fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
sys.cpp
00001 #include "cmsis.h" 00002 #include <sys/types.h> 00003 #include <errno.h> 00004 00005 extern "C" { 00006 00007 struct SCS3Regions { 00008 unsigned long Dummy; 00009 unsigned long* InitRam; 00010 unsigned long* StartRam; 00011 unsigned long InitSizeRam; 00012 unsigned long ZeroSizeRam; 00013 }; 00014 00015 extern unsigned long __cs3_regions; 00016 extern unsigned long __cs3_heap_start; 00017 00018 int main(void); 00019 void __libc_init_array(void); 00020 void exit(int ErrorCode); 00021 00022 static void *heap_pointer = NULL; 00023 00024 void __cs3_start_c(void) { 00025 static SCS3Regions* pCS3Regions = (SCS3Regions*)&__cs3_regions; 00026 unsigned long* pulDest; 00027 unsigned long* pulSrc; 00028 unsigned long ByteCount; 00029 unsigned long i; 00030 00031 pulSrc = pCS3Regions->InitRam; 00032 pulDest = pCS3Regions->StartRam; 00033 ByteCount = pCS3Regions->InitSizeRam; 00034 if (pulSrc != pulDest) { 00035 for(i = 0 ; i < ByteCount ; i += sizeof(unsigned long)) { 00036 *(pulDest++) = *(pulSrc++); 00037 } 00038 } else { 00039 pulDest = (unsigned long*)(void*)((char*)pulDest + ByteCount); 00040 } 00041 00042 ByteCount = pCS3Regions->ZeroSizeRam; 00043 for(i = 0 ; i < ByteCount ; i += sizeof(unsigned long)) { 00044 *(pulDest++) = 0; 00045 } 00046 00047 heap_pointer = &__cs3_heap_start; 00048 __libc_init_array(); 00049 exit(main()); 00050 } 00051 00052 int _kill(int pid, int sig) { 00053 errno = EINVAL; 00054 return -1; 00055 } 00056 00057 void _exit(int status) { 00058 exit(status); 00059 } 00060 00061 int _getpid(void) { 00062 return 1; 00063 } 00064 00065 void *_sbrk(unsigned int incr) { 00066 void *mem; 00067 00068 unsigned int next = ((((unsigned int)heap_pointer + incr) + 7) & ~7); 00069 if (next > __get_MSP()) { 00070 mem = NULL; 00071 } else { 00072 mem = (void *)heap_pointer; 00073 } 00074 heap_pointer = (void *)next; 00075 00076 return mem; 00077 } 00078 00079 }
Generated on Tue Jul 12 2022 13:47:02 by
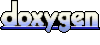