fix for mbed lib issue 3 (i2c problem) see also https://mbed.org/users/mbed_official/code/mbed/issues/3 affected implementations: LPC812, LPC11U24, LPC1768, LPC2368, LPC4088
Fork of mbed-src by
analogout_api.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "analogout_api.h" 00017 00018 #include "cmsis.h" 00019 #include "pinmap.h" 00020 #include "error.h" 00021 00022 #define RANGE_12BIT 0xFFF 00023 00024 static const PinMap PinMap_DAC[] = { 00025 {PTE30, DAC_0, 0}, 00026 {NC , NC , 0} 00027 }; 00028 00029 void analogout_init(dac_t *obj, PinName pin) { 00030 obj->dac = (DACName)pinmap_peripheral(pin, PinMap_DAC); 00031 if (obj->dac == (uint32_t)NC) { 00032 error("DAC pin mapping failed"); 00033 } 00034 00035 SIM->SCGC6 |= SIM_SCGC6_DAC0_MASK; 00036 00037 uint32_t port = (uint32_t)pin >> PORT_SHIFT; 00038 SIM->SCGC5 |= 1 << (SIM_SCGC5_PORTA_SHIFT + port); 00039 00040 DAC0->DAT[obj->dac].DATH = 0; 00041 DAC0->DAT[obj->dac].DATL = 0; 00042 00043 DAC0->C1 = DAC_C1_DACBFMD_MASK; // One-Time Scan Mode 00044 00045 DAC0->C0 = DAC_C0_DACEN_MASK // Enable 00046 | DAC_C0_DACSWTRG_MASK; // Software Trigger 00047 00048 pinmap_pinout(pin, PinMap_DAC); 00049 00050 analogout_write_u16(obj, 0); 00051 } 00052 00053 void analogout_free(dac_t *obj) {} 00054 00055 static inline void dac_write(dac_t *obj, int value) { 00056 DAC0->DAT[obj->dac].DATL = (uint8_t)( value & 0xFF); 00057 DAC0->DAT[obj->dac].DATH = (uint8_t)((value >> 8) & 0xFF); 00058 } 00059 00060 static inline int dac_read(dac_t *obj) { 00061 return ((DAC0->DAT[obj->dac].DATH << 8) | DAC0->DAT[obj->dac].DATL); 00062 } 00063 00064 void analogout_write(dac_t *obj, float value) { 00065 if (value < 0.0) { 00066 dac_write(obj, 0); 00067 } else if (value > 1.0) { 00068 dac_write(obj, RANGE_12BIT); 00069 } else { 00070 dac_write(obj, value * (float)RANGE_12BIT); 00071 } 00072 } 00073 00074 void analogout_write_u16(dac_t *obj, uint16_t value) { 00075 dac_write(obj, value >> 4); // 12-bit 00076 } 00077 00078 float analogout_read(dac_t *obj) { 00079 uint32_t value = dac_read(obj); 00080 return (float)value * (1.0f / (float)RANGE_12BIT); 00081 } 00082 00083 uint16_t analogout_read_u16(dac_t *obj) { 00084 uint32_t value = dac_read(obj); // 12-bit 00085 return (value << 4) | ((value >> 8) & 0x003F); 00086 }
Generated on Tue Jul 12 2022 13:47:00 by
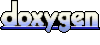