fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
i2c_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "i2c_api.h" 00017 00018 #include "cmsis.h" 00019 #include "pinmap.h" 00020 #include "error.h" 00021 00022 static const PinMap PinMap_I2C_SDA[] = { 00023 {PTE25, I2C_0, 5}, 00024 {PTC9, I2C_0, 2}, 00025 {PTE0, I2C_1, 6}, 00026 {PTB1, I2C_0, 2}, 00027 {PTB3, I2C_0, 2}, 00028 {NC , NC , 0} 00029 }; 00030 00031 static const PinMap PinMap_I2C_SCL[] = { 00032 {PTE24, I2C_0, 5}, 00033 {PTC8, I2C_0, 2}, 00034 {PTE1, I2C_1, 6}, 00035 {PTB0, I2C_0, 2}, 00036 {PTB2, I2C_0, 2}, 00037 {NC , NC, 0} 00038 }; 00039 00040 static const uint16_t ICR[0x40] = { 00041 20, 22, 24, 26, 28, 00042 30, 34, 40, 28, 32, 00043 36, 40, 44, 48, 56, 00044 68, 48, 56, 64, 72, 00045 80, 88, 104, 128, 80, 00046 96, 112, 128, 144, 160, 00047 192, 240, 160, 192, 224, 00048 256, 288, 320, 384, 480, 00049 320, 384, 448, 512, 576, 00050 640, 768, 960, 640, 768, 00051 896, 1024, 1152, 1280, 1536, 00052 1920, 1280, 1536, 1792, 2048, 00053 2304, 2560, 3072, 3840 00054 }; 00055 00056 static uint8_t first_read; 00057 00058 00059 void i2c_init(i2c_t *obj, PinName sda, PinName scl) { 00060 // determine the I2C to use 00061 I2CName i2c_sda = (I2CName)pinmap_peripheral(sda, PinMap_I2C_SDA); 00062 I2CName i2c_scl = (I2CName)pinmap_peripheral(scl, PinMap_I2C_SCL); 00063 obj->i2c = (I2C_Type*)pinmap_merge(i2c_sda, i2c_scl); 00064 if ((int)obj->i2c == NC) { 00065 error("I2C pin mapping failed"); 00066 } 00067 00068 // enable power 00069 switch ((int)obj->i2c) { 00070 case I2C_0: SIM->SCGC5 |= 1 << 13; SIM->SCGC4 |= 1 << 6; break; 00071 case I2C_1: SIM->SCGC5 |= 1 << 11; SIM->SCGC4 |= 1 << 7; break; 00072 } 00073 00074 // set default frequency at 100k 00075 i2c_frequency(obj, 100000); 00076 00077 // enable I2C interface 00078 obj->i2c->C1 |= 0x80; 00079 00080 pinmap_pinout(sda, PinMap_I2C_SDA); 00081 pinmap_pinout(scl, PinMap_I2C_SCL); 00082 00083 first_read = 1; 00084 } 00085 00086 int i2c_start(i2c_t *obj) { 00087 // if we are in the middle of a transaction 00088 // activate the repeat_start flag 00089 if (obj->i2c->S & I2C_S_BUSY_MASK) { 00090 obj->i2c->C1 |= 0x04; 00091 } else { 00092 obj->i2c->C1 |= I2C_C1_MST_MASK; 00093 obj->i2c->C1 |= I2C_C1_TX_MASK; 00094 } 00095 first_read = 1; 00096 return 0; 00097 } 00098 00099 int i2c_stop(i2c_t *obj) { 00100 volatile uint32_t n = 0; 00101 obj->i2c->C1 &= ~I2C_C1_MST_MASK; 00102 obj->i2c->C1 &= ~I2C_C1_TX_MASK; 00103 00104 // It seems that there are timing problems 00105 // when there is no waiting time after a STOP. 00106 // This wait is also included on the samples 00107 // code provided with the freedom board 00108 for (n = 0; n < 100; n++) __NOP(); 00109 first_read = 1; 00110 return 0; 00111 } 00112 00113 static int timeout_status_poll(i2c_t *obj, uint32_t mask) { 00114 uint32_t i, timeout = 1000; 00115 00116 for (i = 0; i < timeout; i++) { 00117 if (obj->i2c->S & mask) 00118 return 0; 00119 } 00120 00121 return 1; 00122 } 00123 00124 // this function waits the end of a tx transfer and return the status of the transaction: 00125 // 0: OK ack received 00126 // 1: OK ack not received 00127 // 2: failure 00128 static int i2c_wait_end_tx_transfer(i2c_t *obj) { 00129 00130 // wait for the interrupt flag 00131 if (timeout_status_poll(obj, I2C_S_IICIF_MASK)) { 00132 return 2; 00133 } 00134 00135 obj->i2c->S |= I2C_S_IICIF_MASK; 00136 00137 // wait transfer complete 00138 if (timeout_status_poll(obj, I2C_S_TCF_MASK)) { 00139 return 2; 00140 } 00141 00142 // check if we received the ACK or not 00143 return obj->i2c->S & I2C_S_RXAK_MASK ? 