fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
pinmap.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "pinmap.h" 00017 #include "error.h" 00018 00019 void pin_function(PinName pin, int function) { 00020 if (pin == (uint32_t)NC) return; 00021 00022 uint32_t port_n = (uint32_t)pin >> PORT_SHIFT; 00023 uint32_t pin_n = (uint32_t)(pin & 0x7C) >> 2; 00024 00025 SIM->SCGC5 |= 1 << (SIM_SCGC5_PORTA_SHIFT + port_n); 00026 __IO uint32_t* pin_pcr = &(((PORT_Type *)(PORTA_BASE + 0x1000 * port_n)))->PCR[pin_n]; 00027 00028 // pin mux bits: [10:8] -> 11100000000 = (0x700) 00029 *pin_pcr = (*pin_pcr & ~0x700) | (function << 8); 00030 } 00031 00032 void pin_mode(PinName pin, PinMode mode) { 00033 if (pin == (uint32_t)NC) { return; } 00034 00035 __IO uint32_t* pin_pcr = (__IO uint32_t*)(PORTA_BASE + pin); 00036 00037 // pin pullup bits: [1:0] -> 11 = (0x3) 00038 *pin_pcr = (*pin_pcr & ~0x3) | mode; 00039 }
Generated on Tue Jul 12 2022 13:47:01 by
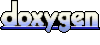