ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
rs.h
00001 /*Copyright (C) 1991-1995 Henry Minsky (hqm@ua.com, hqm@ai.mit.edu) 00002 Copyright (C) 2008-2009 Timothy B. Terriberry (tterribe@xiph.org) 00003 You can redistribute this library and/or modify it under the terms of the 00004 GNU Lesser General Public License as published by the Free Software 00005 Foundation; either version 2.1 of the License, or (at your option) any later 00006 version.*/ 00007 #if !defined(_qrcode_rs_H) 00008 # define _qrcode_rs_H (1) 00009 00010 /*This is one of 16 irreducible primitive polynomials of degree 8: 00011 x**8+x**4+x**3+x**2+1. 00012 Under such a polynomial, x (i.e., 0x02) is a generator of GF(2**8). 00013 The high order 1 bit is implicit. 00014 From~\cite{MD88}, Ch. 5, p. 275 by Patel. 00015 @BOOK{MD88, 00016 author="C. Dennis Mee and Eric D. Daniel", 00017 title="Video, Audio, and Instrumentation Recording", 00018 series="Magnetic Recording", 00019 volume=3, 00020 publisher="McGraw-Hill Education", 00021 address="Columbus, OH", 00022 month=Jun, 00023 year=1988 00024 }*/ 00025 #define QR_PPOLY (0x1D) 00026 00027 /*The index to start the generator polynomial from (0...254).*/ 00028 #define QR_M0 (0) 00029 00030 typedef struct rs_gf256 rs_gf256; 00031 00032 struct rs_gf256{ 00033 /*A logarithm table in GF(2**8).*/ 00034 unsigned char log[256]; 00035 /*An exponential table in GF(2**8): exp[i] contains x^i reduced modulo the 00036 irreducible primitive polynomial used to define the field. 00037 The extra 256 entries are used to do arithmetic mod 255, since some extra 00038 table lookups are generally faster than doing the modulus.*/ 00039 unsigned char exp[511]; 00040 }; 00041 00042 /*Initialize discrete logarithm tables for GF(2**8) using a given primitive 00043 irreducible polynomial.*/ 00044 void rs_gf256_init(rs_gf256 *_gf,unsigned _ppoly); 00045 00046 /*Corrects a codeword with _ndata<256 bytes, of which the last _npar are parity 00047 bytes. 00048 Known locations of errors can be passed in the _erasures array. 00049 Twice as many (up to _npar) errors with a known location can be corrected 00050 compared to errors with an unknown location. 00051 Returns the number of errors corrected if successful, or a negative number if 00052 the message could not be corrected because too many errors were detected.*/ 00053 int rs_correct(const rs_gf256 *_gf,int _m0,unsigned char *_data,int _ndata, 00054 int _npar,const unsigned char *_erasures,int _nerasures); 00055 00056 /*Create an _npar-coefficient generator polynomial for a Reed-Solomon code with 00057 _npar<256 parity bytes.*/ 00058 void rs_compute_genpoly(const rs_gf256 *_gf,int _m0, 00059 unsigned char *_genpoly,int _npar); 00060 00061 /*Adds _npar<=_ndata parity bytes to an _ndata-_npar byte message. 00062 _data must contain room for _ndata<256 bytes.*/ 00063 void rs_encode(const rs_gf256 *_gf,unsigned char *_data,int _ndata, 00064 const unsigned char *_genpoly,int _npar); 00065 00066 #endif 00067
Generated on Tue Jul 12 2022 18:54:12 by
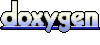