1 : 0; 00144 } 00145 00146 // this function waits the end of a rx transfer and return the status of the transaction: 00147 // 0: OK 00148 // 1: failure 00149 static int i2c_wait_end_rx_transfer(i2c_t *obj) { 00150 // wait for the end of the rx transfer 00151 if (timeout_status_poll(obj, I2C_S_IICIF_MASK)) { 00152 return 1; 00153 } 00154 00155 obj->i2c->S |= I2C_S_IICIF_MASK; 00156 00157 return 0; 00158 } 00159 00160 static void i2c_send_nack(i2c_t *obj) { 00161 obj->i2c->C1 |= I2C_C1_TXAK_MASK; // NACK 00162 } 00163 00164 static void i2c_send_ack(i2c_t *obj) { 00165 obj->i2c->C1 &= ~I2C_C1_TXAK_MASK; // ACK 00166 } 00167 00168 static int i2c_do_write(i2c_t *obj, int value) { 00169 // write the data 00170 obj->i2c->D = value; 00171 00172 // init and wait the end of the transfer 00173 return i2c_wait_end_tx_transfer(obj); 00174 } 00175 00176 static int i2c_do_read(i2c_t *obj, char * data, int last) { 00177 if (last) 00178 i2c_send_nack(obj); 00179 else 00180 i2c_send_ack(obj); 00181 00182 *data = (obj->i2c->D & 0xFF); 00183 00184 // start rx transfer and wait the end of the transfer 00185 return i2c_wait_end_rx_transfer(obj); 00186 } 00187 00188 void i2c_frequency(i2c_t *obj, int hz) { 00189 uint8_t icr = 0; 00190 uint8_t mult = 0; 00191 uint32_t error = 0; 00192 uint32_t p_error = 0xffffffff; 00193 uint32_t ref = 0; 00194 uint8_t i, j; 00195 // bus clk 00196 uint32_t PCLK = 24000000u; 00197 uint32_t pulse = PCLK / (hz * 2); 00198 00199 // we look for the values that minimize the error 00200 00201 // test all the MULT values 00202 for (i = 1; i < 5; i*=2) { 00203 for (j = 0; j < 0x40; j++) { 00204 ref = PCLK / (i*ICR[j]); 00205 error = (ref > hz) ? ref - hz : hz - ref; 00206 if (error < p_error) { 00207 icr = j; 00208 mult = i/2; 00209 p_error = error; 00210 } 00211 } 00212 } 00213 pulse = icr | (mult << 6); 00214 00215 // I2C Rate 00216 obj->i2c->F = pulse; 00217 } 00218 00219 int i2c_read(i2c_t *obj, int address, char *data, int length, int stop) { 00220 uint8_t count; 00221 char dummy_read, *ptr; 00222 00223 if (i2c_start(obj)) { 00224 i2c_stop(obj); 00225 return 1; 00226 } 00227 00228 if (i2c_do_write(obj, (address | 0x01))) { 00229 i2c_stop(obj); 00230 return 1; 00231 } 00232 00233 // set rx mode 00234 obj->i2c->C1 &= ~I2C_C1_TX_MASK; 00235 00236 // Read in bytes 00237 for (count = 0; count < (length); count++) { 00238 ptr = (count == 0) ? &dummy_read : &data[count - 1]; 00239 uint8_t stop_ = (count == (length - 1)) ? 