ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
thread.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _ZBAR_THREAD_H_ 00024 #define _ZBAR_THREAD_H_ 00025 00026 /* simple platform thread abstraction 00027 */ 00028 00029 #include <config.h> 00030 #include "event.h" 00031 00032 #if defined(_WIN32) 00033 00034 # include <windows.h> 00035 # define HAVE_THREADS 00036 # define ZTHREAD DWORD WINAPI 00037 00038 typedef ZTHREAD (zbar_thread_proc_t)(void*); 00039 00040 typedef DWORD zbar_thread_id_t; 00041 00042 #elif defined(HAVE_LIBPTHREAD) 00043 00044 # include <pthread.h> 00045 # include <signal.h> 00046 # define HAVE_THREADS 00047 # define ZTHREAD void* 00048 00049 typedef ZTHREAD (zbar_thread_proc_t)(void*); 00050 00051 typedef pthread_t zbar_thread_id_t; 00052 00053 #else 00054 00055 # undef HAVE_THREADS 00056 # undef ZTHREAD 00057 00058 typedef void zbar_thread_proc_t; 00059 typedef int zbar_thread_id_t; 00060 00061 #endif 00062 00063 00064 typedef struct zbar_thread_s { 00065 zbar_thread_id_t tid; 00066 int started, running; 00067 zbar_event_t notify, activity; 00068 } zbar_thread_t; 00069 00070 00071 #if defined(_WIN32) 00072 00073 static inline void _zbar_thread_init (zbar_thread_t *thr) 00074 { 00075 thr->running = 1; 00076 _zbar_event_trigger(&thr->activity); 00077 } 00078 00079 static inline zbar_thread_id_t _zbar_thread_self () 00080 { 00081 return(GetCurrentThreadId()); 00082 } 00083 00084 static inline int _zbar_thread_is_self (zbar_thread_id_t tid) 00085 { 00086 return(tid == GetCurrentThreadId()); 00087 } 00088 00089 00090 #elif defined(HAVE_LIBPTHREAD) 00091 00092 static inline void _zbar_thread_init (zbar_thread_t *thr) 00093 { 00094 sigset_t sigs; 00095 sigfillset(&sigs); 00096 pthread_sigmask(SIG_BLOCK, &sigs, NULL); 00097 thr->running = 1; 00098 _zbar_event_trigger(&thr->activity); 00099 } 00100 00101 static inline zbar_thread_id_t _zbar_thread_self (void) 00102 { 00103 return(pthread_self()); 00104 } 00105 00106 static inline int _zbar_thread_is_self (zbar_thread_id_t tid) 00107 { 00108 return(pthread_equal(tid, pthread_self())); 00109 } 00110 00111 00112 #else 00113 00114 # define _zbar_thread_start(...) -1 00115 # define _zbar_thread_stop(...) 0 00116 # define _zbar_thread_self(...) 0 00117 # define _zbar_thread_is_self(...) 1 00118 00119 #endif 00120 00121 #ifdef HAVE_THREADS 00122 extern int _zbar_thread_start(zbar_thread_t*, zbar_thread_proc_t*, 00123 void*, zbar_mutex_t*); 00124 extern int _zbar_thread_stop(zbar_thread_t*, zbar_mutex_t*); 00125 #endif 00126 00127 #endif 00128
Generated on Tue Jul 12 2022 18:54:12 by
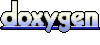