ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
util.h
00001 /*Copyright (C) 2008-2009 Timothy B. Terriberry (tterribe@xiph.org) 00002 You can redistribute this library and/or modify it under the terms of the 00003 GNU Lesser General Public License as published by the Free Software 00004 Foundation; either version 2.1 of the License, or (at your option) any later 00005 version.*/ 00006 #if !defined(_qrcode_util_H) 00007 # define _qrcode_util_H (1) 00008 00009 #define QR_MAXI(_a,_b) ((_a)-((_a)-(_b)&-((_b)>(_a)))) 00010 #define QR_MINI(_a,_b) ((_a)+((_b)-(_a)&-((_b)<(_a)))) 00011 #define QR_SIGNI(_x) (((_x)>0)-((_x)<0)) 00012 #define QR_SIGNMASK(_x) (-((_x)<0)) 00013 /*Unlike copysign(), simply inverts the sign of _a if _b is negative.*/ 00014 #define QR_FLIPSIGNI(_a,_b) ((_a)+QR_SIGNMASK(_b)^QR_SIGNMASK(_b)) 00015 #define QR_COPYSIGNI(_a,_b) QR_FLIPSIGNI(abs(_a),_b) 00016 /*Divides a signed integer by a positive value with exact rounding.*/ 00017 #define QR_DIVROUND(_x,_y) (((_x)+QR_FLIPSIGNI(_y>>1,_x))/(_y)) 00018 #define QR_CLAMPI(_a,_b,_c) (QR_MAXI(_a,QR_MINI(_b,_c))) 00019 #define QR_CLAMP255(_x) ((unsigned char)((((_x)<0)-1)&((_x)|-((_x)>255)))) 00020 /*Swaps two integers _a and _b if _a>_b.*/ 00021 #define QR_SORT2I(_a,_b) \ 00022 do{ \ 00023 int t__; \ 00024 t__=QR_MINI(_a,_b)^(_a); \ 00025 (_a)^=t__; \ 00026 (_b)^=t__; \ 00027 } \ 00028 while(0) 00029 #define QR_ILOG0(_v) (!!((_v)&0x2)) 00030 #define QR_ILOG1(_v) (((_v)&0xC)?2+QR_ILOG0((_v)>>2):QR_ILOG0(_v)) 00031 #define QR_ILOG2(_v) (((_v)&0xF0)?4+QR_ILOG1((_v)>>4):QR_ILOG1(_v)) 00032 #define QR_ILOG3(_v) (((_v)&0xFF00)?8+QR_ILOG2((_v)>>8):QR_ILOG2(_v)) 00033 #define QR_ILOG4(_v) (((_v)&0xFFFF0000)?16+QR_ILOG3((_v)>>16):QR_ILOG3(_v)) 00034 /*Computes the integer logarithm of a (positive, 32-bit) constant.*/ 00035 #define QR_ILOG(_v) ((int)QR_ILOG4((unsigned)(_v))) 00036 00037 /*Multiplies 32-bit numbers _a and _b, adds (possibly 64-bit) number _r, and 00038 takes bits [_s,_s+31] of the result.*/ 00039 #define QR_FIXMUL(_a,_b,_r,_s) ((int)((_a)*(long long)(_b)+(_r)>>(_s))) 00040 /*Multiplies 32-bit numbers _a and _b, adds (possibly 64-bit) number _r, and 00041 gives all 64 bits of the result.*/ 00042 #define QR_EXTMUL(_a,_b,_r) ((_a)*(long long)(_b)+(_r)) 00043 00044 unsigned qr_isqrt(unsigned _val); 00045 unsigned qr_ihypot(int _x,int _y); 00046 int qr_ilog(unsigned _val); 00047 00048 #endif 00049
Generated on Tue Jul 12 2022 18:54:12 by
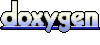