libuav original
Dependents: UAVCAN UAVCAN_Subscriber
storage_marshaller.cpp
00001 /* 00002 * Copyright (C) 2015 Pavel Kirienko <pavel.kirienko@gmail.com> 00003 */ 00004 00005 #include <gtest/gtest.h> 00006 #include <uavcan/protocol/dynamic_node_id_server/storage_marshaller.hpp> 00007 #include "memory_storage_backend.hpp" 00008 00009 TEST(dynamic_node_id_server_StorageMarshaller, Basic) 00010 { 00011 MemoryStorageBackend st; 00012 00013 uavcan::dynamic_node_id_server::StorageMarshaller marshaler(st); 00014 00015 uavcan::dynamic_node_id_server::IStorageBackend::String key; 00016 00017 /* 00018 * uint32 00019 */ 00020 uint32_t u32 = 0; 00021 00022 key = "foo"; 00023 u32 = 0; 00024 ASSERT_LE(0, marshaler.setAndGetBack(key, u32)); 00025 ASSERT_EQ(0, u32); 00026 00027 key = "bar"; 00028 u32 = 0xFFFFFFFF; 00029 ASSERT_LE(0, marshaler.setAndGetBack(key, u32)); 00030 ASSERT_EQ(0xFFFFFFFF, u32); 00031 ASSERT_LE(0, marshaler.get(key, u32)); 00032 ASSERT_EQ(0xFFFFFFFF, u32); 00033 00034 key = "foo"; 00035 ASSERT_LE(0, marshaler.get(key, u32)); 00036 ASSERT_EQ(0, u32); 00037 00038 key = "the_cake_is_a_lie"; 00039 ASSERT_GT(0, marshaler.get(key, u32)); 00040 ASSERT_EQ(0, u32); 00041 00042 /* 00043 * uint8[16] 00044 */ 00045 uavcan::protocol::dynamic_node_id::server::Entry::FieldTypes::unique_id array; 00046 00047 key = "a"; 00048 // Set zero 00049 ASSERT_LE(0, marshaler.setAndGetBack(key, array)); 00050 for (uint8_t i = 0; i < 16; i++) 00051 { 00052 ASSERT_EQ(0, array[i]); 00053 } 00054 00055 // Make sure this will not be interpreted as uint32 00056 ASSERT_GT(0, marshaler.get(key, u32)); 00057 ASSERT_EQ(0, u32); 00058 00059 // Set pattern 00060 for (uint8_t i = 0; i < 16; i++) 00061 { 00062 array[i] = uint8_t(i + 1); 00063 } 00064 ASSERT_LE(0, marshaler.setAndGetBack(key, array)); 00065 for (uint8_t i = 0; i < 16; i++) 00066 { 00067 ASSERT_EQ(i + 1, array[i]); 00068 } 00069 00070 // Set another pattern 00071 for (uint8_t i = 0; i < 16; i++) 00072 { 00073 array[i] = uint8_t(i | (i << 4)); 00074 } 00075 ASSERT_LE(0, marshaler.setAndGetBack(key, array)); 00076 for (uint8_t i = 0; i < 16; i++) 00077 { 00078 ASSERT_EQ(uint8_t(i | (i << 4)), array[i]); 00079 } 00080 00081 // Make sure uint32 cannot be interpreted as an array 00082 key = "foo"; 00083 ASSERT_GT(0, marshaler.get(key, array)); 00084 00085 // Nonexistent key 00086 key = "the_cake_is_a_lie"; 00087 ASSERT_GT(0, marshaler.get(key, array)); 00088 }
Generated on Tue Jul 12 2022 17:17:34 by
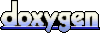