Library for Nuelectronics Nokia 3310/5110 LCD Display and joystick.
Fork of N3310LCD by
Joystick.cpp
00001 /* 00002 * N3310LCD. A program to interface mbed with the nuelectronics 00003 * Nokia 3310 LCD shield from www.nuelectronics.com. Ported from 00004 * the nuelectronics Arduino code. 00005 * 00006 * Copyright (C) <2009> Petras Saduikis <petras@petras.co.uk> 00007 * 00008 * Converted to a mbed library by Andrew D. Lindsay 00009 * 00010 * This file is part of N3310LCD. 00011 * 00012 * N3310LCD is free software: you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation, either version 3 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * N3310LCD is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License 00023 * along with N3310LCD. If not, see <http://www.gnu.org/licenses/>. 00024 */ 00025 00026 #include <mbed.h> 00027 #include "Joystick.h" 00028 00029 //keypad debounce parameter 00030 #define DEBOUNCE_MAX 15 00031 #define DEBOUNCE_ON 10 00032 #define DEBOUNCE_OFF 3 00033 00034 // values correspond to use of a 3.3V supply for the LCD shield. 00035 const int Joystick::adcKeyVal[NUM_KEYS] = {50, // LEFT 00036 200, // CENTER DEPRESSED 00037 400, // DOWN 00038 600, // UP 00039 800 // RIGHT 00040 // 1024 CENTER NOT DEPRESSED 00041 }; 00042 00043 Joystick::Joystick(PinName jstick) : joystick(jstick) 00044 { 00045 // reset button arrays 00046 for (int i = 0; i < NUM_KEYS; i++) { 00047 buttonCount[i] = 0; 00048 buttonStatus[i] = 0; 00049 buttonFlag[i] = 0; 00050 } 00051 } 00052 00053 int Joystick::getKeyState(int i) 00054 { 00055 int retval = 0; 00056 00057 if (i < NUM_KEYS) { 00058 retval = buttonFlag[i]; 00059 } 00060 00061 return retval; 00062 } 00063 00064 void Joystick::resetKeyState(int i) 00065 { 00066 if (i < NUM_KEYS) { 00067 buttonFlag[i] = 0; 00068 } 00069 } 00070 00071 void Joystick::updateADCKey() 00072 { 00073 // NOTE: the mbed analog in is 0 - 3.3V, represented as 0.0 - 1.0. It is important 00074 // that the LCD shield is powered from a 3.3V supply in order for the 'right' joystick 00075 // key to function correctly. 00076 00077 int adcKeyIn = joystick * 1024; // scale this up so we can use int 00078 int keyIn = getKey(adcKeyIn); 00079 00080 for (int i = 0; i < NUM_KEYS; i++) { 00081 if (keyIn == i) { //one key is pressed 00082 if (buttonCount[i] < DEBOUNCE_MAX) { 00083 buttonCount[i]++; 00084 if (buttonCount[i] > DEBOUNCE_ON) { 00085 if (buttonStatus[i] == 0) { 00086 buttonFlag[i] = 1; 00087 buttonStatus[i] = 1; //button debounced to 'pressed' status 00088 } 00089 } 00090 } 00091 } else { // no button pressed 00092 if (buttonCount[i] > 0) { 00093 buttonFlag[i] = 0; 00094 buttonCount[i]--; 00095 if (buttonCount[i] < DEBOUNCE_OFF) { 00096 buttonStatus[i] = 0; //button debounced to 'released' status 00097 } 00098 } 00099 } 00100 } 00101 } 00102 00103 // Convert ADC value to key number 00104 int Joystick::getKey(int input) 00105 { 00106 int k; 00107 00108 for (k = 0; k < NUM_KEYS; k++) { 00109 if (input < adcKeyVal[k]) return k; 00110 } 00111 00112 if (k >= NUM_KEYS) k = -1; // No valid key pressed 00113 00114 return k; 00115 }
Generated on Wed Jul 13 2022 14:26:13 by
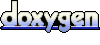