Программа считывает показания датчиков и управляет сервомашинками.
Fork of NUCLEO_BLUENRG by
main.cpp
00001 #include "mbed.h" 00002 #include "tag_math.h" 00003 #include "stm32f4xx_hal.h" 00004 #include "bluenrg_shield_bsp.h" 00005 #include "osal.h" 00006 #include "sample_service.h" 00007 #include <stdio.h> 00008 00009 #define BDADDR_SIZE 6 00010 00011 /* SPI handler declaration */ 00012 SPI_HandleTypeDef SpiHandle; 00013 00014 Serial pc(SERIAL_TX, SERIAL_RX); 00015 PwmOut mypwm(D2); 00016 AnalogIn analog_value(A2); 00017 int flag = 0; 00018 00019 extern volatile uint8_t set_connectable; 00020 extern volatile int connected; 00021 extern volatile uint8_t notification_enabled; 00022 00023 void User_Process(void); 00024 00025 00026 /******************************************************************************/ 00027 /******************************************************************************/ 00028 int main(void) 00029 { 00030 tHalUint8 CLIENT_BDADDR[] = {0xbb, 0x00, 0x00, 0xE1, 0x80, 0x02}; 00031 00032 tHalUint8 bdaddr[BDADDR_SIZE]; 00033 00034 uint16_t service_handle, dev_name_char_handle, appearance_char_handle; 00035 int ret; 00036 00037 /* Hardware init*/ 00038 HAL_Init(); 00039 00040 /* Configure the system clock */ 00041 SystemClock_Config(); 00042 00043 mypwm.period_ms(20); 00044 //mypwm.pulsewidth_ms(1.5); 00045 00046 /* Initialize the BlueNRG SPI driver */ 00047 BNRG_SPI_Init(); 00048 00049 00050 /* Initialize the BlueNRG HCI */ 00051 HCI_Init(); 00052 00053 /* Reset BlueNRG hardware */ 00054 BlueNRG_RST(); 00055 00056 Osal_MemCpy(bdaddr, CLIENT_BDADDR, sizeof(CLIENT_BDADDR)); 00057 00058 ret = aci_hal_write_config_data(CONFIG_DATA_PUBADDR_OFFSET, 00059 CONFIG_DATA_PUBADDR_LEN, 00060 bdaddr); 00061 if(ret){ 00062 pc.printf("Setting BD_ADDR failed.\n"); 00063 } 00064 00065 ret = aci_gatt_init(); 00066 if(ret){ 00067 pc.printf("GATT_Init failed.\n"); 00068 } 00069 00070 ret = aci_gap_init(GAP_CENTRAL_ROLE, &service_handle, &dev_name_char_handle, &appearance_char_handle); 00071 00072 if(ret != BLE_STATUS_SUCCESS){ 00073 pc.printf("GAP_Init failed.\n"); 00074 } 00075 00076 ret = aci_gap_set_auth_requirement(MITM_PROTECTION_REQUIRED, 00077 OOB_AUTH_DATA_ABSENT, 00078 NULL, 00079 7, 00080 16, 00081 USE_FIXED_PIN_FOR_PAIRING, 00082 123456, 00083 BONDING); 00084 00085 00086 if (ret != BLE_STATUS_SUCCESS) { 00087 pc.printf("BLE Stack Initialization failed.\n"); 00088 } 00089 00090 /* Set output power level */ 00091 ret = aci_hal_set_tx_power_level(1,4); 00092 00093 static uint32_t cnt1=0; 00094 00095 /* Infinite loop */ 00096 while (1) 00097 { 00098 /* 00099 for(float offset=0.0; offset<0.001; offset+=0.0001) { 00100 mypwm.pulsewidth(0.001 + offset); // servo position determined by a pulsewidth between 1-2ms 00101 wait(0.25); 00102 } 00103 */ 00104 00105 if (HAL_GetTick() > (cnt1 + 1000)) 00106 { 00107 cnt1=HAL_GetTick(); 00108 if (flag==0){ 00109 mypwm.period_ms(20); 00110 mypwm.pulsewidth_ms(0.9); 00111 flag++; 00112 } 00113 else if (flag==1){ 00114 mypwm.period_ms(20); 00115 mypwm.pulsewidth_ms(1.5); 00116 flag++; 00117 } 00118 else if (flag==2){ 00119 mypwm.period_ms(20); 00120 mypwm.pulsewidth_ms(2.0); 00121 flag=0; 00122 } 00123 } 00124 HCI_Process(); 00125 User_Process(); 00126 } 00127 00128 } 00129 00130 /******************************************************************************/ 00131 /******************************************************************************/ 00132 00133 void User_Process(void) 00134 { 00135 static uint32_t cnt; 00136 static uint8_t acc_en_sent = 0; 00137 tHalUint8 data[2]; 00138 uint8_t* temp; 00139 //uint8_t data_length; 00140 00141 if(set_connectable){ 00142 Make_Connection(); 00143 set_connectable = FALSE; 00144 } 00145 00146 /*if(connected && !notification_enabled) { 00147 enableNotification(); 00148 }*/ 00149 00150 if((connected)&&(!acc_en_sent)){ 00151 00152 HAL_Delay(100); 00153 data[0] = 0x01; 00154 sendData(0x0029, data, 1); 00155 00156 HAL_Delay(100); 00157 data[0] = 0x01; 00158 sendData(0x0031, data, 1); 00159 00160 HAL_Delay(100); 00161 data[0] = 0x01; 00162 sendData(0x003C, data, 1); 00163 00164 HAL_Delay(100); 00165 data[0] = 0x10; 00166 sendData(0x0034, data, 1); 00167 00168 00169 acc_en_sent = 1; 00170 cnt = HAL_GetTick(); 00171 } 00172 00173 if (HAL_GetTick() > (cnt +2000)) 00174 { 00175 cnt = HAL_GetTick(); 00176 if (connected && acc_en_sent) 00177 { 00178 00179 00180 float sheint = analog_value.read(); 00181 pc.printf("Light = %f;\n", sheint); 00182 pc.printf("T = %f; ", calcTmpTarget(readValue(0x25, NULL))); 00183 temp = readValue(0x2D, NULL); 00184 pc.printf("Ax = %d; Ay = %d; Az = %d ", (signed char) temp[0], (signed char) temp[1], (signed char) temp[2]); 00185 temp = readValue(0x38, NULL); 00186 pc.printf("H = %f; \r\n", calcHumRel(temp)); 00187 00188 00189 /*temp = readValue(0x2D, NULL); 00190 pc.printf("%d %d \r\n", (signed char) temp[0], (signed char) temp[1]);*/ 00191 00192 /*pc.printf("%d ", (signed short)calcTmpTarget(readValue(0x25, NULL))); 00193 temp = readValue(0x38, NULL); 00194 pc.printf("%d \r\n", (signed short)calcHumRel(temp));*/ 00195 00196 //GATT services scan 00197 /*for (unsigned char j = 1; j < 0x88; j++) 00198 { 00199 pc.printf("\n %.2X: ", j); 00200 uint8_t* temp = readValue(j+1, &data_length); 00201 00202 00203 for(int i = 0; i < data_length; i++) { 00204 pc.printf("%.2X:", temp[i]); 00205 } 00206 00207 for(int i = 0; i < data_length; i++) { 00208 pc.printf("%c", temp[i]); 00209 } 00210 00211 } 00212 */ 00213 00214 } 00215 } 00216 00217 } 00218 /******************************************************************************/ 00219 /******************************************************************************/ 00220 00221 /*********************** END OF FILE ******************************************/
Generated on Tue Jul 12 2022 22:44:50 by
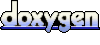