Library for Nuelectronics Nokia 3310/5110 LCD Display and joystick.
Fork of N3310LCD by
N3310LCD.h
00001 /** 00002 * @section DESCRIPTION 00003 * N3310LCD. A program to interface mbed with the nuelectronics 00004 * Nokia 3310 LCD shield from www.nuelectronics.com. Ported from 00005 * the nuelectronics Arduino code. 00006 * 00007 * @section LICENSE 00008 * 00009 * Copyright (C) <2009> Petras Saduikis <petras@petras.co.uk> 00010 * 00011 * Converted to a mbed library by Andrew D. Lindsay 00012 * 00013 * This file is part of N3310LCD. 00014 * 00015 * N3310LCD is free software: you can redistribute it and/or modify 00016 * it under the terms of the GNU General Public License as published by 00017 * the Free Software Foundation, either version 3 of the License, or 00018 * (at your option) any later version. 00019 * 00020 * N3310LCD is distributed in the hope that it will be useful, 00021 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 * GNU General Public License for more details. 00024 * 00025 * You should have received a copy of the GNU General Public License 00026 * along with N3310LCD. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 00029 #ifndef N3310LCD_H 00030 #define N3310LCD_H 00031 00032 #include <mbed.h> 00033 #include "N3310LCDDefs.h" 00034 00035 #define LCDCOLMAX 84 00036 #define LCDROWMAX 6 00037 #define LCDPIXELROWMAX 48 00038 #define PIXEL_OFF 0 00039 #define PIXEL_ON 1 00040 #define PIXEL_XOR 2 00041 #define FONT_5x7 0 00042 #define FONT_6x8 1 00043 #define FONT_ALPHA_17x17 2 00044 00045 00046 class N3310LCD : public Stream 00047 { 00048 public: 00049 /** Constructor 00050 * 00051 * @param mosi Pin name 00052 * @param miso pin name 00053 * @param sck Clock pin name 00054 * @param cd Chip enable pin name 00055 * @param dat_cmd Data/Command selection pin 00056 * @param lcd_rst Reset pin name 00057 * @param bl_on Backlight control pin 00058 */ 00059 N3310LCD(PinName mosi, PinName miso, PinName sck, 00060 PinName ce, PinName dat_cmd, PinName lcd_rst, PinName bl_on); 00061 00062 /** Main initialisation function 00063 */ 00064 void init(); 00065 00066 /** Clear LCD screen and home cursor 00067 */ 00068 void cls(); 00069 00070 /** Set backlight state, either on or off 00071 * 00072 * @param state The backlight state, ON or OFF 00073 */ 00074 void backlight(eBacklight state); 00075 00076 /** Write a single command to the LCD module 00077 * 00078 * @param data The command data to send 00079 */ 00080 void writeCommand(BYTE data); 00081 00082 /** Write a byte of data to LCD module 00083 * 00084 * @param data The data byte to send 00085 */ 00086 void writeData(BYTE data); 00087 00088 /** Move the cursor to a particular location 00089 * 00090 * @param xPos X position, 0 to 83, left to right 00091 * @param yPos Y position, 0 to 47, top to bottom 00092 */ 00093 void locate(BYTE xPos, BYTE yPos); 00094 00095 /** Copy bitmap data to display, can be smaller than full screen for partial updates 00096 * 00097 * @param xPos X position, 0 to 83 00098 * @param yPos Y position, 0 to 47 00099 * @param bitmap Bitmap data 00100 * @param bmpXSise Number of X pixels in bitmap 00101 * @param bmpYSize Number of Y pixels in bitmap 00102 */ 00103 void drawBitmap(BYTE xPos, BYTE yPos, BYTE* bitmap, BYTE bmpXSize, BYTE bmpYSize); 00104 00105 /** Clear an area of the display 00106 * 00107 * @param xPos X position, 0 to 83 00108 * @param yPos Y position, 0 to 47 00109 * @param bmpXSise Number of X pixels in bitmap 00110 * @param bmpYSize Number of Y pixels in bitmap 00111 */ 00112 void clearBitmap(BYTE xPos,BYTE yPos, BYTE size_x, BYTE size_y); 00113 00114 /** Set the font to use for future characters 00115 * 00116 * @param fornt The font to use 00117 */ 00118 void setFont(BYTE font ); 00119 00120 /** Write a string of chars to the display starting at specified position 00121 * and using specified display mode 00122 * 00123 * @param xPos X position, 0 to 83 00124 * @param yPos Y position, 0 to 47 00125 * @param string The string to display 00126 * @param mode NORMAL or HIGHLIGHT used to diplay text 00127 */ 00128 void writeString(BYTE xPos, BYTE yPos, char* string, eDisplayMode mode); 00129 00130 /** Write a string of chars to the display starting at specified position 00131 * and using specified display mode in the big font 00132 * 00133 * @param xPos X position, 0 to 83 00134 * @param yPos Y position, 0 to 47 00135 * @param string The string to display 00136 * @param mode NORMAL or HIGHLIGHT used to diplay text 00137 */ 00138 void writeStringBig(BYTE xPos, BYTE yPos, char* string, eDisplayMode mode); 00139 00140 /** Write a single character to display in specified mode 00141 * 00142 * @param ch The character to display 00143 * @param mode NORMAL or HIGHLIGHT used to diplay text 00144 */ 00145 void writeChar(BYTE ch, eDisplayMode mode); 00146 00147 /** Write a single big character to display in specified mode 00148 * 00149 * @param xPos X position, 0 to 83 00150 * @param yPos Y position, 0 to 47 00151 * @param ch The character to display 00152 * @param mode NORMAL or HIGHLIGHT used to diplay text 00153 */ 00154 void writeCharBig(BYTE xPos, BYTE yPos, BYTE ch, eDisplayMode mode); 00155 00156 /** Set a single pixel either on or off 00157 * 00158 * @param x The X position, 0 to 83 00159 * @param y The Y position, 0 to 47 00160 * @param c The state, on or off. 00161 */ 00162 void setPixel( BYTE x, BYTE y, BYTE c ); 00163 00164 /** Draw a line from one point to another in the specified colour 00165 * 00166 * @param x1 Origin X 00167 * @param y1 Origin Y 00168 * @param x2 Destination X 00169 * @param y2 Destination Y 00170 * @param c Colour, either PIXEL_ON, PIXEL_OFF or PIXEL_XOR 00171 */ 00172 void drawLine(BYTE x1, BYTE y1, BYTE x2, BYTE y2, BYTE c); 00173 00174 /** Draw a rectangle by specifying opposite corners. Can be on or off to draw or clear. 00175 * 00176 * @param x1 Origin X 00177 * @param y1 Origin Y 00178 * @param x2 Destination X 00179 * @param y2 Destination Y 00180 * @param c Colour, either PIXEL_ON, PIXEL_OFF or PIXEL_XOR 00181 */ 00182 void drawRectangle(BYTE x1, BYTE y1,BYTE x2, BYTE y2, BYTE c); 00183 00184 /** Draw a solid filled rectangle by specifying opposite corners. Can be on or off to draw or clear. 00185 * 00186 * @param x1 Origin X 00187 * @param y1 Origin Y 00188 * @param x2 Destination X 00189 * @param y2 Destination Y 00190 * @param c Colour, either PIXEL_ON, PIXEL_OFF or PIXEL_XOR 00191 */ 00192 void drawFilledRectangle(BYTE x1, BYTE y1, BYTE x2, BYTE y2, BYTE c); 00193 00194 /** Draw a circle of a given radius. Points outside of the display are not plotted allowing 00195 * circles that go outside of display to be drawn. 00196 * 00197 * @param x1 Centre X 00198 * @param y1 Centre Y 00199 * @param r Radius length 00200 * @param c Colour, either PIXEL_ON, PIXEL_OFF or PIXEL_XOR 00201 */ 00202 void drawCircle(BYTE xc, BYTE yc, BYTE r, BYTE c); 00203 00204 protected: 00205 /** Implementation of virtual functions used by printf stream 00206 */ 00207 virtual int _putc(int value); 00208 /** Dummy for stream read 00209 */ 00210 virtual int _getc(); 00211 00212 private: 00213 // I/O 00214 SPI lcdPort; // does SPI MOSI, MISO and SCK 00215 DigitalOut ceWire; // does SPI CE 00216 DigitalOut dcWire; // does 3310 DAT_CMD 00217 DigitalOut rstWire; // does 3310 LCD_RST 00218 DigitalOut blWire; // does 3310 BL_ON (backlight) 00219 }; 00220 00221 #endif
Generated on Wed Jul 13 2022 14:26:13 by
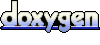