Программа считывает показания датчиков и управляет сервомашинками.
Fork of NUCLEO_BLUENRG by
sample_service.cpp
00001 /******************************************************************************/ 00002 /******************************************************************************/ 00003 #include "mbed.h" 00004 #include "sample_service.h" 00005 00006 //Set client address here 00007 //#define CLIENT_ADDRESS {0x36, 0x1F, 0xAC, 0x29, 0x6A, 0xBC} 00008 #define CLIENT_ADDRESS {0x05, 0x25, 0xAC, 0x29, 0x6A, 0xBC}; 00009 00010 volatile int connected = FALSE; 00011 volatile uint8_t set_connectable = 1; 00012 volatile uint16_t connection_handle = 0; 00013 volatile uint8_t notification_enabled = FALSE; 00014 volatile uint8_t read_finished = FALSE; 00015 00016 uint8_t *readData; 00017 uint8_t dataLength; 00018 00019 extern Serial pc; 00020 /******************************************************************************/ 00021 /******************************************************************************/ 00022 00023 #define COPY_UUID_128(uuid_struct, uuid_15, uuid_14, uuid_13, uuid_12, uuid_11, uuid_10, uuid_9, uuid_8, uuid_7, uuid_6, uuid_5, uuid_4, uuid_3, uuid_2, uuid_1, uuid_0) \ 00024 do {\ 00025 uuid_struct.uuid128[0] = uuid_0; uuid_struct.uuid128[1] = uuid_1; uuid_struct.uuid128[2] = uuid_2; uuid_struct.uuid128[3] = uuid_3; \ 00026 uuid_struct.uuid128[4] = uuid_4; uuid_struct.uuid128[5] = uuid_5; uuid_struct.uuid128[6] = uuid_6; uuid_struct.uuid128[7] = uuid_7; \ 00027 uuid_struct.uuid128[8] = uuid_8; uuid_struct.uuid128[9] = uuid_9; uuid_struct.uuid128[10] = uuid_10; uuid_struct.uuid128[11] = uuid_11; \ 00028 uuid_struct.uuid128[12] = uuid_12; uuid_struct.uuid128[13] = uuid_13; uuid_struct.uuid128[14] = uuid_14; uuid_struct.uuid128[15] = uuid_15; \ 00029 }while(0) 00030 00031 00032 00033 /******************************************************************************/ 00034 /******************************************************************************/ 00035 void Make_Connection(void) 00036 { 00037 tBleStatus ret; 00038 00039 // 00040 tBDAddr bdaddr = CLIENT_ADDRESS; 00041 00042 ret = aci_gap_create_connection(0x4000, 0x4000, PUBLIC_ADDR, bdaddr, PUBLIC_ADDR, 9, 9, 0, 60, 1000 , 1000); 00043 00044 if (ret != 0) { 00045 pc.printf("Error while starting connection.\n"); 00046 Clock_Wait(100); 00047 } 00048 00049 } 00050 /******************************************************************************/ 00051 /******************************************************************************/ 00052 void sendData(uint16_t handle, uint8_t* data_buffer, uint8_t Nb_bytes) 00053 { 00054 aci_gatt_write_without_response(connection_handle, handle, Nb_bytes, data_buffer); 00055 } 00056 00057 /******************************************************************************/ 00058 /******************************************************************************/ 00059 00060 uint8_t *readValue(unsigned short handle, uint8_t* len) 00061 { 00062 volatile unsigned char res; 00063 static uint32_t timeout = 0; 00064 00065 res = aci_gatt_read_charac_val(connection_handle, handle); 00066 00067 timeout = 0; 00068 while ((!read_finished)&&(timeout < READ_TIMEOUT)) 00069 { 00070 HCI_Process(); 00071 timeout++; 00072 } 00073 if (timeout == READ_TIMEOUT) 00074 { 00075 dataLength = 0; 00076 } 00077 00078 *len = dataLength; 00079 00080 read_finished = 0; 00081 return readData; 00082 00083 } 00084 00085 /******************************************************************************/ 00086 /******************************************************************************/ 00087 void enableNotification() 00088 { 00089 