac_t *obj, float value) { 00057 if (value < 0.0f) { 00058 dac_write(0); 00059 } else if (value > 1.0f) { 00060 dac_write(0x3FF); 00061 } else { 00062 dac_write(value * (float)0x3FF); 00063 } 00064 } 00065 00066 void analogout_write_u16(dac_t *obj, uint16_t value) { 00067 dac_write(value >> 6); // 10-bit 00068 } 00069 00070 float analogout_read(dac_t *obj) { 00071 uint32_t value = dac_read(); 00072 return (float)value * (1.0f / (float)0x3FF); 00073 } 00074 00075 uint16_t analogout_read_u16(dac_t *obj) { 00076 uint32_t value = dac_read(); // 10-bit 00077 return (value << 6) | ((value >> 4) & 0x003F); 00078 }
Generated on Tue Jul 12 2022 13:47:00 by
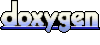