ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
timer.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _ZBAR_TIMER_H_ 00024 #define _ZBAR_TIMER_H_ 00025 00026 #include <time.h> 00027 #include <sys/time.h> /* gettimeofday */ 00028 00029 /* platform timer abstraction 00030 * 00031 * zbar_timer_t stores the absolute expiration of a delay from 00032 * when the timer was initialized. 00033 * 00034 * _zbar_timer_init() initialized timer with specified ms delay. 00035 * returns timer or NULL if timeout < 0 (no/infinite timeout) 00036 * _zbar_timer_check() returns ms remaining until expiration. 00037 * will be <= 0 if timer has expired 00038 */ 00039 00040 #if _POSIX_TIMERS > 0 00041 00042 typedef struct timespec zbar_timer_t; 00043 00044 static inline zbar_timer_t *_zbar_timer_init (zbar_timer_t *timer, 00045 int delay) 00046 { 00047 if(delay < 0) 00048 return(NULL); 00049 00050 clock_gettime(CLOCK_REALTIME, timer); 00051 timer->tv_nsec += (delay % 1000) * 1000000; 00052 timer->tv_sec += (delay / 1000) + (timer->tv_nsec / 1000000000); 00053 timer->tv_nsec %= 1000000000; 00054 return(timer); 00055 } 00056 00057 static inline int _zbar_timer_check (zbar_timer_t *timer) 00058 { 00059 if(!timer) 00060 return(-1); 00061 00062 struct timespec now; 00063 clock_gettime(CLOCK_REALTIME, &now); 00064 int delay = ((timer->tv_sec - now.tv_sec) * 1000 + 00065 (timer->tv_nsec - now.tv_nsec) / 1000000); 00066 return((delay >= 0) ? delay : 0); 00067 } 00068 00069 00070 #elif defined(_WIN32) 00071 00072 # include <windows.h> 00073 00074 typedef DWORD zbar_timer_t; 00075 00076 static inline zbar_timer_t *_zbar_timer_init (zbar_timer_t *timer, 00077 int delay) 00078 { 00079 if(delay < 0) 00080 return(NULL); 00081 00082 *timer = timeGetTime() + delay; 00083 return(timer); 00084 } 00085 00086 static inline int _zbar_timer_check (zbar_timer_t *timer) 00087 { 00088 if(!timer) 00089 return(INFINITE); 00090 00091 int delay = *timer - timeGetTime(); 00092 return((delay >= 0) ? delay : 0); 00093 } 00094 00095 00096 #else 00097 00098 typedef struct timeval zbar_timer_t; 00099 00100 static inline zbar_timer_t *_zbar_timer_init (zbar_timer_t *timer, 00101 int delay) 00102 { 00103 if(delay < 0) 00104 return(NULL); 00105 00106 gettimeofday(timer, NULL); 00107 timer->tv_usec += (delay % 1000) * 1000; 00108 timer->tv_sec += (delay / 1000) + (timer->tv_usec / 1000000); 00109 timer->tv_usec %= 1000000; 00110 return(timer); 00111 } 00112 00113 static inline int _zbar_timer_check (zbar_timer_t *timer) 00114 { 00115 if(!timer) 00116 return(-1); 00117 00118 struct timeval now; 00119 gettimeofday(&now, NULL); 00120 return((timer->tv_sec - now.tv_sec) * 1000 + 00121 (timer->tv_usec - now.tv_usec) / 1000); 00122 } 00123 00124 #endif 00125 00126 #endif 00127
Generated on Tue Jul 12 2022 18:54:12 by
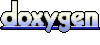