ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
refcnt.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _REFCNT_H_ 00024 #define _REFCNT_H_ 00025 00026 #include <config.h> 00027 #include <assert.h> 00028 00029 #if defined(_WIN32) 00030 # include <windows.h> 00031 00032 typedef volatile LONG refcnt_t; /* FIXME where did volatile come from? */ 00033 00034 static inline int _zbar_refcnt (refcnt_t *cnt, 00035 int delta) 00036 { 00037 int rc = -1; 00038 if(delta > 0) 00039 while(delta--) 00040 rc = InterlockedIncrement(cnt); 00041 else if(delta < 0) 00042 while(delta++) 00043 rc = InterlockedDecrement(cnt); 00044 assert(rc >= 0); 00045 return(rc); 00046 } 00047 00048 00049 #elif defined(HAVE_LIBPTHREAD) 00050 # include <pthread.h> 00051 00052 typedef int refcnt_t; 00053 00054 extern pthread_mutex_t _zbar_reflock; 00055 00056 static inline int _zbar_refcnt (refcnt_t *cnt, 00057 int delta) 00058 { 00059 pthread_mutex_lock(&_zbar_reflock); 00060 int rc = (*cnt += delta); 00061 pthread_mutex_unlock(&_zbar_reflock); 00062 assert(rc >= 0); 00063 return(rc); 00064 } 00065 00066 00067 #else 00068 00069 typedef int refcnt_t; 00070 00071 static inline int _zbar_refcnt (refcnt_t *cnt, 00072 int delta) 00073 { 00074 int rc = (*cnt += delta); 00075 assert(rc >= 0); 00076 return(rc); 00077 } 00078 00079 #endif 00080 00081 00082 void _zbar_refcnt_init(void); 00083 00084 #endif 00085
Generated on Tue Jul 12 2022 18:54:12 by
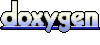