ZBar bar code reader . http://zbar.sourceforge.net/ ZBar is licensed under the GNU LGPL 2.1 to enable development of both open source and commercial projects.
Dependents: GR-PEACH_Camera_in_barcode levkov_ov7670
mutex.h
00001 /*------------------------------------------------------------------------ 00002 * Copyright 2007-2009 (c) Jeff Brown <spadix@users.sourceforge.net> 00003 * 00004 * This file is part of the ZBar Bar Code Reader. 00005 * 00006 * The ZBar Bar Code Reader is free software; you can redistribute it 00007 * and/or modify it under the terms of the GNU Lesser Public License as 00008 * published by the Free Software Foundation; either version 2.1 of 00009 * the License, or (at your option) any later version. 00010 * 00011 * The ZBar Bar Code Reader is distributed in the hope that it will be 00012 * useful, but WITHOUT ANY WARRANTY; without even the implied warranty 00013 * of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser Public License 00017 * along with the ZBar Bar Code Reader; if not, write to the Free 00018 * Software Foundation, Inc., 51 Franklin St, Fifth Floor, 00019 * Boston, MA 02110-1301 USA 00020 * 00021 * http://sourceforge.net/projects/zbar 00022 *------------------------------------------------------------------------*/ 00023 #ifndef _ZBAR_MUTEX_H_ 00024 #define _ZBAR_MUTEX_H_ 00025 00026 #include <config.h> 00027 00028 /* simple platform mutex abstraction 00029 */ 00030 00031 #if defined(_WIN32) 00032 00033 # include <windows.h> 00034 00035 # define DEBUG_LOCKS 00036 # ifdef DEBUG_LOCKS 00037 00038 typedef struct zbar_mutex_s { 00039 int count; 00040 CRITICAL_SECTION mutex; 00041 } zbar_mutex_t; 00042 00043 static inline int _zbar_mutex_init (zbar_mutex_t *lock) 00044 { 00045 lock->count = 1; 00046 InitializeCriticalSection(&lock->mutex); 00047 return(0); 00048 } 00049 00050 static inline void _zbar_mutex_destroy (zbar_mutex_t *lock) 00051 { 00052 DeleteCriticalSection(&lock->mutex); 00053 } 00054 00055 static inline int _zbar_mutex_lock (zbar_mutex_t *lock) 00056 { 00057 EnterCriticalSection(&lock->mutex); 00058 if(lock->count++ < 1) 00059 assert(0); 00060 return(0); 00061 } 00062 00063 static inline int _zbar_mutex_unlock (zbar_mutex_t *lock) 00064 { 00065 if(lock->count-- <= 1) 00066 assert(0); 00067 LeaveCriticalSection(&lock->mutex); 00068 return(0); 00069 } 00070 00071 # else 00072 00073 typedef CRITICAL_SECTION zbar_mutex_t; 00074 00075 static inline int _zbar_mutex_init (zbar_mutex_t *lock) 00076 { 00077 InitializeCriticalSection(lock); 00078 return(0); 00079 } 00080 00081 static inline void _zbar_mutex_destroy (zbar_mutex_t *lock) 00082 { 00083 DeleteCriticalSection(lock); 00084 } 00085 00086 static inline int _zbar_mutex_lock (zbar_mutex_t *lock) 00087 { 00088 EnterCriticalSection(lock); 00089 return(0); 00090 } 00091 00092 static inline int _zbar_mutex_unlock (zbar_mutex_t *lock) 00093 { 00094 LeaveCriticalSection(lock); 00095 return(0); 00096 } 00097 00098 # endif 00099 00100 00101 #elif defined(HAVE_LIBPTHREAD) 00102 00103 # include <pthread.h> 00104 00105 typedef pthread_mutex_t zbar_mutex_t; 00106 00107 static inline int _zbar_mutex_init (zbar_mutex_t *lock) 00108 { 00109 # ifdef DEBUG_LOCKS 00110 pthread_mutexattr_t attr; 00111 pthread_mutexattr_init(&attr); 00112 pthread_mutexattr_settype(&attr, PTHREAD_MUTEX_ERRORCHECK); 00113 int rc = pthread_mutex_init(lock, &attr); 00114 pthread_mutexattr_destroy(&attr); 00115 return(rc); 00116 # else 00117 return(pthread_mutex_init(lock, NULL)); 00118 # endif 00119 } 00120 00121 static inline void _zbar_mutex_destroy (zbar_mutex_t *lock) 00122 { 00123 pthread_mutex_destroy(lock); 00124 } 00125 00126 static inline int _zbar_mutex_lock (zbar_mutex_t *lock) 00127 { 00128 int rc = pthread_mutex_lock(lock); 00129 # ifdef DEBUG_LOCKS 00130 assert(!rc); 00131 # endif 00132 /* FIXME save system code */ 00133 /*rc = err_capture(proc, SEV_ERROR, ZBAR_ERR_LOCKING, __func__, 00134 "unable to lock processor");*/ 00135 return(rc); 00136 } 00137 00138 static inline int _zbar_mutex_unlock (zbar_mutex_t *lock) 00139 { 00140 int rc = pthread_mutex_unlock(lock); 00141 # ifdef DEBUG_LOCKS 00142 assert(!rc); 00143 # endif 00144 /* FIXME save system code */ 00145 return(rc); 00146 } 00147 00148 00149 #else 00150 00151 typedef int zbar_mutex_t[0]; 00152 00153 #define _zbar_mutex_init(l) -1 00154 #define _zbar_mutex_destroy(l) 00155 #define _zbar_mutex_lock(l) 0 00156 #define _zbar_mutex_unlock(l) 0 00157 00158 #endif 00159 00160 #endif 00161
Generated on Tue Jul 12 2022 18:54:12 by
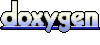