1 : 0; 00240 if (i2c_do_read(obj, ptr, stop_)) { 00241 i2c_stop(obj); 00242 return 1; 00243 } 00244 } 00245 00246 // If not repeated start, send stop. 00247 if (stop) { 00248 i2c_stop(obj); 00249 } 00250 00251 // last read 00252 data[count-1] = obj->i2c->D; 00253 00254 return 0; 00255 } 00256 int i2c_write(i2c_t *obj, int address, const char *data, int length, int stop) { 00257 int i; 00258 00259 if (i2c_start(obj)) { 00260 i2c_stop(obj); 00261 return 1; 00262 } 00263 00264 if (i2c_do_write(obj, (address & 0xFE))) { 00265 i2c_stop(obj); 00266 return 1; 00267 } 00268 00269 for (i = 0; i < length; i++) { 00270 if(i2c_do_write(obj, data[i])) { 00271 i2c_stop(obj); 00272 return 1; 00273 } 00274 } 00275 00276 if (stop) { 00277 i2c_stop(obj); 00278 } 00279 00280 return 0; 00281 } 00282 00283 void i2c_reset(i2c_t *obj) { 00284 i2c_stop(obj); 00285 } 00286 00287 int i2c_byte_read(i2c_t *obj, int last) { 00288 char data; 00289 00290 // set rx mode 00291 obj->i2c->C1 &= ~I2C_C1_TX_MASK; 00292 00293 if(first_read) { 00294 // first dummy read 00295 i2c_do_read(obj, &data, 0); 00296 first_read = 0; 00297 } 00298 00299 if (last) { 00300 // set tx mode 00301 obj->i2c->C1 |= I2C_C1_TX_MASK; 00302 return obj->i2c->D; 00303 } 00304 00305 i2c_do_read(obj, &data, last); 00306 00307 return data; 00308 } 00309 00310 int i2c_byte_write(i2c_t *obj, int data) { 00311 first_read = 1; 00312 00313 // set tx mode 00314 obj->i2c->C1 |= I2C_C1_TX_MASK; 00315 00316 return !i2c_do_write(obj, (data & 0xFF)); 00317 } 00318 00319 00320 #if DEVICE_I2CSLAVE 00321 void i2c_slave_mode(i2c_t *obj, int enable_slave) { 00322 if (enable_slave) { 00323 // set slave mode 00324 obj->i2c->C1 &= ~I2C_C1_MST_MASK; 00325 obj->i2c->C1 |= I2C_C1_IICIE_MASK; 00326 } else { 00327 // set master mode 00328 obj->i2c->C1 |= I2C_C1_MST_MASK; 00329 } 00330 } 00331 00332 int i2c_slave_receive(i2c_t *obj) { 00333 switch(obj->i2c->S) { 00334 // read addressed 00335 case 0xE6: return 1; 00336 00337 // write addressed 00338 case 0xE2: return 3; 00339 00340 default: return 0; 00341 } 00342 } 00343 00344 int i2c_slave_read(i2c_t *obj, char *data, int length) { 00345 uint8_t dummy_read, count; 00346 uint8_t * ptr; 00347 00348 // set rx mode 00349 obj->i2c->C1 &= ~I2C_C1_TX_MASK; 00350 00351 // first dummy read 00352 dummy_read = obj->i2c->D; 00353 if(i2c_wait_end_rx_transfer(obj)) { 00354 return 0; 00355 } 00356 00357 // read address 00358 dummy_read = obj->i2c->D; 00359 if(i2c_wait_end_rx_transfer(obj)) { 00360 return 0; 00361 } 00362 00363 // read (length - 1) bytes 00364 for (count = 0; count < (length - 1); count++) { 00365 data[count] = obj->i2c->D; 00366 if(i2c_wait_end_rx_transfer(obj)) { 00367 return 0; 00368 } 00369 } 00370 00371 // read last byte 00372 ptr = (length == 0) ? &dummy_read : (uint8_t *)&data[count]; 00373 *ptr = obj->i2c->D; 00374 00375 return (length) ? (count + 1) : 0; 00376 } 00377 00378 int i2c_slave_write(i2c_t *obj, const char *data, int length) { 00379 uint32_t i, count = 0; 00380 00381 // set tx mode 00382 obj->i2c->C1 |= I2C_C1_TX_MASK; 00383 00384 for (i = 0; i < length; i++) { 00385 if(i2c_do_write(obj, data[count++]) == 2) { 00386 return 0; 00387 } 00388 } 00389 00390 // set rx mode 00391 obj->i2c->C1 &= ~I2C_C1_TX_MASK; 00392 00393 // dummy rx transfer needed 00394 // otherwise the master cannot generate a stop bit 00395 obj->i2c->D; 00396 if(i2c_wait_end_rx_transfer(obj) == 2) { 00397 return 0; 00398 } 00399 00400 return count; 00401 } 00402 00403 void i2c_slave_address(i2c_t *obj, int idx, uint32_t address, uint32_t mask) { 00404 obj->i2c->A1 = address & 0xfe; 00405 } 00406 #endif 00407
Generated on Tue Jul 12 2022 13:47:01 by
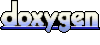