libuav original
Dependents: UAVCAN UAVCAN_Subscriber
Array< T, ArrayMode, MaxSize_ > Class Template Reference
Generic array implementation. More...
#include <array.hpp>
Inherits ArrayImpl< T, ArrayMode, MaxSize_ >.
Public Types | |
typedef T | RawValueType |
This may be not the same as the element type. | |
typedef StorageType< T >::Type | ValueType |
This is the actual stored element type. | |
typedef Base::SizeType | SizeType |
Minimal width size type. | |
typedef ValueType | value_type |
Aliases for compatibility with standard containers. | |
Public Member Functions | |
Array () | |
Default constructor zero-initializes the storage even if it consists of primitive types. | |
Array (const char *str) | |
String constructor - only for string-like arrays. | |
void | pop_back () |
Only for dynamic arrays. | |
void | resize (SizeType new_size, const ValueType &filler) |
Only for dynamic arrays. | |
void | resize (SizeType new_size) |
Only for dynamic arrays. | |
bool | operator== (const char *chr) const |
This operator can only be used with string-like arrays; otherwise it will fail to compile. | |
template<typename R > | |
bool | operator!= (const R &rhs) const |
operator==() | |
SelfType & | operator= (const char *chr) |
This operator can only be used with string-like arrays; otherwise it will fail to compile. | |
SelfType & | operator+= (const char *chr) |
This operator can only be used with string-like arrays; otherwise it will fail to compile. | |
template<uavcan::ArrayMode RhsArrayMode, unsigned RhsMaxSize> | |
SelfType & | operator+= (const Array< T, RhsArrayMode, RhsMaxSize > &rhs) |
Appends another Array<> with the same element type. | |
template<typename A > | |
void | appendFormatted (const char *const format, const A value) |
Formatting appender. | |
void | convertToUpperCaseASCII () |
Converts the string to upper/lower case in place, assuming that encoding is ASCII. | |
template<typename ScalarType > | |
void | packSquareMatrix (const ScalarType(&src_row_major)[MaxSize]) |
Fills this array as a packed square matrix from a static array. | |
void | packSquareMatrix () |
Fills this array as a packed square matrix in place. | |
template<typename ScalarType > | |
void | unpackSquareMatrix (ScalarType(&dst_row_major)[MaxSize]) const |
Reconstructs full matrix, result will be saved into a static array. | |
void | unpackSquareMatrix () |
Reconstructs full matrix in place. | |
Data Fields | |
EnableIf< sizeof((reinterpret_cast < const R * >0))- | size )())&&sizeof((*(reinterpret_cast< const R * >(0)))[0]) |
This operator accepts any container with size() and []. | |
EnableIf< sizeof((reinterpret_cast < const R * >0))- | begin )())&&sizeof((reinterpret_cast< const R * >(0))->size())> |
Fills this array as a packed square matrix from any container that has the following public entities:
|
Detailed Description
template<typename T, ArrayMode ArrayMode, unsigned MaxSize_>
class uavcan::Array< T, ArrayMode, MaxSize_ >
Generic array implementation.
This class is compatible with most standard library functions operating on containers (e.g. std::sort(), std::lexicographical_compare(), etc.). No dynamic memory is used. All functions that can modify the array or access elements are range checking. If the range error occurs:
- if exceptions are enabled, std::out_of_range will be thrown;
- if UAVCAN_ASSERT() is enabled, program will be terminated on UAVCAN_ASSERT(0);
- otherwise the index value will be constrained to the closest valid value.
Definition at line 424 of file array.hpp.
Member Typedef Documentation
typedef T RawValueType |
typedef Base::SizeType SizeType |
Minimal width size type.
Reimplemented from ArrayImpl< T, ArrayMode, MaxSize_ >.
typedef ValueType value_type |
typedef StorageType<T>::Type ValueType |
Constructor & Destructor Documentation
Array | ( | ) |
Array | ( | const char * | str ) |
Member Function Documentation
void appendFormatted | ( | const char *const | format, |
const A | value | ||
) |
Formatting appender.
This method doesn't raise an overflow error; instead it silently truncates the data to fit the array capacity. Works only with string-like arrays, otherwise fails to compile.
- Parameters:
-
format Format string for std::snprintf(), e.g. "%08x", "%f" value Arbitrary value of a primitive type (should fail to compile if there's a non-primitive type)
void convertToUpperCaseASCII | ( | ) |
SelfType& operator+= | ( | const char * | chr ) |
SelfType& operator= | ( | const char * | chr ) |
bool operator== | ( | const char * | chr ) | const |
void packSquareMatrix | ( | const ScalarType(&) | src_row_major[MaxSize] ) |
void packSquareMatrix | ( | ) |
void pop_back | ( | ) |
void resize | ( | SizeType | new_size ) |
void unpackSquareMatrix | ( | ScalarType(&) | dst_row_major[MaxSize] ) | const |
void unpackSquareMatrix | ( | ) |
Field Documentation
EnableIf<sizeof((reinterpret_cast<const R*>0))- begin)())&&sizeof((reinterpret_cast< const R * >(0))->size())> |
Fills this array as a packed square matrix from any container that has the following public entities:
- method begin()
- method size()
- only for C++03: type value_type Please refer to the specification to learn more about matrix packing.
Reconstructs full matrix, result will be saved into container that has the following public entities:
- method begin()
- method size()
- only for C++03: type value_type Please refer to the specification to learn more about matrix packing.
Note that matrix packing code uses areClose() for comparison.
EnableIf<sizeof((reinterpret_cast<const R*>0))- size)())&&sizeof((*(reinterpret_cast< const R * >(0)))[0]) |
This operator accepts any container with size() and [].
This method compares two arrays using areClose(), which ensures proper comparison of floating point values, or DSDL data structures which contain floating point fields at any depth.
Members must be comparable via operator ==.
Please refer to the documentation of areClose() to learn more about how it works and how to define custom fuzzy comparison behavior. Any container with size() and [] is acceptable.
Generated on Tue Jul 12 2022 17:17:37 by
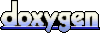