fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
cmsis_nvic.c
00001 /* mbed Microcontroller Library - cmsis_nvic for LCP1768 00002 * Copyright (c) 2009-2011 ARM Limited. All rights reserved. 00003 * 00004 * CMSIS-style functionality to support dynamic vectors 00005 */ 00006 #include "cmsis_nvic.h" 00007 00008 #define NVIC_NUM_VECTORS (16 + 33) // CORE + MCU Peripherals 00009 #define NVIC_RAM_VECTOR_ADDRESS (0x10000000) // Location of vectors in RAM 00010 00011 void NVIC_SetVector(IRQn_Type IRQn, uint32_t vector) { 00012 static volatile uint32_t* vectors = (uint32_t*)NVIC_RAM_VECTOR_ADDRESS; 00013 int i; 00014 // Copy and switch to dynamic vectors if first time called 00015 if (SCB->VTOR != NVIC_RAM_VECTOR_ADDRESS) { 00016 uint32_t *old_vectors = (uint32_t*)SCB->VTOR; 00017 for (i=0; i<NVIC_NUM_VECTORS; i++) { 00018 vectors[i] = old_vectors[i]; 00019 } 00020 SCB->VTOR = (uint32_t)vectors; 00021 } 00022 00023 vectors[IRQn + 16] = vector; 00024 } 00025 00026 uint32_t NVIC_GetVector(IRQn_Type IRQn) { 00027 uint32_t *vectors = (uint32_t*)SCB->VTOR; 00028 return vectors[IRQn + 16]; 00029 } 00030
Generated on Tue Jul 12 2022 13:47:00 by
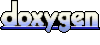