uint8_t client_char_conf_data[] = {0x01, 0x00}; // Enable notifications 00090 struct timer t; 00091 Timer_Set(&t, CLOCK_SECOND*10); 00092 00093 while(aci_gatt_write_charac_descriptor(connection_handle, 0x0060, 2, client_char_conf_data)==BLE_STATUS_NOT_ALLOWED){ 00094 /* Radio is busy */ 00095 if(Timer_Expired(&t)) break; 00096 } 00097 notification_enabled = TRUE; 00098 } 00099 00100 /******************************************************************************/ 00101 /******************************************************************************/ 00102 void GAP_ConnectionComplete_CB(uint8_t addr[6], uint16_t handle) 00103 { 00104 connected = TRUE; 00105 connection_handle = handle; 00106 00107 } 00108 00109 /******************************************************************************/ 00110 /******************************************************************************/ 00111 void GAP_DisconnectionComplete_CB(void) 00112 { 00113 connected = FALSE; 00114 set_connectable = TRUE; 00115 notification_enabled = FALSE; 00116 } 00117 00118 /******************************************************************************/ 00119 /******************************************************************************/ 00120 00121 void GATT_Notification_CB(uint16_t attr_handle, uint8_t attr_len, uint8_t *attr_value) 00122 { 00123 //reserved 00124 } 00125 00126 /******************************************************************************/ 00127 /******************************************************************************/ 00128 00129 void GATT_Read_CB(uint16_t attr_handle, uint8_t attr_len, uint8_t *attr_value) 00130 { 00131 readData = attr_value; 00132 dataLength = attr_len; 00133 read_finished = 1; 00134 } 00135 00136 /******************************************************************************/ 00137 /******************************************************************************/ 00138 00139 void HCI_Event_CB(void *pckt) 00140 { 00141 hci_uart_pckt *hci_pckt = (hci_uart_pckt *)pckt; 00142 hci_event_pckt *event_pckt = (hci_event_pckt*)hci_pckt->data; 00143 00144 if(hci_pckt->type != HCI_EVENT_PKT) 00145 return; 00146 00147 switch(event_pckt->evt){ 00148 00149 case EVT_DISCONN_COMPLETE: 00150 { 00151 GAP_DisconnectionComplete_CB(); 00152 } 00153 break; 00154 00155 case EVT_LE_META_EVENT: 00156 { 00157 evt_le_meta_event *evt = (evt_le_meta_event *)event_pckt->data; 00158 00159 switch(evt->subevent){ 00160 case EVT_LE_CONN_COMPLETE: 00161 { 00162 evt_le_connection_complete *cc = (evt_le_connection_complete *)evt->data; 00163 GAP_ConnectionComplete_CB(cc->peer_bdaddr, cc->handle); 00164 } 00165 break; 00166 } 00167 } 00168 break; 00169 00170 case EVT_VENDOR: 00171 { 00172 evt_blue_aci *blue_evt = (evt_blue_aci *)event_pckt->data; 00173 switch(blue_evt->ecode){ 00174 00175 case EVT_BLUE_GATT_NOTIFICATION: { 00176 evt_gatt_attr_notification *evt = (evt_gatt_attr_notification*)blue_evt->data; 00177 GATT_Notification_CB(evt->attr_handle, evt->data_length - 2, evt->attr_value); 00178 } 00179 break; 00180 case EVT_BLUE_ATT_READ_RESP: { 00181 evt_gatt_procedure_complete *evt = (evt_gatt_procedure_complete*)blue_evt->data; 00182 GATT_Read_CB(evt->conn_handle, evt->data_length, evt->data); 00183 00184 } 00185 break; 00186 } 00187 } 00188 break; 00189 } 00190 } 00191 /******************************************************************************/ 00192 /******************************************************************************/ 00193 00194 /*****************************END OF FILE**************************************/
Generated on Tue Jul 12 2022 22:44:50 by
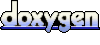