fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
rtc_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "rtc_api.h" 00017 00018 #if DEVICE_RTC 00019 00020 static void init(void) { 00021 // enable PORTC clock 00022 SIM->SCGC5 |= SIM_SCGC5_PORTC_MASK; 00023 00024 // enable RTC clock 00025 SIM->SCGC6 |= SIM_SCGC6_RTC_MASK; 00026 00027 /* 00028 * configure PTC1 with alternate function 1: RTC_CLKIN 00029 * As the kl25z board does not have a 32kHz osc, 00030 * we use an external clock generated by the 00031 * interface chip 00032 */ 00033 PORTC->PCR[1] &= ~PORT_PCR_MUX_MASK; 00034 PORTC->PCR[1] = PORT_PCR_MUX(1); 00035 00036 // select RTC_CLKIN as RTC clock source 00037 SIM->SOPT1 &= ~SIM_SOPT1_OSC32KSEL_MASK; 00038 SIM->SOPT1 |= SIM_SOPT1_OSC32KSEL(2); 00039 } 00040 00041 void rtc_init(void) { 00042 init(); 00043 00044 //Configure the TSR. default value: 1 00045 RTC->TSR = 1; 00046 00047 // enable counter 00048 RTC->SR |= RTC_SR_TCE_MASK; 00049 } 00050 00051 void rtc_free(void) { 00052 // [TODO] 00053 } 00054 00055 /* 00056 * Little check routine to see if the RTC has been enabled 00057 * 0 = Disabled, 1 = Enabled 00058 */ 00059 int rtc_isenabled(void) { 00060 // even if the RTC module is enabled, 00061 // as we use RTC_CLKIN and an external clock, 00062 // we need to reconfigure the pins. That is why we 00063 // call init() if the rtc is enabled 00064 00065 // if RTC not enabled return 0 00066 SIM->SCGC5 |= SIM_SCGC5_PORTC_MASK; 00067 SIM->SCGC6 |= SIM_SCGC6_RTC_MASK; 00068 if ((RTC->SR & RTC_SR_TCE_MASK) == 0) 00069 return 0; 00070 00071 init(); 00072 return 1; 00073 } 00074 00075 time_t rtc_read(void) { 00076 return RTC->TSR; 00077 } 00078 00079 void rtc_write(time_t t) { 00080 // disable counter 00081 RTC->SR &= ~RTC_SR_TCE_MASK; 00082 00083 // we do not write 0 into TSR 00084 // to avoid invalid time 00085 if (t == 0) 00086 t = 1; 00087 00088 // write seconds 00089 RTC->TSR = t; 00090 00091 // re-enable counter 00092 RTC->SR |= RTC_SR_TCE_MASK; 00093 } 00094 00095 #endif
Generated on Tue Jul 12 2022 13:47:01 by
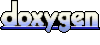